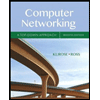
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Here is a command-line inheritance program exercise that has to operate in javaFx.
Most of the structure is there, you just need to add functionality.
This is the prompt:
public class Exhibit {
// Enum Climate arctic, forest, jungle, desert
// make sure all the enum values are public
// by convention the enum values should be capitalized
// ArrayList<Animal> animals
// ArrayList<String> decorations
// Climate environment // a variable to store the climate type in
// add getters and setters
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- In C++ create a program that acts like a bank account. That has 3 classes. The first class must be a generic class that holds basic info deposit, withdraw, calculate monthly interest rate, and a monthly service fee.The second class must be a savings account class that if the savings account falls below $25 it becomes inactive. The final class must be a checking account if someone withdraws more than what they have a service charge of $15 is added and go into a negative balance. The output should display the basic information how much someone deposited or withdrew and their monthly interest rate.arrow_forwardThis is a subclass and doesn't has a main method yet. class Vector{Object items[];int length;int size; void Grow(){// Duplicate sizesize = size * 2; // Allocate new itemsObject new_items[] = new Object[size]; // Copy old itemsfor (int i = 0; i < length; i++)new_items[i] = items[i]; // Discard old itemsitems = new_items; // MessageSystem.out.println("Growing capacity to " + size + " elements");} public Vector(){size = 2;items = new Object[2];} public void Print(){// MessageSystem.out.println("Content:"); // Traversefor (int i = 0; i < length; i++)System.out.println(items[i]);} public void Insert(int index, Object item){// Check indexif (index < 0 || index > length){System.out.println("Invalid index");return;} // Grow if necessaryif (length == size)Grow(); // Shiftfor (int i = length - 1; i >= index; i--)items[i + 1] = items[i]; // Insertitems[index] = item; // One more itemlength++; // MessageSystem.out.println("Inserted " + item);} public void Add(Object…arrow_forwardWrite a program in Java that handles the order list for an online retailer. Your program should contain a minimum of three classes: Order Class Display Class Main Class Create a Order Class that uses a queue to hold the orders in the order they were taken. The queue should contain the customer last name, order number, and order total cost. Create a Display Class that will store a copy of the order queue in two arrays. Each array will contain the order list but one will be sorted by name and the other sorted by order number. When a order is taken and stored in the Order Class the program will update theDisplay Class arrays automatically sort them in descending order using quick sort and outputs the the contents to the console upon each entry of new data. Create a Main Class that will handle operator data input and when an order is added or removed, the program will update the Order Class and Display Class. The user will be presented a menu to add a order, remove a order and display…arrow_forward
- In java ****arrow_forwardJAVA PROGRAM For this program, you are tasked to implement the Beverage class which has the following private properties: name - a string value volume - this is an integer number which represents its current remaining volume in mL isChilled - this is a boolean field which is set to true if the drink is chilled It should have the following methods: isEmpty() - returns true if the volume is already 0 {toString() - returns the details of the object in the following format: {name} ({volume}mL) {"is still chilled" | "is not chilled anymore"}.Example returned strings: Beer (249mL) is still chilled Water (500mL) is not chilled anymore A constructor method with the following signature: public Beverage(name, volume, isChilled) Getter methods for all the 3 properties. Then, create two final subclasses that inherit from this Beverage class. The first one is the Water class which has the additional private property, type, which is a String and can only be either "Purified", "Regular",…arrow_forwardIn C++ create a program that acts like a bank account. It asks the user whether to deposit or withdraw. That has 3 classes. The first class must be a generic class that holds basic info deposit, withdraw, calculate monthly interest rate, and a monthly service fee.The second class must be a savings account class that if the savings account falls below $25 it becomes inactive. The final class must be a checking account if someone withdraws more than what they have a service charge of $15 is added and go into a negative balance. The output should display the basic information how much someone deposited or withdrew and their monthly interest rate.arrow_forward
- T/F 3. Any Java class must have a main method, which is the first method that is called when the Java class is invoked.arrow_forwardData structure & Algrothium java program Create the three following classes: 1. class containing two strings attributes first name and last name.2. class containing the name object and an ArrayList of string to store the list gifts. This class would extend the attached NicePersonInterface.java3. class containing one ArrayList of Names to store the naughty names. Another ArrayList of NicePerson to store the nice names and gifts. Add atleast 4 names in each list and display all information.arrow_forwardIn C++ Create a new project named lab8_1. You will be implementing two classes: A Book class, and a Bookshelf class. The Bookshelf has a Book object (actually 3 of them). You will be able to choose what Book to place in each Bookshelf. Here are their UML diagrams Book class UML Book - author : string- title : string- id : int- count : static int + Book()+ Book(string, string)+ setAuthor(string) : void+ setTitle(string) : void+ print() : void+ setID() : void And the Bookshelf class UML Bookshelf - book1 : Book- book2 : Book- book3 : Book + Bookshelf()+ Bookshelf(Book, Book, Book)+ setBook1(Book) : void+ setBook2(Book) : void+ setBook3(Book) : void+ print() : void Some additional information: The Book class also has two constructors, which again offer the option of declaring a Book object with an author and title, or using the default constructor to set the author and title later, via the setters . The Book class will have a static member variable…arrow_forward
- PLZ help with the following: True/False In java it is possible to throw an exception, catch it, then re-throw that same exception if it is desired GUIs are windowing interfaces that handle user input and output. An interface can contain defined constants as well as method headings or instead of method headings. When a recursive call is encountered, computation is temporarily suspended; all of the information needed to continue the computation is saved and the recursive call is evaluated. Can you have a static method in a nonstatic inner class?arrow_forwardCode: import java.util.*; //Bicycle interface interface Bicycle { abstract void changeCadence(int newValue); //will change value of candence to new value abstract void changeGear (int newValue); //changes gear of car abstract void speedUp(int increment); //increments speed of car by adding new Value to existing speed abstract void applyBrakes(int decrement); } //ACMEBicycle class definition class ACMEBicycle implements Bicycle { int cadence = 0; Â Â int speed = 0; Â Â int gear = 1; //methods of interface public void changeCadence(int newValue) { this.cadence=newValue; } public void changeGear (int newValue) { this.gear=newValue; } public void speedUp(int increment) { this.speed+=increment; } public void applyBrakes(int decrement) { this.speed-=decrement; } //display method void display() { System.out.println("Cadence: "+this.cadence); System.out.println("Gear: "+this.gear); System.out.println("Speed: "+this.speed); } } //KEYOBicycle class definition class KEYOBicycle implements…arrow_forwardCan you please help me with this, its in java. Thank you. Write classes in an inheritance hierarchy Implement a polymorphic method Create an ArrayList object Use ArrayList methods For this, please design and write a Java program to keep track of various menu items. Your program will store, display and modify salads, sandwiches and frozen yogurts. Present the user with the following menu options: Actions: 1) Add a salad2) Add a sandwich3) Add a frozen yogurt4) Display an item5) Display all items6) Add a topping to an item9) QuitIf the user does not enter one of these options, then display:Sorry, <NUMBER> is not a valid option.Where <NUMBER> is the user's input. All menu items have the following: Name (String) Price (double) Topping (StringBuilder or StringBuffer of comma separated values) In addition to everything that a menu item has, a main dish (salad or sandwich) also has: Side (String) A salad also has: Dressing (String) A sandwich also has: Bread type…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
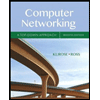
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
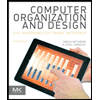
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
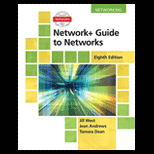
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
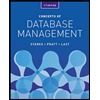
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
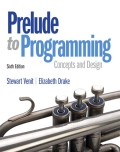
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
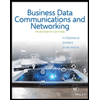
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY