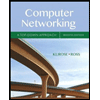
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
USE C++!!
Given a string you need to print all possible strings that can be made by placing spaces (zero or one) in between them. The output should be printed in sorted increasing order of strings.
You don't need to read input or print anything. Your task is to complete the function
Expected Time Complexity: O(2^n)
Expected Auxiliary Space: O(1)
CONSTRAINTS:
1 < |S| < 10
S only contains lowercase and Uppercase English letters.
ATTACHED BELOW ARE THE REQUIRED DRIVER CODES
![```cpp
// { Driver Code Starts
#include <bits/stdc++.h>
using namespace std;
// } Driver Code Ends
class Solution{
public:
vector<string> permutation(string S){
// Code Here
}
};
// { Driver Code Starts.
int main(){
int t;
cin >> t;
while(t--){
string S;
cin >> S;
vector<string> ans;
Solution obj;
ans = obj.permutation(S);
for(int i=0; i < ans.size(); i++){
cout << "(" << ans[i] << ")";
}
cout << endl;
}
}
// } Driver Code Ends
```
### Explanation
This C++ code is designed to calculate permutations of a given string. Here's how the code is structured:
1. **Includes and Namespace:**
- `#include <bits/stdc++.h>`: Includes all standard C++ libraries.
- `using namespace std;`: Allows usage of standard library components without prefixing them with `std::`.
2. **Class Definition:**
- The class `Solution` contains a public member function `permutation`, which takes a string `S` as input and returns a `vector<string>`. The actual implementation of the permutation logic inside the function is missing (indicated by `// Code Here`).
3. **Main Function:**
- Reads an integer `t` which denotes the number of test cases.
- For each test case, it:
- Reads a string `S`.
- Creates an instance of `Solution`.
- Calls the `permutation` method to compute permutations of `S`.
- Prints each permutation in the format `(permutation)`, separated by space.
- Outputs a newline after printing all permutations for a test case.
### Diagram Explanation
There is no visual diagram in the code. The focus is on string permutation logic, which must be completed inside the `permutation` function for the full functionality.](https://content.bartleby.com/qna-images/question/220fce40-52d4-4175-9c12-2aa3a603c4e5/609721dc-20aa-41c9-9a76-24da7a0af5ed/fhytksq_thumbnail.png)
Transcribed Image Text:```cpp
// { Driver Code Starts
#include <bits/stdc++.h>
using namespace std;
// } Driver Code Ends
class Solution{
public:
vector<string> permutation(string S){
// Code Here
}
};
// { Driver Code Starts.
int main(){
int t;
cin >> t;
while(t--){
string S;
cin >> S;
vector<string> ans;
Solution obj;
ans = obj.permutation(S);
for(int i=0; i < ans.size(); i++){
cout << "(" << ans[i] << ")";
}
cout << endl;
}
}
// } Driver Code Ends
```
### Explanation
This C++ code is designed to calculate permutations of a given string. Here's how the code is structured:
1. **Includes and Namespace:**
- `#include <bits/stdc++.h>`: Includes all standard C++ libraries.
- `using namespace std;`: Allows usage of standard library components without prefixing them with `std::`.
2. **Class Definition:**
- The class `Solution` contains a public member function `permutation`, which takes a string `S` as input and returns a `vector<string>`. The actual implementation of the permutation logic inside the function is missing (indicated by `// Code Here`).
3. **Main Function:**
- Reads an integer `t` which denotes the number of test cases.
- For each test case, it:
- Reads a string `S`.
- Creates an instance of `Solution`.
- Calls the `permutation` method to compute permutations of `S`.
- Prints each permutation in the format `(permutation)`, separated by space.
- Outputs a newline after printing all permutations for a test case.
### Diagram Explanation
There is no visual diagram in the code. The focus is on string permutation logic, which must be completed inside the `permutation` function for the full functionality.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps

Knowledge Booster
Similar questions
- Imagine that you are a biomedical engineer analyzing DNA sequences. You have numerical measurements from two different measurement sources, m1 and m2, both of which are arrays. Write a function named dna that takes these two arrays as inputs. It should return a character array string of nucleotides (represented by the letters A, C, G and T). For a given index, i, the nucleotide in the string is: - ‘A’ if m1(i) >= 0 and m2(i) >= 0 - ‘C’ if m1(i) < 0 and m2(i) >= 0 - ‘G’ if m1(i) >= 0 and m2(i) < 0 - ‘T’ if m1(i) < 0 and m2(i) < 0 code to call the function m1 = [-2 -3 2.5 0.3]; m2 = [1.1 2.1 -0.8 0.1]; sequecne = dna(m1,m2); function dna Please use MATLABarrow_forwardDefine a function named GetWordFrequency that takes a vector of strings and a search word as parameters. Function GetWordFrequency() then returns the number of occurrences of the search word in the vector parameter (case insensitive). Then, write a main program that reads a list of words into a vector, calls function GetWordFrequency() repeatedly, and outputs the words in the vector with their frequencies. The input begins with an integer indicating the number of words that follow. Ex: If the input is: 5 hey Hi Mark hi mark the output is: hey 1 Hi 2 Mark 2 hi 2 mark 2 Hint: Use tolower() to set the first letter of each word to lowercase before comparing. The program must define and use the following function:int GetWordFrequency(vector<string> wordsList, string currWord) write code in c++arrow_forwardExp2: A vectory is given by X = [−3.5 −5 6.2 11 0 8.1 −9 0 3 −1 3 2.5] Using conditional statement and loops, develop a program that creates two vectors from X: Vector P contains all positive elements of X Vector N contains the negative elements of X. The elements in new vectors are in the same order as in X. Please show me how to code this on matlab. I have tried watching tutorials on youtube and can not figure out how to code this for the life of me. Thank you.arrow_forward
- Integers are read from input and stored into a vector until 0 is read. If the vector's last element is odd, output the odd elements in the vector. Otherwise, output the even elements in the vector. End each number with a newline. Ex: If the input is -9 12 -6 1 0, the vector's last element is 1. Thus, the output is: -9 1 Note: (x % 2 != 0) returns true if x is odd. int value; int i; bool isodd; 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 } cin >> value; while (value != 0) { } for (i = 0; i > value; Check return 0; 1 Next level 2 X 1: Compare output 3 4 For input -9 12 -6 1 0, the vector elements are -9, 12, -6, and 1. The last element, 1, is odd. The odd elements in the vector, -9 and 1, are output, each on a new line. Not all tests passed. 2 V 3arrow_forwardYou may insert an element into an arbitrary position inside a vector using an iterator. Write a function, insert(vector, value) which inserts value into its sorted position inside the vector. The function returns the vector after it is modified. #include <vector>using namespace std; vector<int>& insert(vector<int>& v, int value){ ................ return v; }arrow_forwardIntegers are read from input and stored into a vector until 0 is read. If the vector's last element is odd, output the odd elements in the vector. Otherwise, output the even elements in the vector. End each number with a newline. Ex: If the input is -9 12 -6 1 0, the vector's last element is 1. Thus, the output is: -9 1 Note: (x % 2!= 0) returns true if x is odd. 4 5 int main() { 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20} vector vect1; int value; int i; bool isodd; cin >> value; while (value != 0) { vect1.push_back(value); cin >> value; } V* Your code goes here */ return 0; 2 3arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
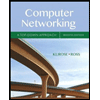
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
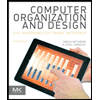
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
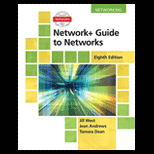
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
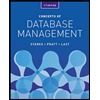
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
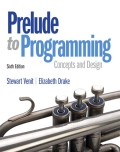
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
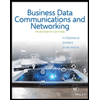
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY