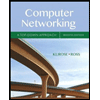
write SQL statements for given questions in order to get familiar to basic
SQL data manipulation and definition statements by
using insert
using update
using alter
create table
create view
2 Problem description
Questions are listed below. See template for required insert, delete and update statements. You will also find
some comments and queries in order to see some possible problems.
1. DEFINE TABLE AUTHORS WITH COLUMNS AUTHORID INT AND AUTHORNAME VARCHAR(30),ADD COLUMN LEVEL PRIMARY KEY CONSTRAINT FOR AUTHORID, ADD COLUMN LEVEL NOT NULL CONSTRAINT FOR AUTHORNAME
2. DEFINE TABLE PUBLISHERS WITH COLUMNS PUBLISHERID INT AND PUBLISHERNAME
VARCHAR(30), ADD TABLE LEVEL PRIMARY KEY CONSTRAINT FOR PUBLISHERID, ADD
TABLE LEVEL UNIQUE CONSTRAINT FOR PUBLISHERNAME
3. ADD NEW COLUMN(CITY VARCHAR(20)) TO PUBLISHERS TABLE
4. SET CITY OF PUBLISHER CAN YAYINLARI TO ˙ISTANBUL
5. DEFINE TABLE NAMED BOOKS WITH COLUMNS BOOKID INT, TITLE VARCHAR(30), AUTHORID ?, PUBLISHERID ?, GENRE VARCHAR(20), ADD FOREIGN KEY CONSTRAINT PUBLISHERID, ADD FOREIGN KEY CONSTRAINT AUTHORID WITH ON DELETE CASCADE
6. ALTER TABLE BOOKS AND ADD NEW PRIMARY KEY CONSTRAINT FOR BOOKID
7. ADD NEW COLUMN (PRIZE NUMERIC(5,2)) FOR BOOKS TABLE
8. ALTER TABLE BOOKS AND ADD NEW CHECK CONSTRAINT FOR PRIZE ≥ 5.00
9. ADD NEW COLUMN (NATION CHAR(2)) FOR AUTHORS TABLE
10. CREATE VIEW BOOKSVIEW WITH AUTHORS, BOOK TITLES AND PRIZE
11. CREATE VIEW PUBVIEW OF PUBLISHERS WITH ID AND NAME
demo.sql
DROP TABLE IF EXISTS EMP;
CREATE TABLE EMP
(EMPNO DECIMAL(4), ENAME VARCHAR(10), JOB VARCHAR(9), MGR DECIMAL(4), HIREDATE DATE, SAL DECIMAL(7, 2), COMM DECIMAL(7, 2), DEPTNO DECIMAL(2));
INSERT INTO emp VALUES ('7369','SMITH','CLERK','7902','1980-12-17','800.00',NULL,'20');INSERT INTO emp VALUES ('7499','ALLEN','SALESMAN','7698','1981-02-20','1600.00','300.00','30');INSERT INTO emp VALUES ('7521','WARD','SALESMAN','7698','1981-02-22','1250.00','500.00','30'); INSERT INTO emp VALUES ('7566','JONES','MANAGER','7839','1981-04-02','2975.00',NULL,'20'); INSERT INTO emp VALUES ('7654','MARTIN','SALESMAN','7698','1981-09-28','1250.00','1400.00','30'); INSERT INTO emp VALUES ('7698','BLAKE','MANAGER','7839','1981-05-01','2850.00',NULL,'30'); INSERT INTO emp VALUES ('7782','CLARK','MANAGER','7839','1981-06-09','2450.00',NULL,'10'); INSERT INTO emp VALUES ('7788','SCOTT','ANALYST','7566','1982-12-09','3000.00',NULL,'20');INSERT INTO emp VALUES ('7839','KING','PRESIDENT',NULL,'1981-11-17','5000.00',NULL,'10'); INSERT INTO emp VALUES ('7844','TURNER','SALESMAN','7698','1981-09-08','1500.00','0.00','30'); INSERT INTO emp VALUES ('7876','ADAMS','CLERK','7788','1983-01-12','1100.00',NULL,'20'); INSERT INTO emp VALUES ('7900','JAMES','CLERK','7698','1981-12-03','950.00',NULL,'30'); INSERT INTO emp VALUES ('7902','FORD','ANALYST','7566','1981-12-03','3000.00',NULL,'20'); INSERT INTO emp VALUES ('7934','MILLER','CLERK','7782','1982-01-23','1300.00',NULL,'10'); DROP TABLE IF EXISTS DEPT; CREATE TABLE DEPT (DEPTNO DECIMAL(2), DNAME VARCHAR(14), LOC VARCHAR(13) ); INSERT INTO DEPT VALUES (10, 'ACCOUNTING', 'NEW YORK'); INSERT INTO DEPT VALUES (20, 'RESEARCH', 'DALLAS'); INSERT INTO DEPT VALUES (30, 'SALES', 'CHICAGO'); INSERT INTO DEPT VALUES (40, 'OPERATIONS', 'BOSTON'); DROP TABLE IF EXISTS BONUS; CREATE TABLE BONUS (ENAME VARCHAR(10), JOB VARCHAR(9), SAL DECIMAL, COMM DECIMAL); DROP TABLE IF EXISTS SALGRADE; CREATE TABLE SALGRADE (GRADE DECIMAL, LOSAL DECIMAL, HISAL DECIMAL); INSERT INTO SALGRADE VALUES (1, 700, 1200); INSERT INTO SALGRADE VALUES (2, 1201, 1400); INSERT INTO SALGRADE VALUES (3, 1401, 2000); INSERT INTO SALGRADE VALUES (4, 2001, 3000); INSERT INTO SALGRADE VALUES (5, 3001, 9999);

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Write Create Table SQL statements based on the above ERD. All tables must have primary keys. All tables must have appropriate foreign key constraints. Foreign keys must have the same name, datatype and size of the primary key that they refer to The following columns datatypes and sizes must be used bid, authid number(4) title, sname, fname varchar2(30) sellingprice, payrate number(6,2)arrow_forwardYou have the following tables: APARTMENTS (ADDRESS, CITY, STATE, RENTER_ID, RENTER_LAST_NAME) RENTERS (RENTER_ID, FIRST_NAME, LAST_NAME) What is the primary key for the APARTMENTS table? (It may be a composite key involving 2 or more fields) What are the foreign keys, if any? What is the primary key for the RENTERS table? What are the foreign keys, if any? What field in RENTERS can be eliminated so that the RENTERS table is normalized?arrow_forwardI. Retrieve the SSN_NUMBER of all employees whose name starts with N. Attribute First Name Last Name SSN Number Data Type Varchar(15) Varchar(15) Char(9) Not Null Not Null Constraint Primary Keyarrow_forward
- Please provide step-by-step in Microsoft Access for the following problem. Create a new query in Query Design View based on the Consultant table with the following instructions to test the new custom function, the Travel function, as used within a query: Use LastName, Reside, and Salary fields from the Consultant Create a new calculated field named TravelExpense that uses the new Travel function with the Reside field for the CountryValue argument and the Salary field for the SalaryValue Save the query with the name TravelCalculation and then display it in Datasheet View to test your new custom function and calculated field. Close the TravelCalculation query.arrow_forwardUsing the Winners table from Figure 12-2, write a parameter query that selects theActor and Actress fields for the year provided by the user.arrow_forwarda column is acting as a unique reference key for a table which kind of key is this? a) compound b) primary c) composite d) foreignarrow_forward
- Write a SELECT statement that returns all rows with salary, last name, street address and ZIP code (SALARY, LAST_NAME, STREET, ZIP_CODE) from the EMPLOYEE and ADDRESS tables, sorted by SALARY from largest to smallest. STREET and ZIP_CODE are in the ADDRESS table, while the other fields are in the EMPLOYEE table. The common key is EMPLOYEE_ID.arrow_forwardPractice Question 6arrow_forwardTask 6: The Car Maintenance team considered that the available maintenance tasks should also have the price information in the database. Alter the MAINTENANCE_TYPES table to include a new column named MAINTENANCE_PRICE of type FLOAT. ANSWER IN MYSQL PLEASEarrow_forward
- Create a table in SQL developer and name it as ‘MyCrush’. The table should consists of only three attributes as follows: Crush_ID which will be the primary key with the number length of .Crush_Name with the maximum character length of 30.Crush_Description with the maximum character length of 10.Insert exactly three records with the above given attributes. Crush_ID values should be 1, 2 and 3 for the three records. Crush_Description should be ‘Present’ for record 1, ‘Past’ for record 2 and ‘Childhood’ for record 3. If you don’t have any crush from your childhood to present, you can insert some random names. Hmm.. Seems Interesting!! It’s time to implement your programming skills using PL/SQL stored procedures and functions. Create another table called ‘MyDetails’ and should contain exactly four attributes as below: Student_ID,Student_Name ,Student_Term ,Student_GPA If you are not a Master’s student, let’s assume you are a student pursuing masters and taking four semesters or terms.…arrow_forward15. Open the AttendeeFirstName query in Design View and add criteria to select only those records where the FirstName field value begins with Lau followed by any other letters. Save the changes to the query. Open the query in Datasheet View, confirm that two records appear in the query results, and then close it.arrow_forwardWrite Create Table SQL statements based on the above ERD. All tables must have primary keys. All tables must have appropriate foreign key constraints. Foreign keys must have the same name, datatype and size of the primary key that they refer to The following columns datatypes and sizes must be used: bid,authid / number(4) title,sname,fname / varchar2(30) sellingprice, payrate / number(6,2) Table Column Type Range author sname & fname Unique book title Not Null book sellingprice Check not negative allocation payrate Check 1 to 79.99arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
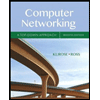
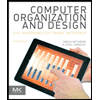
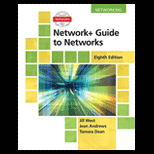
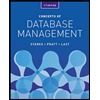
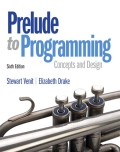
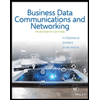