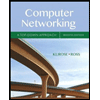
8.8 LAB: Course information (derived classes)
Given main(), define a Course base class with methods to set and get the courseNumber and courseTitle. Also define a derived class OfferedCourse with methods to set and get instructorName, term, and classTime.
Ex. If the input is:
ECE287 Digitalthe output is:
Course Information: Course Number: ECE287 Course Title: Digital Systems Design Course Information: Course Number: ECE387 Course Title: Embedded Systems Design Instructor Name: Mark Patterson Term: Fall 2018 Class Time: WF: 2-3:30 pmimport java.util.Scanner;
public class CourseInformation {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
Course myCourse = new Course();
OfferedCourse myOfferedCourse = new OfferedCourse();
String courseNumber, courseTitle;
String oCourseNumber, oCourseTitle, instructorName, term, classTime;
courseNumber = scnr.nextLine();
courseTitle = scnr.nextLine();
oCourseNumber = scnr.nextLine();
oCourseTitle = scnr.nextLine();
instructorName = scnr.nextLine();
term = scnr.nextLine();
classTime = scnr.nextLine();
myCourse.setCourseNumber(courseNumber);
myCourse.setCourseTitle(courseTitle);
myCourse.printInfo();
myOfferedCourse.setCourseNumber(oCourseNumber);
myOfferedCourse.setCourseTitle(oCourseTitle);
myOfferedCourse.setInstructorName(instructorName);
myOfferedCourse.setTerm(term);
myOfferedCourse.setClassTime(classTime);
myOfferedCourse.printInfo();
System.out.println(" Instructor Name: " + myOfferedCourse.getInstructorName());
System.out.println(" Term: " + myOfferedCourse.getTerm());
System.out.println(" Class Time: " + myOfferedCourse.getClassTime());
}
}
public class Course{
// TODO: Declare private fields - courseNumber, courseTitle
/*answer/*
// TODO: Define mutator methods -
// setCourseNumber(), setCourseTitle()
/*answer/*
// TODO: Define accessor methods -
// getCourseNumber(), getCourseTitle()
/*answer/*
// TODO: Define printInfo()
/*answer/*
}
public class OfferedCourse extends Course {
// TODO: Declare private fields - instructorName, term, classTime
/*answer/*
// TODO: Define mutator methods -
// setInstructorName(), setTerm(), setClassTime()
/*answer/*
// TODO: Define accessor methods -
// getInstructorName(), getTerm(), getClassTime()
/*answer/*
}

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 1 images

This isn't showing the full Expected Output, how do you get it to print the first course details that show only number & title?
This isn't showing the full Expected Output, how do you get it to print the first course details that show only number & title?
- solve the followingarrow_forwardKindly Solve this C++ question as per the instructions. Thank you for your help! Instructions: 1- Put the class definition in Flight.h and the implementation of the constructors and functions in Flight.cpp Implement the Flight.h and Flight.cpp so that class Flight contains: 4 private instance variables: Name of data type string, which describe the three electric vehicle charger types (AC975, DL521, and AC863). Destination of data type string that shows the final destination of that flight. Hour and Minute of data type int that show the departure time. A default constructor which sets all of the numeric instance variables to zero and the String instance variables to null. A constructor with 4 parameters that sets the 4 instance variables to the corresponding values passed. Implement an accessor method for each information (Flight name, Flight destination, and Flight Time) that will return the value of the instance variable. For example, the getX() method for the instance variables…arrow_forwardInstructions-Java Assignment is to define a class named Address. The Address class will have three private instance variables: an int named street_number a String named street_name and a String named state. Write three constructors for the Address class: an empty constructor (no input parameters) that initializes the three instance variables with default values of your choice, a constructor that takes the street values as input but defaults the state to "Arizona", and a constructor that takes all three pieces of information as input Next create a driver class named Main.java. Put public static void main here and test out your class by creating three instances of Address, one using each of the constructors. You can choose the particular address values that are used. I recommend you make them up and do not use actual addresses. Run your code to make sure it works. Next add the following public methods to the Address class and test them from main as you go: Write getters and…arrow_forward
- 17. Create a class ShuffleCipher that implements the interface MessageEncoder, as described in Exercise 15. The constructor should have one parameter called n. Define the method encode so that the message is shuffled n times. To perform one shuffle, split the message in half and then take characters from each half alternately. For example, if the message is abcdefghi, the halves are abcde and fghi. The shuffled message is afbgchdie. (Hint: You may wish to define a private method that performs one shuffle.)arrow_forwardJava:arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
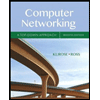
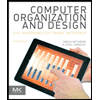
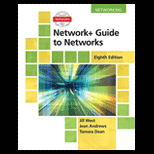
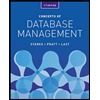
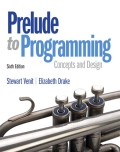
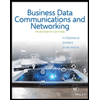