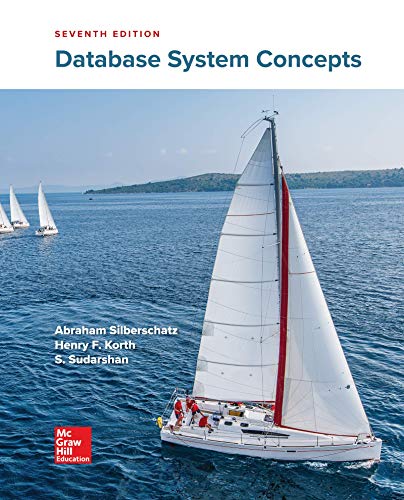
Use C++ code and draw a flow chart
Write a program that simulates a magic square using 3 one dimensional parallel arrays of integer type.
Each one of the arrays corresponds to a row of the magic square.
The program asks the user to enter the values of the magic square row by row and informs the user if the grid is a magic square or not.
Project Specifications
Input for this project:
- Values of the grid (row by row)
Output for this project:
- Whether or not the grid is a magic square
- Programmer’s full name
- Project number
- Project due date
Processing Requirements
Use the following template to start your project:
#include<iostream>
using namespace std;
// Global constants
const int ROWS = 3; // The number of rows in the array
const int COLS = 3; // The number of columns in the array
const int MIN = 1; // The value of the smallest number
const int MAX = 9; // The value of the largest number
// Function prototypes
bool isMagicSquare(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkRange(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max);
bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkRowSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
bool checkColSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
bool checkDiagSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
void fillArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
void showArray(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
int main()
{
/* Define a Lo Shu Magic Square using 3 parallel arrays corresponding to each row of the grid */
int magicArrayRow1[COLS], magicArrayRow2[COLS], magicArrayRow3[COLS];
// Your code goes here
return 0;
}
// Function definitions go here
Create and use the following functions:
- void fillArray(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size) - Accepts 3 int arrays and size as arguments, and fills the arrays out with values entered by the user. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square
- void showArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - accepts 3 int arrays and size as arguments and displays their content.
Example:
1 3 5 (arrayRow1)
6 7 9 (arrayRow2)
8 2 4 (arrayRow3)
- bool isMagicSquare(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - accepts 3 int arrays and a size as arguments and returns true if all the requirements of a magic square are met. Otherwise, it returns false. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square.
- bool checkRange(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max) - accepts 3 int arrays, a size, and a min and max value as arguments and returns true if the values in the arrays are within the specified range min and max. Otherwise, it returns false. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square.
- bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - accepts 3 int arrays and size as arguments, and returns true if the values in the arrays are unique (only one occurrence of numbers between 1-9). Otherwise, it returns false. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square.
- bool checkRowSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - accepts 3 int arrays and size as arguments and returns true if the sum of the values in each of the rows are equal. Otherwise, it returns false. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square.
- bool checkColSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - accepts 3 int arrays and size as arguments and returns true if the sum of the values in each of the columns are equal. Otherwise, it returns false. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square.
- bool checkDiagSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size) - accepts 3 int arrays and size as arguments and returns true if the sum of the values in each of the array's diagonals are equal. Otherwise, it returns false. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 6 images

- Write a C# program that will generate a 1000000 element integer array and fill the array with random numbers between 1 and 10000 (inclusive). Your program should then ask the user to enter an integer and search the array for the integer the user entered and output all the locations (subscripts) at which the array contains the number. (See below for some tips on random numbers).arrow_forwardWrite a C# program that creates an integer array of size 10 and fills the array with numbers set by the programmer (not entered by the user). Then, the program will create two arrays, one of them contains only the odd values of the original array, and the other contains the even values. Note that the size of the newly created arrays should be equal to the number of elements in the array. For example, if the original array contains three odd values, the size of the array that contains the odd values should be equal to three.arrow_forwardJAVA based programarrow_forward
- You can Use C++ programming, Windows Programming, Java, or Ptyhon to solve it The following table contains quarterly sales figures for five (5) departments: Quarter 1 Quarter 2 Quarter 3 Quarter 4 Total Department 1 750 660 910 800 Department 2 800 700 950 900 Department 3 700 600 750 600 Department 4 850 800 1000 950 Department 5 900 800 960 980 Total Design and write a Windows program/module named SalesAnalysis that will: Declare a two-dimensional integer array named sales – (Note: you have 6 rows and 5 columns) - that will hold the 4 quarterly sales for 5 departments Populate the first four columns for the 5 departments using the data in the preceding table. Contain a loop to compute and populate the total column. Store each department’s total in the array as it is being computed. Contain a loop to compute and populate the total row. Store each quarter’s total in the array as it is…arrow_forwardDescription Dot Product Task: Write a program that outputs the dot product of two given vectors. Your program should read the vectors from command line arguments, convert them to integers, output the vectors, and finally compute and output the resulting dot product. Let V and U be two vectors, where |V| we run the program as $ python dotproduct.py 156724 then the two vectors are V = [1, 5, 6] U = [7, 2, 4] and their dot product is calculated by V • U = (1 × 7) + (5 * 2) + (6 × 4) Example $ python dotproduct.py 1 23321 V = { 1, 2, 3 } U = {3, 2, 1 } V. U = 10 = ||U|= = 3 (the size of both vectors will always be 3). Ifarrow_forwardA vector in Matlab has magnitude and direction is an array with only one column or one row is a table of numbers contains at most three numbers, one for each dimension. When adding two arrays in Matlab the arrays only need to have the same number of elements the arrays must have the exact same size and shape you need to put a period in front of the plus sign both b and c are true When combining a scalar c to an array A with a command like c + A Matlab adds the c to every element in A an error is displayed because the dimensions are different the result will be different than the command A + carrow_forward
- program must include a processing loop that allows multiple sets of data to be processed and the program should be terminated when there is no more data to process Develop a program in python that allows the user to enter a start value of 1 to 4, a stop value of 5 to 12 and a multiplier of 2 to 8. The program must display a multiplication table with results using these values. For example, if the user enters a start value of 3, a stop value of 7 and a multiplier value of 3, the table should be displayed as follows: Multiplication Table 3 x 3 = 9 3 x 4 = 12 3 x 5 = 15 3 x 6 = 18 3 x 7 = 21 Run the program with the following values: Start Stop Multiplier 2 10 4 4 12 6arrow_forwardYou can use any C# compiler or the method you already know. The following table contains quarterly sales figures for five (5) departments: Quarter 1 Quarter 2 Quarter 3 Quarter 4 Total Department 1 750 660 910 800 Department 2 800 700 950 900 Department 3 700 600 750 600 Department 4 850 800 1000 950 Department 5 900 800 960 980 Total Design and write a Windows program/module named SalesAnalysis that will: Declare a two-dimensional integer array named sales – (Note: you have 6 rows and 5 columns) - that will hold the 4 quarterly sales for 5 departments Populate the first four columns for the 5 departments using the data in the preceding table. Contain a loop to compute and populate the total column. Store each department’s total in the array as it is being computed. Contain a loop to compute and populate the total row. Store each quarter’s total in the array as it is being computed.…arrow_forwardin c++ using 2D Arrays The following diagram represents an island with dry land (represented by “-“) surrounded by water ((represented by “#“). ##-########## #-----------# #-----------# #------------ # -----------# #------X----# #-----------# ############# Two bridges lead off the island. A mouse (represented by “X”) is placed on the indicated square. Write a program to make the mouse take a walk across the island. The mouse is allowed to travel one square at a time, either horizontally or vertically. A random number from 1 to 4 should be used to decide which direction the mouse is to take; for the sake of uniformity assume that 1 = up, 2 = down, 3 = left, and 4 = right. Since the mouse is wearing cement mouse galoshes, the mouse drowns when he hits the water. He escapes when he steps on a bridge. You may generate a random number up to 100 times allowing the mouse to take 100 steps. If the mouse does not find a bridge by the 100th try, he will wither away and die of starvation.…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
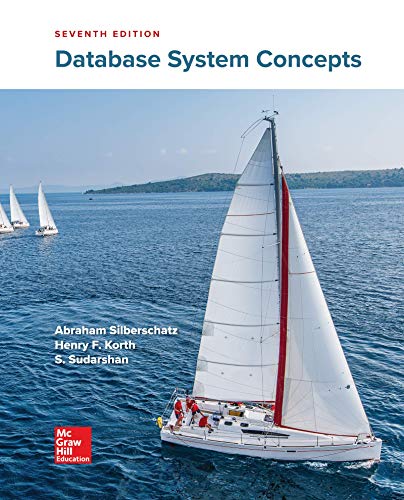
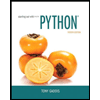
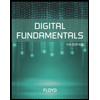
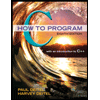
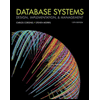
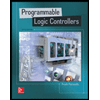