Search of Strings 1. Write a version of the selection sort algorithm presented in the unit, which is used to search a list of strings. 2. Write a version of the binary search algorithm presented in the unit, which is used to search a list of strings. (Use the selection sort that you designed above to sort the list of strings.) 3. Create a test program that primes the list with a set of strings, sorts the list, and then prompts the user to enter a search string. Your program should then search the list using your binary search algorithm to determine if the string is in the list. Allow the user to continue to search for strings until they choose to exit the program
Binary Search of Strings
1. Write a version of the selection sort
to search a list of strings.
2. Write a version of the binary search algorithm presented in the unit, which is
used to search a list of strings. (Use the selection sort that you designed above
to sort the list of strings.)
3. Create a test
then prompts the user to enter a search string. Your program should then search
the list using your binary search algorithm to determine if the string is in the list.
Allow the user to continue to search for strings until they choose to exit the
program

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

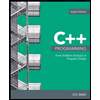
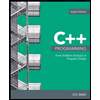