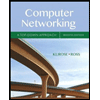
The following is the script for the SQL:
set sql_safe_updates=0;
drop table if exists message;
drop table if exists person;
create table person ( pid integer primary key, pname varchar(20) not null, msgs_sent integer default 0, msgs_rcvd integer default 0 );
create table message ( msg varchar(200) not null, sendtime datetime, senderid integer, receiverid integer, constraint message_pk primary key (sendtime,senderid,receiverid),
constraint message_fk1 foreign key (senderid) references person(pid),
constraint message_fk2 foreign key (receiverid) references person(pid) );
DROP TRIGGER IF EXISTS MESSAGE_SEND;
set sql_safe_updates=0;
DELIMITER $$
CREATE TRIGGER MESSAGE_SEND BEFORE INSERT ON message
FOR EACH ROW
BEGIN
update person set msgs_sent = msgs_sent+1 where pid=new.senderid;
update person set msgs_rcvd = msgs_rcvd+1 where pid=new.receiverid;
END $$
DELIMITER ;
In SQL script create a trigger that fires on deletes to 'message', to keep the sent/received counts correct even when messages are deleted. Test it by
a. Creating some message from BATMAN0 to BATMAN1
b. Doing SELECT * FROM person WHERE pid=1000;
c. Deleting the message you just created.
d. Doing SELECT * FROM person WHERE pid=1000; which should show the mesgs_sent decreasing by 1.

Step by stepSolved in 2 steps with 1 images

- PLZ help with the following using ORACLE command sql Use subqueries CREATE TABLE MEMBERSHIP ( MEM_NUM CHAR(3) CONSTRAINT MEMBER_MEM_NUM_PK PRIMARY KEY, MEM_FNAME VARCHAR(30) NOT NULL, MEM_LNAME VARCHAR(30) NOT NULL, MEM_STREET VARCHAR(15), MEM_CITY VARCHAR(10), MEM_STATE CHAR(2), MEM_ZIP CHAR(5), MEM_BALANCE NUMBER (2)); CREATE TABLE RENTAL ( RENT_NUM CHAR(4) CONSTRAINT RENTAL_RENT_NUM_PK PRIMARY KEY, RENT_DATE DATE, MEM_NUM CHAR(3), CONSTRAINT RENTAL_MEM_NUM_FK FOREIGN KEY (MEM_NUM) REFERENCES MEMBERSHIP); CREATE TABLE PRICE (PRICE_CODE CHAR(1) CONSTRAINT PRICE_PRICE_CODE_PK PRIMARY KEY, PRICE_DESC VARCHAR(20), PRICE_RENTFEE NUMBER (3,1), PRICE_DAILYATFEE NUMBER(3,1)); CREATE TABLE MOVIE (MOVIE_NUM CHAR(4) CONSTRAINT MOVIE_MOVIE_NUM_PK PRIMARY KEY, MOVIE_NAME VARCHAR(30) NOT NULL, MOVIE_YEAR CHAR(4), MOVIE_COST NUMBER(5,2), MOVIE_GENRE VARCHAR(15), PRICE_CODE CHAR(1), CONSTRAINT MOVIE_PRICE_CODE_FK FOREIGN KEY (PRICE_CODE) REFERENCES PRICE); CREATE TABLE VIDEO…arrow_forwardCreate an anonymous block in PL/SQL to list all the countries from Country table where Currency contain keyword ‘Dollar’. Perform following steps: Create user define type with name of the country, currency.Type should contain datatype for name and currency using %type only.Display should include Name of the country, currency code using user defined type. Filter for the data : All the countries from Country table where Currency contain keyword ‘Dollar’.arrow_forwardWrite queries in SQL to answer each of the following questions: 1. Find all students in Comp. Sci. dept 2. Find all students with total credits > 100 3. Find all students who took course in Spring 2010 (Remove duplicates please) 4. Find all courses taken by the student whose ID is 76543 and also find his name create table student (ID varchar(5), name varchar(20) not null, dept_name varchar(20), tot_cred numeric(3,0), primary key (ID) ); create table takes (ID varchar(5), course_id varchar(8), sec_id varchar(8), semester varchar(6), year numeric(4,0), grade varchar(2), primary key (ID, course_id, sec_id, semester, year) ); insert into student values ('00128', 'Zhang', 'Comp. Sci.', '102'); insert into student values ('12345', 'Shankar', 'Comp. Sci.', '32'); insert into student values ('19991', 'Brandt', 'History', '80'); insert into student values ('23121', 'Chavez', 'Finance', '110'); insert into student values ('44553', 'Peltier', 'Physics', '56'); insert into student values…arrow_forward
- use oracle sql developper or Oracle sql developper problem in picture the database CREATE TABLE MEMBERSHIP( MEM_NUM CHAR(3) CONSTRAINT MEMBER_MEM_NUM_PK PRIMARY KEY,MEM_FNAME VARCHAR(30) NOT NULL,MEM_LNAME VARCHAR(30) NOT NULL,MEM_STREET VARCHAR(15),MEM_CITY VARCHAR(10),MEM_STATE CHAR(2),MEM_ZIP CHAR(5),MEM_BALANCE NUMBER (2)); ALTER TABLE MEMBERSHIPMODIFY MEM_STREET VARCHAR(25);CREATE TABLE RENTAL( RENT_NUM CHAR(4) CONSTRAINT RENTAL_RENT_NUM_PK PRIMARY KEY,RENT_DATE DATE,MEM_NUM CHAR(3),CONSTRAINT RENTAL_MEM_NUM_FK FOREIGN KEY (MEM_NUM) REFERENCES MEMBERSHIP); CREATE TABLE PRICE(PRICE_CODE CHAR(1) CONSTRAINT PRICE_PRICE_CODE_PK PRIMARY KEY,PRICE_DESC VARCHAR(20),PRICE_RENTFEE NUMBER (3,1),PRICE_DAILYATFEE NUMBER(3,1)); CREATE TABLE MOVIE(MOVIE_NUM CHAR(4) CONSTRAINT MOVIE_MOVIE_NUM_PK PRIMARY KEY,MOVIE_NAME VARCHAR(30) NOT NULL,MOVIE_YEAR CHAR(4),MOVIE_COST NUMBER(5,2),MOVIE_GENRE VARCHAR(15),PRICE_CODE CHAR(1),CONSTRAINT MOVIE_PRICE_CODE_FK FOREIGN KEY (PRICE_CODE) REFERENCES…arrow_forwardwhich type of joins is not supported by SQL Inner joins Right Joins Full Joins left joinsarrow_forwardORACLE SQL - I have this code and I am getting the error message that there is an error near: WHERE SQR_FT > '1500'; INSERT INTO PROPERTY (office_num, address, sqr_ft, bdrms, floors, monthly_rent, owner_num)vALUES (office_num_val, address_val, sqr_ft_value, bdrms_value, floors_value, monthly_rent_value, owner_num_value)WHERE SQR_FT > '1500';arrow_forward
- HOW TO FIX THIS CODE IN ORACLE SQL ?CREATE OR REPLACE PROCEDURE CONVERT_VAL(V1 VARCHAR,V2 VARCHAR,AMT NUMBER) IS val VARCHAR2(100); val1 VARCHAR2(2); val2 varchar2(2); amount number; DECLARE meter NUMBER := 0; Cem NUMBER := 0; BEGIN val1:=v1; val2:=v2; amount:=amt; Select case when val1!=val2 then case when lower(val1)='km' meter := km * 1000; Cem := meter * 100; dbms_output.Put_line('The value of KM NUMBER KM to meters is: ' ||meter); dbms_output.Put_line('The value of KM NUMBER KM to centimeters is: ' ||cem); when lower(val1)='m' dbms_output.Put_line('The value of M NUMBER M to KM is: ' ||KM); dbms_output.Put_line('The value of M NUMBER M to centimeters is: ' ||cem); when lower(val1)='Cm' dbms_output.Put_line('The value of M NUMBER CM to KM is: ' ||KM); dbms_output.Put_line('The value of M NUMBER CM to M is: ' ||M); else 'same…arrow_forwardFor SQL, Modify the following select statement to make it work. Add one more columns to the second select statement. You can use either a null or a literal value.select number_1, word_1, date_1from sec1508_more_columnsunionselect number_2, word_2from twos;arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
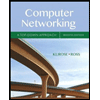
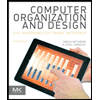
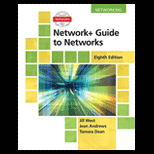
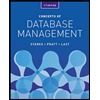
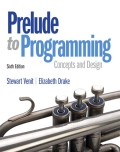
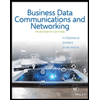