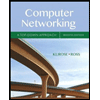
C#. Using the Automobile class you created Chapter 10, Programming Exercise 5A as a base, derive a FinancedAutomobile class that contains all the data of an Automobile, plus the following field:
- AmtFinanced - The amount financed (a double)
Override the parent class ToString() method to include the child class’s additional data.
Create a program named AutomobileDemo2 that contains an array of four FinancedAutomobile objects. Prompt the user for all the necessary data, and do not allow duplicate ID numbers and do not allow the amount financed to be greater than the price of the automobile.
Sort all the records in ID number order and display them with a total price for all FinancedAutomobiles and a total amount financed. For example, the output should be in the following format:
Summary: FinancedAutomobile 1 2017 Honda Price is $26,000.00 Amount financed $12,000.00 FinancedAutomobile 4 2019 Honda Price is $36,000.00 Amount financed $0.00 FinancedAutomobile 5 2016 Toyota Price is $30,000.00 Amount financed $3,000.00 FinancedAutomobile 8 2018 Honda Price is $16,000.00 Amount financed $6,000.00 Total for all Automobiles is $108,000.00 Total financed all Automobiles is $21,000.00
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- PLEASE CODE IN PYTHON PLEASE USE CLASSES The game of Nim starts with a random number of stones between 15 and 30. Two players alternateturns and on each turn may take either 1, 2, or 3 stones from the pile. The player forced to take the laststone loses. Use object-oriented development to create a Nim2 application that allows the user to play Nim against the computer. The Nim2 application and its objects should: • Generate the number of stones to begin with. • Allow the player to go first. • Use a random number generator to determine the number of stones the computertakes. • Prevent the player and the computer from taking an illegal number of stones. Forexample, neither should be allowed to take three stones when there are only 1 or 2left.arrow_forwardI need the java for number 9arrow_forwardCreate a class called Array for performing a float one dimensional array. Define all the necessary variables to represent the private part. Provide a constructor that enables an object of this class to be initialized when it's declared. The constructor should contain default size in case no initializers are provided which is 10. Provide a proper destructor. Provide public methods that perform each of the following tasks: Set the array values (Set). This function sets the array elements with a specific location. Get the array values (Get). This function returns the array element with a specific location. Function called Max to get the maximum element of the array. Write a program to test your class. Define an object (array) with size 20 and another object with the default value. Fill the two arrays with values. Find the maximum number of the first array.arrow_forward
- Which of the statements are true about the class Magazine? 01: class Magazine { 02: public: 03: Magazine (int maxSize = 100); 04: void add (Article a); 05: int numArticles() const; 06: Article getArticle (int i); 07: private: 08: Article* array; 09: int numberOfArticles; 10: }; The compiler will provide a destructor for this class. It has a default constructor It is const-correct. It has a copy constructor The programmer should provide an assignment operatorarrow_forwardC++ Create a class named Student that has three member variables:name – A string that stores the name of the studentnumClasses – An integer that tracks how many courses the student is currently enrolledinclassList – A dynamic array of strings used to store the names of the classes that thestudent is enrolled inWrite appropriate constructor(s), mutator, and accessor methods for the class along with thefollowing: A method that inputs all values from the user, including the list of class names. Thismethod will have to support input for an arbitrary number of classes. A method that outputs the name and list of all courses. A method that resets the number of classes to 0 and the classList to an empty list. An overloaded assignment operator that correctly makes a new copy of the list ofcourses. A destructor that releases all memory that has been allocated.Write a main method that tests all of your functions.arrow_forward2. The MyInteger Class Problem Description: Design a class named MyInteger. The class contains: n [] [] A private int data field named value that stores the int value represented by this object. A constructor that creates a MyInteger object for the specified int value. A get method that returns the int value. Methods isEven () and isOdd () that return true if the value is even or odd respectively. Static methods isEven (int) and isOdd (int) that return true if the specified value is even or odd respectively. Static methods isEven (MyInteger) and isOdd (MyInteger) that return true if the specified value is even or odd respectively. Methods equals (int) and equals (MyInteger) that return true if the value in the object is equal to the specified value. Implement the class. Write a client program that tests all methods in the class.arrow_forward
- Lab 2 – Designing a class This lab requires you to think about the steps that take place in a program by writing pseudocode. Read the following program prior to completing the lab. Design a class named Computer that holds the make, model, and amount of memory of a computer. Include methods to set the values for each data field, and include a method that displays all the values for each field. For the programming problem, create the pseudocode that defines the class and enter it below. Enter pseudocode herearrow_forwardSummary In this lab, you create a derived class from a base class, and then use the derived class in a Python program. The program should create two Motorcycle objects, and then set the Motorcycle’s speed, accelerate the Motorcycle object, and check its sidecar status. Instructions Open the file named Motorcycle.py. Create the Motorcycle class by deriving it from the Vehicle class. Call the parent class __init()__ method inside the Motorcycle class's __init()__ method. In theMotorcycle class, create an attribute named sidecar. Write a public set method to set the value for sidecar. Write a public get method to retrieve the value of sidecar. Write a public accelerate method. This method overrides the accelerate method inherited from the Vehicle class. Change the message in the accelerate method so the following is displayed when the Motorcycle tries to accelerate beyond its maximum speed: "This motorcycle cannot go that fast". Open the file named MyMotorcycleClassProgram.py. In the…arrow_forwardMortgage Calculator In this exercise, you will be completing part of the MortgageCalc class. The MortgageCalc class is a class containing static methods to help calculate elements related to home loans, such as a payment calculator, principal payment calculator, and a remaining principal balance calculator. You will write two methods of the class. public class MortgageCalc { /** * This method calculates the monthly payments for a mortgage payment * @param principal Initial amount of the loan * @param numPayments number of months in the loan * @return the monthly payment for the loan */ public static double payment(double principal, int numPayments) { /* To Be implemented in part A */ } /** * This method returns the remaining balance for a given month * in the loan. * @param originalBal original balance of the loan * @param numPayments original number of months in the loan * @param currPeriod the month to calculate the remaining balance * @return the remaining balance on the…arrow_forward
- Write a program that will contain an array of person class objects. The program should include two classes: 1) One class will be the person class. 2) The second class will be the team class, which contain the main method and the array or array list. The person class should include the following data fields; Name Phone number Birth Date Jersey Number Be sure to include get and set methods for each data field.The team class should contain the data fields for the team, such as: Team name Coach name Conference name The program should include the following functionality. Add person objects to the array; Find a specific person object in the array; (find a person using any data field you choose such as name or jersey number) Output the contents of the array, including all data fields of each person object. (display roster)arrow_forwardHelp me solve this in Java, don't make it to complicated.arrow_forwardWritten in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
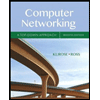
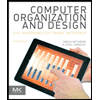
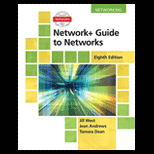
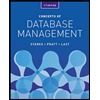
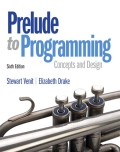
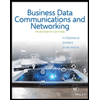