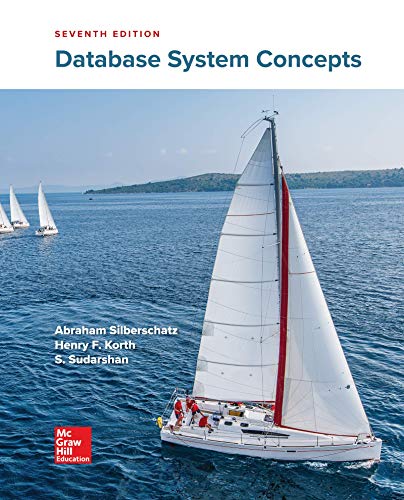
Trying to figure C++
File IO
Control structures
Looping
Operator precedence
Error checking (bad data, filestream fail state, etc.)
Use of proper data types; type coersion and/or explict type conversion
Exact output
Write a program that will calculate numerical and letter grades for a student and output a report:
- The information will be in the provided data file txt. The data file consists of several rows of data containing a student name and information for 3 types of assignments:
First Name, Last Name, number of homework grades, values for homework grades, percentage of total grade, number of program grades, values for program grades, percental of total grade, number of exam grades, values for exam grades, percentage of exam grades. For example, the first row of the data file contains the following:
Sherlock Holmes 3 100 100 100 0.15 3 75 80 90 0.40 3 60 70 70 0.45
The student, Sherlock Holmes, has three homework grades, each with a value of 100. Homework is 15% of total grade. He has three program grades with values of 75, 80, 90. Program is 40% of grade. He has three exam grades of 60, 70, 70. Exams are 45% of grade.
- Request the name of the input file from the user. Do not “hard-code” the file name in the program. Check to ensure the input file opens. If it does not open, display an appropriate message to the user and exit the program.
- Using a looping construct, read until end of file. Do not hard code the number of rows in the loop. The file must be read using an outer loop and three inner/nested loops to read all the data properly. The first number will aid in determining the NUMBER of grades to read. Do NOT assume there will be only 3 values in each category. Code for a variable number of values in each category.
- The maximum points for each assignment, homework and exam are 100. The maximum points for each may be calculated by multiplying the number of assignments in the group by 100. Calculate final grade using the following formula:
((total HOMEWORK Points earned/maximum HOMEWORK points) * percent of total grade) + (total PROGRAM Points earned/maximum PROGRAM points) * percent of total grade) + (total EXAM Points earned/maximum EXAM points) * percent of total grade)) * 100
- Use the final numerical grade to look up corresponding letter grade as follows:
90 - 100 = A
80 - 89 = B
70 - 79 = C
60 - 69 = D
< 60 = F
- Use the final letter grade to look up a "witty" comment of your choice, using a SWITCH statement. A statement must be output for each letter grade above. For example:
A = "Most excellent work”
B = "IMHO You Passed"
C="Got to be quicker than that"
D= "Dog gone try harder"
F= "Try again next time"
- Request an output file name from the user. Do not “hard-code” the file name in the program. Check to ensure the output file opens. If it does not open, display an appropriate message to the user and exit the program.
- For each student, output the following to the console (screen) and the output file in a neat, readable format. Output each input line on one output line. Use manipulators to output values in readable columns:
Student Name, Total Points (numerical grade): NN. Letter Grade: X. Depending on letter grade, The witty comment.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- File Handling Assignment -2 Write a program to generate a file numbers.txt that contains the first 50 odd natural numbers and each line in the file should contain 5 numbers only. Attach the screenshot of numbers.txt. In C or C++arrow_forwardAdd comments for Program 04arrow_forwardC programming language Criteria graded: Declare file pointers Open file Read from file Write to file Close file Instructions: Write a segment of code that opens a file called “numbers.txt” for reading. This file is known to have 10 numbers in it. Read in each number and print a ‘*’ to the screen if the number is even. If the number is odd, do nothing. Conclude by closing this file.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
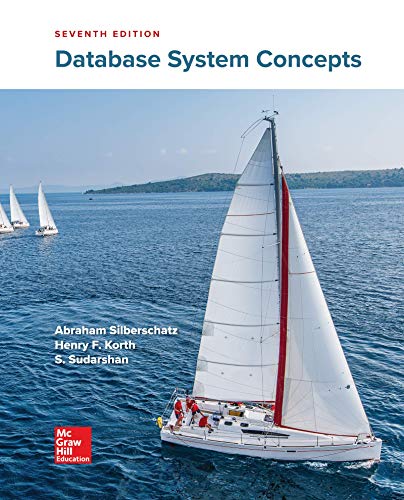
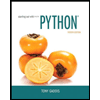
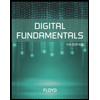
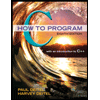
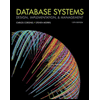
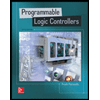