TASK: Add an exception handler (try/catch/throw) to the class that throws an error message (e.g. “value out of range”) in the getValue function that is caught and handled in the main program. Implement two version of the program. In version 1, throw the error in getValue, catch the error in the main, display an error message in the main, and then allow the program to terminate. In the second version, perform the same basic actions (throw and catch) but keep re-invoking the getValue function from the main program until the user enters a valid value. // Starter Code class subRange { public: subRange( int, int ); int getValue( ); private: int lower, upper; }; subRange::subRange( int low, int high ) { lower = low; upper = high; } int subRange::getValue() { int v; cout << "Enter value [ " << lower << ", " << upper << " ]: "; cin >> v; return v; } void main() { subRange x(1,10); cout << x.getValue( ) << endl; }
The following
TASK: Add an exception handler (try/catch/throw) to the class that throws an error message (e.g. “value out of range”) in the getValue function that is caught and handled in the main program. Implement two version of the program. In version 1, throw the error in getValue, catch the error in the main, display an error message in the main, and then allow the program to terminate. In the second version, perform the same basic actions (throw and catch) but keep re-invoking the getValue function from the main program until the user enters a valid value. // Starter Code class subRange { public: subRange( int, int ); int getValue( ); private: int lower, upper; }; subRange::subRange( int low, int high ) { lower = low; upper = high; } int subRange::getValue() { int v; cout << "Enter value [ " << lower << ", " << upper << " ]: "; cin >> v; return v; } void main() { subRange x(1,10); cout << x.getValue( ) << endl; }

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

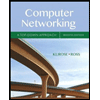
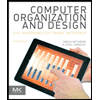
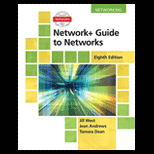
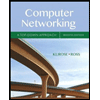
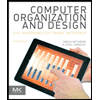
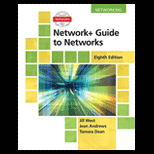
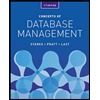
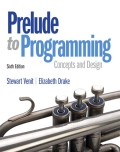
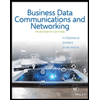