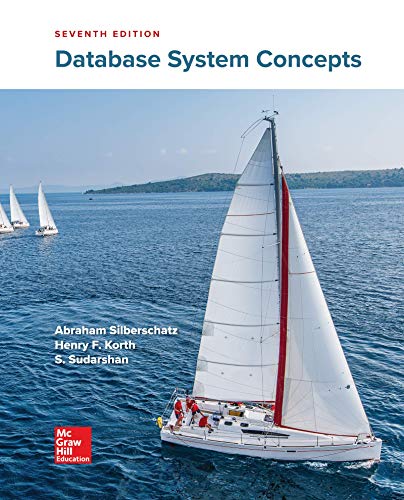
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
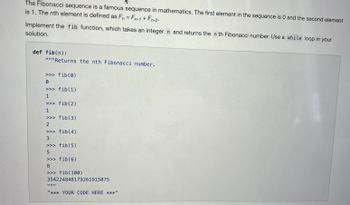
Transcribed Image Text:The Fibonacci sequence is a famous sequence in mathematics. The first element in the sequence is 0 and the second element
is 1. The nth element is defined as Fn = Fn-1 + Fn-2-
Implement the fib function, which takes an integer n and returns the n th Fibonacci number. Use a while loop in your
solution.
def fib(n):
"""Returns the nth Fibonacci number.
>>> fib (0)
0
>>> fib (1)
1
>>> fib(2)
1
>>> fib(3)
2
>>> fib(4)
3
>>> fib(5)
5
>>> fib(6)
8
>>> fib (100)
354224848179261915075
"*** YOUR CODE HERE ***"
Expert Solution

arrow_forward
Step 1
According to the information given:-
We have to follow the instruction mentioned in order to get desired outcome.
Step by stepSolved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- + dgenuity.com/Player/ Semester A def fib(n): Complete the code for this recursive function. if n == 1: return 0 if n == 2: return 1 else: 3 return 5 # The first number in the list. # The second number in the list. # Add the previous two numbers. Mark this and return (n-1) + O (n-2) Σ B 0 Save and Exit Next Sign out English Martarrow_forwardUsing Python: This assignment requires the main function and a custom value-returning function. The value-returning function takes a list of random Fahrenheit temperatures as its only argument and returns a smaller list of only the temperatures above freezing. This value-returning function must use a list comprehension to create the list of above freezing temperatures.In the main function, code these steps in this sequence: create an empty list that will the hold random integer temperatures. use a loop to add 24 random temperatures to the list. All temperatures should be between 10 and 55, inclusive. Duplicates are okay. sort the list in ascending order. use another loop to display all 24 sorted temperatures on one line separated by spaces. 32 could be in the list. If it is, report the index of the first instance of 32. if 32 isn't in the list, add it at index 4. assign a slice consisting of the first six temperatures to a new list. Then print it. execute the custom value-returning…arrow_forwardIn python, The function decodeFromAscii takes a list L of integers in the range 32 through 126. Each item in L is an ASCII code representing a single printable character. The function accumulates the string of characters represented by the list of ASCII codes and returns that string. For example, decodeFromAscii([78, 105, 99, 101, 33]) returns "Nice!" because N is chr(78), i is chr(105), c is chr(99), etc. Note: ASCII was created in the 1960's so programmers could have a standard encoding for common keyboard characters as numbers. It was later expanded to create Unicode so that characters from languages other than English, mathematical symbols, and many other symbols could also be represented by numerical codes. For example: Test Result print(decodeFromAscii([36, 49, 44, 48, 48, 48])) $1,000 print(decodeFromAscii([115, 110, 111, 119])) snowarrow_forward
- Code in Python Write a function, print_perfect_cubes(n), that takes an integer parameter n and prints the perfect cubes starting from 03 = 0 and ending with n3. When n is negative, the function prints nothing; but do not check for this condition with an "if" statement, it is unneccesary; use a for loop that will automatically print nothing when n < 0. Hint for choosing the right range for your for loop: In the general case, when n is not negative, your function will need to print n+1 different values. For example: Test Result print_perfect_cubes(2) 0 1 8 print_perfect_cubes(5) 0 1 8 27 64 125 print_perfect_cubes(-10)arrow_forwardComputer Science please in java. must use while loop.arrow_forwardGiven the following pseudocode: function fun(n) { var outer_count=0; var inner_count=0; var sum = 0; for (var i=0; i 0, as an expression of normal arithmetic and n, what is the value of outer_count that counts the number of times the outer loop executes? Enter an expression Fun.1.inner: Assuming n EN and n > 0, as an expression of normal arithmetic and n, what is the value of inner_count that counts the number of times the inner loop executes? Enter an expressionarrow_forward
- 3-9 Identify Big 0 of each loop.arrow_forwardUsing recursion, write a function sum that takes a single argument n and computes the sum of all integers between 0 and n inclusive. Do not write this function using a while or for loop. Assume n is non-negative. def sum(n): """Using recursion, computes the sum of all integers between 1 and n, inclusive. Assume n is positive. >>> sum(1) 1 >>> sum(5) # 1 + 2 + 3+ 4+ 5 15 "*** YOUR CODE HERE ***"arrow_forwardIn c++ Write a for loop to print all elements in courseGrades, following each element with a space (including the last). Print forwards, th backwards End each loop with a newline. Ex If courseGrades (7, 9, 11, 10), print: 7 9 11 10 10 11 9 7 Hint Use two for loops. Second loop starts with i=NUM VALS-1. (Notes) Note: These activities may test code with different test values. This activity will perform two tests, both with a 4-element array (int courseGrades(4) See 'How to Use zyBooks Also note: If the submitted code tries to access an invalid array element, such as courseGrades[9] for a 4-element array, the test may generate strange results. Or the test may crash and report "Program end never reached", in which case the system doesn't print the test case that caused the reported message 32344345730rity? 2 using namespace std; 3 4 int main() { 5 6 7 8 9 10 11 12 13 14 const int NUM VALS-4; int courseGrades [NUM VALS]; int i; for (i = 0; i > courseGrades[1]; } y Your solution goes here Yarrow_forward
- PrimeAA.java Write a program that will tell a user if their number is prime or not. Your code will need to run in a loop (possibly many loops) so that the user can continue to check numbers. A prime is a number that is only divisible by itself and the number 1. This means your code should loop through each value between 1 and the number entered to see if it’s a divisor. If you only check for a small handful of numbers (such as 2, 3, and 5), you will lose most of the credit for this project. Include a try/catch to catch input mismatches and include a custom exception to catch negative values. If the user enters 0, the program should end. Not only will you tell the user if their number is prime or not, you must also print the divisors to the screen (if they exist) on the same line as shown below AND give a count of how many divisors there are. See examples below. Your program should run the test case exactly as it appears below, and should work on any other case in general. Output…arrow_forwardJava program I need help creating a program that creates a Christmas tree. It has a method that accepts two parameters (one for the number of segments and one for the height of each segment). The left tree has 3 segments with a height of 4 and the right one has two segments with a height of 5. Can you explain how the for loops would work?arrow_forwardCode in R Write a while loop what prints all numbers up to 20, but it skips a collection of numbers: 3,9,13,19. A next statement is useful when we want to skip the current iteration of a loop without terminatingit. On encountering next, the R parser skips further evaluation and starts next iteration of the loop. x <- 1:5for (val in x) {if (val == 3){next}print(val)}arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
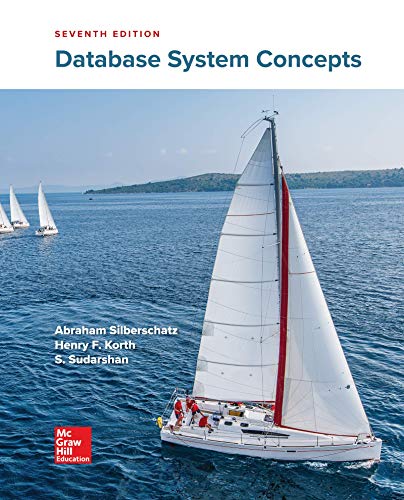
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
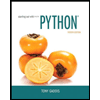
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
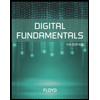
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
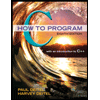
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
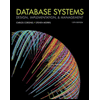
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
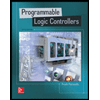
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education