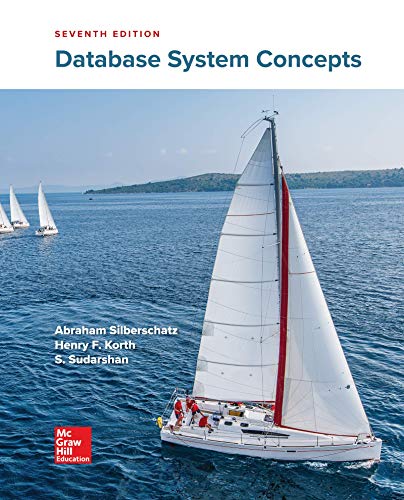
Concept explainers
Search the Tree
A binary search tree is a data structure that consists of JavaScript objects called "nodes". A tree always has a root node which holds its own integer valueproperty and can have up to two child nodes (or leaf nodes), a left and right attribute. A leaf node holds a value attribute and, likewise, a left and rightattribute each potentially pointing to another node in the binary tree. Think of it as a Javascript object with potentially more sub-objects referenced by the left and right attributes (as seen in assignment 4). There are certain rules that apply to a binary tree:
- A node's left leaf node has a value that is <= than to its own value
- A node's right leaf node has a value that is => its own value
In other words:
let node = {
value: <some number>
left: <a node object with value attribute <= this object's value>
right: <a node object with value attribute >= this object's value>
}
If you need a visual aid, below is an example of what a binary tree looks like according to the above rules: -- image attached.
You will be writing a function called isPresent that takes two arguments: the root object and a value (number), and returns a boolean: true if the value is present in the tree or false if it is not present.
function isPresent(root, value) {
// your code here
// return boolean
}
Let's translate the above image into a familiar JavaScript object below as an example:
let tree = {
"value": 100,
"left": {
"value": 50,
"left": {
"value": 25,
"left": null,
"right": null
},
"right": {
"value": 75,
"left": null,
"right": null
}
},
"right": {
"value": 150,
"left": null
"right": null
}
}
console.log(isPresent(tree, 25))
Output: true
Reasoning: 25 is the value of a leaf node in the binary tree
..
"left": {
"value": 25,
"left": null,
"right": null
},
..
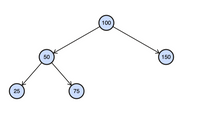

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- JAVA Create binary search tree shown as below. Now delete the key 18, and then calculate the balance factor and convert into AVLtree by proper rotationarrow_forwardJava / Trees: *Please refer to attached image* What is the inorder of this tree? Multiple chocie. G X C A N V F Q L W G X C A N V F L W E A C V N X G F L W E A C V N VG F L W Earrow_forward1. Goals and Overview The goal of this assignment is to practice creating a hash table. This assignment also has an alternative, described in Section 5. A hash table stores values into a table (meaning an array) at a position determined by a hash function. 2. Hash Function and Values Every Java object has a method int hashCode() which returns an int suitable for use in a hash table. Most classes use the hashCode method they inherit from object, but classes such as string define their own hashCode method, overriding the one in object. For this assignments, your hash table stores values of type public class HashKeyValue { K key; V value; } 3. Hash Table using Chained Hashing Create a hash table class (called HashTable211.java) satisfying the following interface: public interface HashInterface { void add(K key, V value); // always succeeds as long as there is enough memory V find (K key); // returns null if there is no such key } Internally, your hash table is defined as a private…arrow_forward
- Don't give me AI generated answer or plagiarised answer. If I see these things I'll give you multiple downvotes and will report immediately.arrow_forwardCreate a function that uses Node * pointing to root of AVL Tree as an input and returns the height of the AVL tree in O(log(n)).arrow_forwardIn Java I'm trying to make a Red Black Tree through inheritance with the Binary Tree I created (as provided along with the Binary Node) yet I'm having a lot of difficulty knowing where and how to start as well as how to override and even create the Insert and Remove functions for the Red Black Tree. Code and assistance in this topic will be greatly appreciated... here is the code I'm utilizing for this project. Binary Node I created: public class BinNode{private String data;private BinNode left;private BinNode right;private int height; //height fieldpublic BinNode(){data = "";left = null;right = null;}public int getHeight() {return height;}public void setHeight(int height) {this.height = height;}public BinNode(String d){data = d;left = null;right = null;}public void setData(String d){this.data = d;}public String getData(){return this.data;}public void setLeft(BinNode l){this.left = l;}public BinNode getLeft(){return this.left;}public void setRight(BinNode r){this.right = r;}public…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
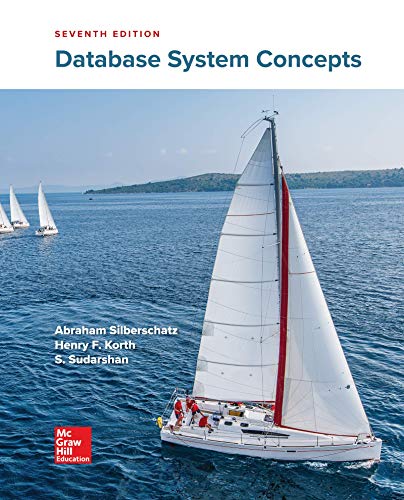
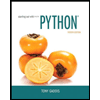
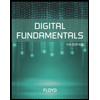
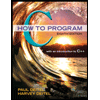
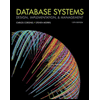
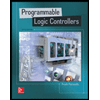