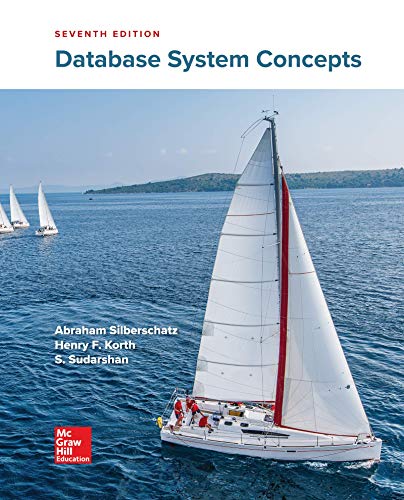
Concept explainers
Task:
Design and implement an assembly language
- Access the RAM address dedicated the keyboard input.
- Continuously check the keyboard input register for the keystroke.
- When a key is pressed, read the character.
- Create a bitmap image and display the character to the simulated screen.
- Upon pressing the Enter key, terminate the program.
Requirements:
- The program should be written in the Hack assembly language.
- The program should use the nand2tetris tools to run on the emulated CPU.
- The program should correctly read input from the keyboard and display it to the simulated screen.
- The program should handle special keys such as Backspace and Enter appropriately.
- The program should be able to handle multiple simultaneous key presses.
Starting code:
// This file is part of www.nand2tetris.org
// and the book "The Elements of Computing Systems"
// by Nisan and Schocken, MIT Press.// Runs an infinite loop that listens to the keyboard input.
// When a key is pressed (any key), the program blackens the screen,
// i.e. writes "black" in every pixel;
// the screen should remain fully black as long as the key is pressed.
// When no key is pressed, the program clears the screen, i.e. writes
// "white" in every pixel;
// the screen should remain fully clear as long as no key is pressed.// Put your code here.
(RESTART)
@SCREEN
D=A
@0
M=D //PUT SCREEN START LOCATION IN RAM0///////////////////////////
(KBDCHECK)@KBD
D=M
@BLACK
D;JGT //JUMP IF ANY KBD KEYS ARE PRESSED
@WHITE
D;JEQ //ELSE JUMP TO WHITEN@KBDCHECK
0;JMP
///////////////////////////
(BLACK)
@1
M=-1 //WHAT TO FILL SCREEN WITH (-1=11111111111111)
@CHANGE
0;JMP(WHITE)
@1
M=0 //WHAT TO FILL SCREEN WITH
@CHANGE
0;JMP
//////////////////////////
(CHANGE)
@1 //CHECK WHAT TO FILL SCREEN WITH
D=M //D CONTAINS BLACK OR WHITE@0
A=M //GET ADRESS OF SCREEN PIXEL TO FILL
M=D //FILL IT@0
D=M+1 //INC TO NEXT PIXEL
@KBD
D=A-D //KBD-SCREEN=A@0
M=M+1 //INC TO NEXT PIXEL
A=M@CHANGE
D;JGT //IF A=0 EXIT AS THE WHOLE SCREEN IS BLACK
/////////////////////////
@RESTART
0;JMP

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- When a procedure written in assembly language is called by a high-level language program, must the calling program and the procedure use the same memory model?arrow_forwardRandom access memory (RAM) is a kind of computer memory that works in a variety of ways. Separate the items in your list into two lists. It's unknown what role it plays in software storage in embedded computers. Explain.arrow_forwardIs it detrimental to a computer's health to use the CPU as little as possible?arrow_forward
- Assembly Language:A memory module is connected to the CPU so that it provides memory words at all addresses from 0x00008000 to 0x00008fff inclusive. How many ARM instructions can the memory hold?arrow_forwardQ1: Write an Assembly language program that allows the user to enter a number and prints the factors of a number. Note: o Assume the number less than 10. o Use a loop instruction. SEA emulator screen (80x25 chars) Enter a number: 8 The factors are: 1 2 4 8 SEH emulator screen (80x25 chars) Enter a number: 5 The factors are: 1 5arrow_forwardA form of memory dynamic relocation requires special hardware registers: A/ and A/ limit registers which one contain the physical address where a program begins and the other the length of the program.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
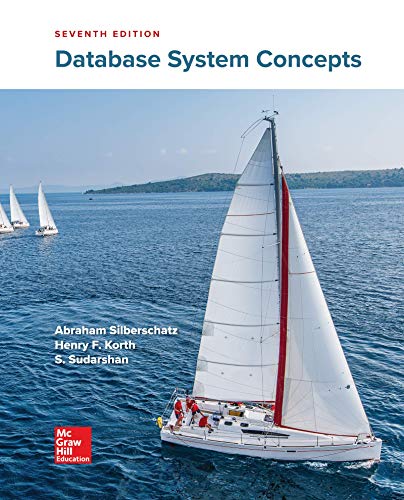
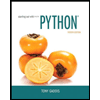
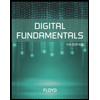
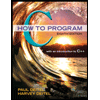
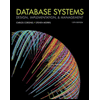
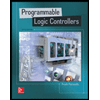