I worked on this assignment and I'm not sure what I need to corect to make it work. Please help me! public class CustomIntegerArrayList { private ArrayList arr; public CustomIntegerArrayList() { this.arr = new ArrayList(); } public ArrayList getArrayList() { return this.arr;} public int get(int index) { return this.arr.get(index); } public void add(int element) { this.arr.add(element); return; } public void add(int index, int element) { this.arr.add(index, element); } public int remove(int index) { return this.arr.remove(index); } public void remove(int num, int element) { int count = 0; while (count < num) { if (this.arr.contains(Integer.valueOf(element))) { this.arr.remove(Integer.valueOf(element)); count+=1; } }; } public ArrayList splice(int index, int num) { int count = 0; while (count < num) { //this.arr.get(index); this.arr.remove(index); count+=1; }; return null; } /** * Removes the specified number (num) of elements from the internal ArrayList of elements, starting at the given index, and inserts the elements in the given otherArray at the given index. * Uses the splice(int index, int num) method. * If index < 0, don't remove anything or insert anything, and return an empty ArrayList. * If index is too large (>= to the size of this CustomIntegerArrayList), don't remove anything or insert anything, and return an empty ArrayList. * if num == 0, don't remove anything or insert anything, and return an empty ArrayList. * If the number of elements after the given index is less than the given num, just remove the rest of the elements in the internal ArrayList. * Example(s): * - For a defined CustomIntegerArrayList containing the integers: 1, 2, 3, 4, 5 * - Calling splice(1, 2, [6, 7]) would remove 2 and 3 (the 2nd and 3rd items), and insert 6 and 7 at index 1. * - The CustomIntegerArrayList will then contain the integers: 1, 6, 7, 4, 5 and this method would return a new ArrayList containing the removed elements: 2 and 3 * - For a defined CustomIntegerArrayList containing the integers: 1, 2, 3, 4, 5 * - In a call to splice(3, 4, [1000, 1001]), the number of elements after the given index 3 is less than the given num 4 * - This would remove 4 and 5 (the 4th and 5th items) and insert 1000 and 1001 at index 3. * - The CustomIntegerArrayList will then contain the integers: 1, 2, 3, 1000, 1001 and this method would return a new ArrayList containing the removed elements: 4 and 5 * - For a defined CustomIntegerArrayList containing the integers: 100, 200, 500 * - Calling splice(1, 0, [700]) would remove nothing and insert nothing and this method would return a new empty ArrayList * - For a defined CustomIntegerArrayList containing the integers: 5, 2, 7, 3, 7, 8 * - Calling splice(6, 3, [9]) would remove nothing and insert nothing and this method would return a new empty ArrayList */ public ArrayList splice(int index, int num, int[] otherArray) { int count = 0; while(count arr2Elements = new ArrayList(); arr2Elements.add(5); arr2Elements.add(15); arr2Elements.add(27); CustomIntegerArrayList arr2 = new CustomIntegerArrayList(arr2Elements); //get internal arraylist of elements System.out.println(arr2.getArrayList()); // [5, 15, 27] } }
I worked on this assignment and I'm not sure what I need to corect to make it work. Please help me!
public class CustomIntegerArrayList {
private ArrayList<Integer> arr;
public CustomIntegerArrayList() {
this.arr = new ArrayList<Integer>(); }
public ArrayList<Integer> getArrayList() {
return this.arr;}
public int get(int index) {
return this.arr.get(index);
}
public void add(int element) {
this.arr.add(element);
return;
}
public void add(int index, int element) {
this.arr.add(index, element);
}
public int remove(int index) {
return this.arr.remove(index);
}
public void remove(int num, int element) {
int count = 0;
while (count < num) {
if (this.arr.contains(Integer.valueOf(element))) {
this.arr.remove(Integer.valueOf(element));
count+=1;
}
};
}
public ArrayList<Integer> splice(int index, int num) {
int count = 0;
while (count < num) {
//this.arr.get(index);
this.arr.remove(index);
count+=1;
};
return null;
}
/**
* Removes the specified number (num) of elements from the internal ArrayList of elements, starting at the given index, and inserts the elements in the given otherArray at the given index.
* Uses the splice(int index, int num) method.
* If index < 0, don't remove anything or insert anything, and return an empty ArrayList.
* If index is too large (>= to the size of this CustomIntegerArrayList), don't remove anything or insert anything, and return an empty ArrayList.
* if num == 0, don't remove anything or insert anything, and return an empty ArrayList.
* If the number of elements after the given index is less than the given num, just remove the rest of the elements in the internal ArrayList.
* Example(s):
* - For a defined CustomIntegerArrayList containing the integers: 1, 2, 3, 4, 5
* - Calling splice(1, 2, [6, 7]) would remove 2 and 3 (the 2nd and 3rd items), and insert 6 and 7 at index 1.
* - The CustomIntegerArrayList will then contain the integers: 1, 6, 7, 4, 5 and this method would return a new ArrayList containing the removed elements: 2 and 3
* - For a defined CustomIntegerArrayList containing the integers: 1, 2, 3, 4, 5
* - In a call to splice(3, 4, [1000, 1001]), the number of elements after the given index 3 is less than the given num 4
* - This would remove 4 and 5 (the 4th and 5th items) and insert 1000 and 1001 at index 3.
* - The CustomIntegerArrayList will then contain the integers: 1, 2, 3, 1000, 1001 and this method would return a new ArrayList containing the removed elements: 4 and 5
* - For a defined CustomIntegerArrayList containing the integers: 100, 200, 500
* - Calling splice(1, 0, [700]) would remove nothing and insert nothing and this method would return a new empty ArrayList
* - For a defined CustomIntegerArrayList containing the integers: 5, 2, 7, 3, 7, 8
* - Calling splice(6, 3, [9]) would remove nothing and insert nothing and this method would return a new empty ArrayList */
public ArrayList<Integer> splice(int index, int num, int[] otherArray) {
int count = 0;
while(count<num){
otherArray[count] = this.arr.get(index);
this.arr.remove(index);
count+=1;
}
System.out.println(otherArray);
return null;
}
public static void main(String args[]) {
//create new empty CustomIntegerArrayList
CustomIntegerArrayList arr1 = new CustomIntegerArrayList();
//add element
arr1.add(2);
//get internal arraylist of elements
System.out.println(arr1.getArrayList()); // [2]
//add element
arr1.add(0, 5);
//get internal arraylist of elements
System.out.println(arr1.getArrayList()); // [5, 2]
//remove element
arr1.remove(2, 2);
//get internal arraylist of elements
System.out.println(arr1.getArrayList()); // [5]
//add more elements
arr1.add(6);
arr1.add(2);
arr1.add(7); // [3, 6, 2, 7]
//splice 2 elements at index 0
arr1.splice(0, 2);
//get internal arraylist of elements
System.out.println(arr1.getArrayList()); // [2, 7]
//splice 1 element at index 0 and add [4, 5]
arr1.splice(0, 1, new int[] { 4, 5 });
//get internal arraylist of elements
System.out.println(arr1.getArrayList()); // [4, 5, 7]
//create new CustomIntegerArrayList with the elements in the given ArrayList
ArrayList<Integer> arr2Elements = new ArrayList<Integer>();
arr2Elements.add(5);
arr2Elements.add(15);
arr2Elements.add(27);
CustomIntegerArrayList arr2 = new CustomIntegerArrayList(arr2Elements);
//get internal arraylist of elements
System.out.println(arr2.getArrayList()); // [5, 15, 27]
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 7 images

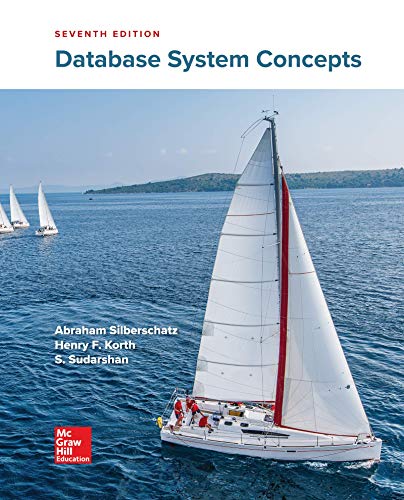
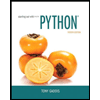
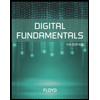
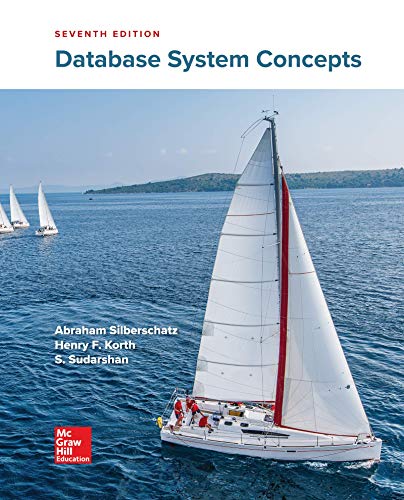
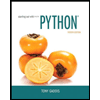
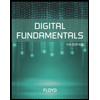
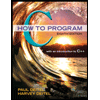
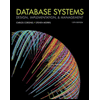
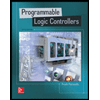