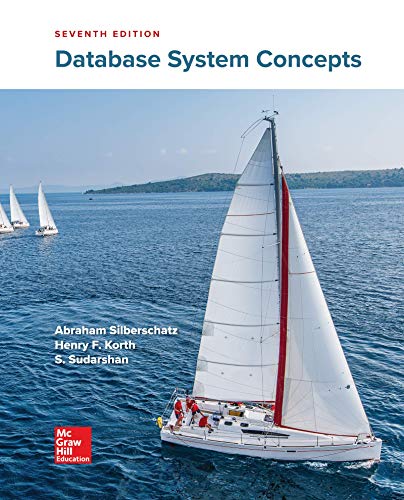
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
The
using System;
using static System.Console;
namespace CheckingForOverflow
{
class Program
{
static void Main(string[] args)
{
try
{
checked
{
int x = int.MaxValue - 1;
WriteLine($"Initial value: {x}");
x++;
WriteLine($"After incrementing: {x}");
x++;
WriteLine($"After incrementing: {x}");
x++;
WriteLine($"After incrementing: {x}");
}
}
catch (OverflowException)
{
WriteLine("The code overflowed but I caught the exception.");
}
unchecked
{
int y = int.MaxValue + 1;
WriteLine($"Initial value: {y}");
y--;
WriteLine($"After decrementing: {y}");
y--;
WriteLine($"After decrementing: {y}");
}
}
}
}
Expert Solution

arrow_forward
Step 1
The program uses keywords checked/unchecked to manage the Overflow program. Comment out try/catch block. Run this code. Update x to var assigned to max decimal. Run it. Update y to object assigned to max ulong. Run it.
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- What is the function of a router in the OSI model, and which layer does it primarily operate in?arrow_forwardSimple try-catch Program This lab is a simple program that demonstrates how try-catch works. You will notice the output when you enter incorrect input (for example, enter a string or double instead of an integer). Type up the code, execute and submit the results ONLY. Do at least 2 valid inputs and 1 invalid. NOTE: The program does not stop executing after it encounters an error! CODE: import java.util.Scanner; public class TryCatch Example Simple { public static void main(String[] args) { Scanner input = new Scanner(System.in); int num = 0; System.out.println("Even number tester.\n"); System.out.println("Enter your name: "); String name = input.nextLine(); while (true) { try { System.out.println("Enter an integer : ");arrow_forwardusing System; class TicTacToe { Console.WriteLine("Do you want be X or O: "); Console.Write("Press 1 for 'X' or Press 2 for 'O'\n"); choice = int.Parse(Console.ReadLine()); if(choice==1) staticint player = 1 staticint choice; { staticvoid Print(char[] board) { Console.WriteLine(); Console.WriteLine($" {board[0]} | {board[1]} | {board[2]} "); Console.WriteLine($" {board[3]} | {board[4]} | {board[5]} "); Console.WriteLine($" {board[6]} | {board[7]} | {board[8]} "); Console.WriteLine(); } staticvoid Main(string[] args) { char[] board = newchar[9]; for (int i = 0; i < 9; i = i + 1) { board[i] = ' '; } Print(board); board[4] = 'X'; Print(board); board[0] = 'O'; Print(board); board[3] = 'X'; Print(board); board[5] = 'O'; Print(board); board[6] = 'X'; Print(board); board[2] = 'O'; Print(board); } } } How can this program be fixed to ask the user "It is your turn to place an X. Where would you like to place it?"arrow_forward
- Integer userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forwardDo in Scala languagearrow_forwardin python create function that has a parameter of dict[str, str] key should be a name value should be a word when given a dict of names and word, function should return the word that is most common ******************************* no using key, value for loops no .get, .items, .defaultset no importing builtins no using .values, .keys, or .index must do it through dictionary cycling ************************** should return a stringarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
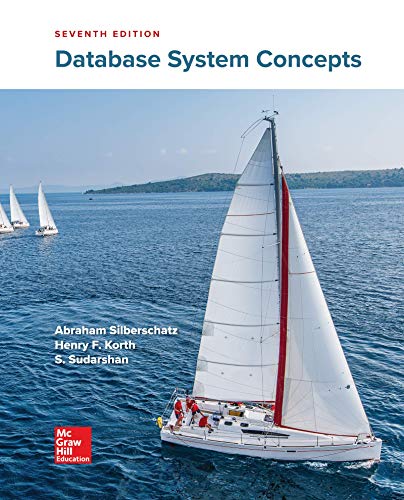
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
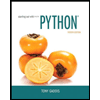
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
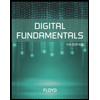
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
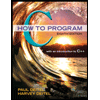
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
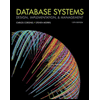
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
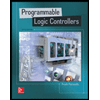
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education