• Shape (this will be the base class) o The _init_ method should take in just the identifier and store it. No dimensions are required. o Both the get_area and get_perimeter methods should display (print) messages saying that the area or perimeter cannot be calculated and then return None. o The get_id method should just return the identifier. Because every other class will be derived from Shape as a child or grandchild, this method will be available to them as well without reimplementation. Ellipse (derived from the Shape class) o The _init__ method should take in an identifier, the major axis radius, and the minor axis radius, call the parent class's (hint: super()) constructor to store the identifier, then store the dimensions (major and minor axis radii) as data variables. o There is no good way to get a perimeter of an ellipse, so the get_perimeter method should display (print) a message saying that the perimeter cannot be calculated. o The get_area method is implementable. Look online for resources. Circle (derived from Ellipse class) o The _init_ method should take in an identifier and radius, then call the parent class's constructor to store the identifier and to store the input argument radius as both the major and minor axis radii of the parent class Ellipse. o The get_area method does not need to be implemented in this classsince the parent class Ellipse already has an implementation. o You will need to implement the get_perimeter method, since there does exist a simple formula to find the perimeter, or circumference, of a circle. • Rectangle (derived from the Shape class) o The _init_ method should take in an identifier, the width, and the length, call the parent class's (hint: super() constructor to store the identifier, then store the dimensions (the width and length) as data variables. o You will need to implement both get_area and get_perimeter. Square (derived from the Rectangle class) • The _init_ method should take an identifier and the side length, calle the parent class's constructor to store the identifier and to store the input argument length as both the width and length of the parent class Rectangle constructor. o You should not need to implement either get_area or get_perimeter in this class, since the parent class Rectangle should cover these methods
• Shape (this will be the base class) o The _init_ method should take in just the identifier and store it. No dimensions are required. o Both the get_area and get_perimeter methods should display (print) messages saying that the area or perimeter cannot be calculated and then return None. o The get_id method should just return the identifier. Because every other class will be derived from Shape as a child or grandchild, this method will be available to them as well without reimplementation. Ellipse (derived from the Shape class) o The _init__ method should take in an identifier, the major axis radius, and the minor axis radius, call the parent class's (hint: super()) constructor to store the identifier, then store the dimensions (major and minor axis radii) as data variables. o There is no good way to get a perimeter of an ellipse, so the get_perimeter method should display (print) a message saying that the perimeter cannot be calculated. o The get_area method is implementable. Look online for resources. Circle (derived from Ellipse class) o The _init_ method should take in an identifier and radius, then call the parent class's constructor to store the identifier and to store the input argument radius as both the major and minor axis radii of the parent class Ellipse. o The get_area method does not need to be implemented in this classsince the parent class Ellipse already has an implementation. o You will need to implement the get_perimeter method, since there does exist a simple formula to find the perimeter, or circumference, of a circle. • Rectangle (derived from the Shape class) o The _init_ method should take in an identifier, the width, and the length, call the parent class's (hint: super() constructor to store the identifier, then store the dimensions (the width and length) as data variables. o You will need to implement both get_area and get_perimeter. Square (derived from the Rectangle class) • The _init_ method should take an identifier and the side length, calle the parent class's constructor to store the identifier and to store the input argument length as both the width and length of the parent class Rectangle constructor. o You should not need to implement either get_area or get_perimeter in this class, since the parent class Rectangle should cover these methods
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 1GZ
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
Code should be in Python
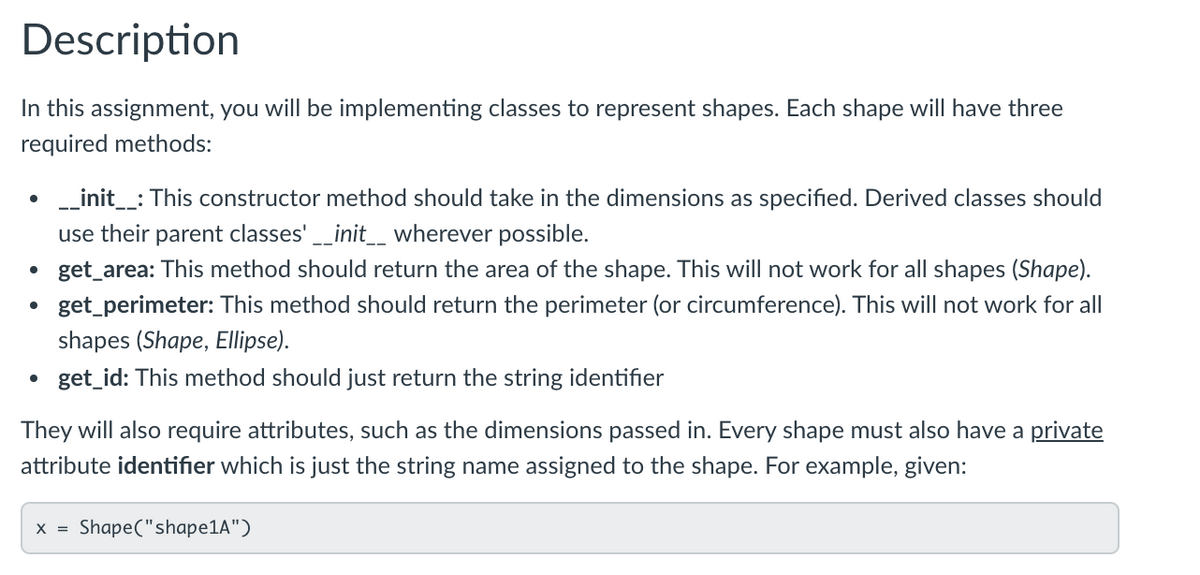
Transcribed Image Text:Description
In this assignment, you will be implementing classes to represent shapes. Each shape will have three
required methods:
• _init_: This constructor method should take in the dimensions as specified. Derived classes should
use their parent classes' _init__ wherever possible.
get_area: This method should return the area of the shape. This will not work for all shapes (Shape).
get_perimeter: This method should return the perimeter (or circumference). This will not work for all
shapes (Shape, Ellipse).
get_id: This method should just return the string identifier
They will also require attributes, such as the dimensions passed in. Every shape must also have a private
attribute identifier which is just the string name assigned to the shape. For example, given:
X =
Shape("shape1A")
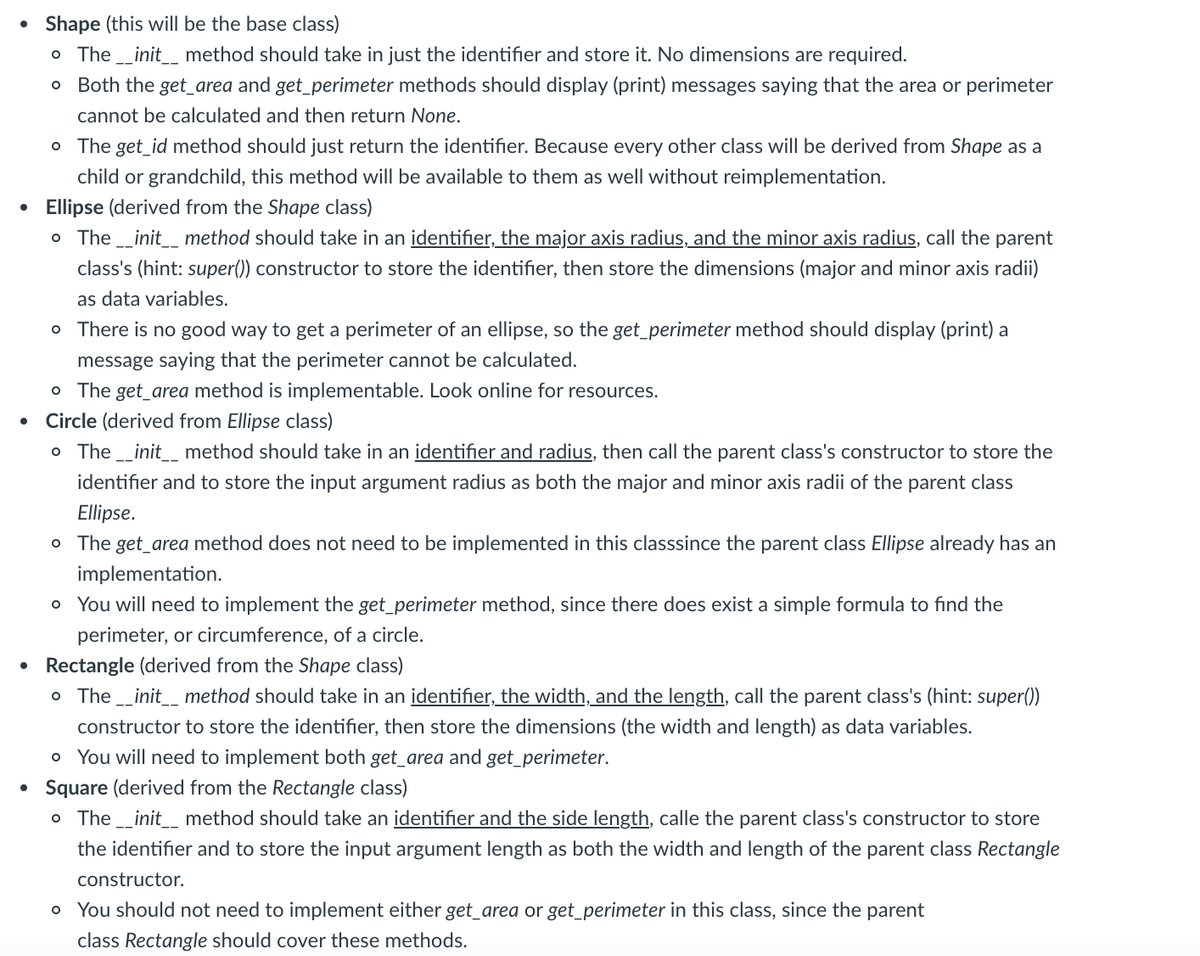
Transcribed Image Text:• Shape (this will be the base class)
The _init_ method should take in just the identifier and store it. No dimensions are required.
o Both the get_area and get_perimeter methods should display (print) messages saying that the area or perimeter
cannot be calculated and then return None.
o The get_id method should just return the identifier. Because every other class will be derived from Shape as a
child or grandchild, this method will be available to them as well without reimplementation.
Ellipse (derived from the Shape class)
o The _init_ method should take in an identifier, the major axis radius, and the minor axis radius, call the parent
class's (hint: super()) constructor to store the identifier, then store the dimensions (major and minor axis radii)
as data variables.
o There is no good way to get a perimeter of an ellipse, so the get_perimeter method should display (print) a
message saying that the perimeter cannot be calculated.
o The get_area method is implementable. Look online for resources.
Circle (derived from Ellipse class)
o The _init_ method should take in an identifier and radius, then call the parent class's constructor to store the
identifier and to store the input argument radius as both the major and minor axis radii of the parent class
Ellipse.
o The get_area method does not need to be implemented in this classsince the parent class Ellipse already has an
implementation.
o You will need to implement the get_perimeter method, since there does exist a simple formula to find the
perimeter, or circumference, of a circle.
• Rectangle (derived from the Shape class)
o The _init_ method should take in an identifier, the width, and the length, call the parent class's (hint: super()
constructor to store the identifier, then store the dimensions (the width and length) as data variables.
o You will need to implement both get_area and get_perimeter.
Square (derived from the Rectangle class)
o The _init_ method should take an identifier and the side length, calle the parent class's constructor to store
the identifier and to store the input argument length as both the width and length of the parent class Rectangle
constructor.
o You should not need to implement either get_area or get_perimeter in this class, since the parent
class Rectangle should cover these methods.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
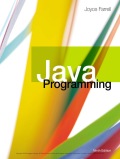
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
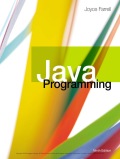
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT