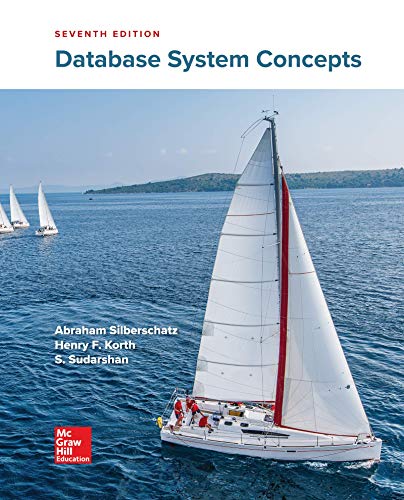
USE SIMPLE PYTHON CODE TO COMPLETE
Basic version with two levels of threads (master and slaves)
One master thread aggregates and sums the result of n slave-threads where each slavethread sums a different range of values in an array of 1000 random integers (please
program to generate 1000 random integers to populate the array).
The number of slave-threads is a parameter which the user can change. For example, if the
user chooses 4 slave threads, each slave thread will sum 1000/4 = 250 numbers. If the user
chooses 3 slave threads, the first two may each sum 333 numbers and the third slave thread
sums the rest 334 numbers.
2) Advanced version with more than two levels of threads
The master thread creates two slave-threads where each slave-thread is responsible to sum
half segment of the array.
Each slave thread will fork/spawn two new slave-threads where each new slave-thread
sums half of the array segment received by its parent. Each slave thread will return the
subtotal to its parent thread and the parent thread aggregates and returns the total to its
parent thread. Start with 7 nodes thread tree, when you are comfortable, you can extend it
to a full thread tree.
*** HERE IS MULTI PROCESSING REPLACE WITH MULTI THREADING PART 1 AND PART 2 PLEASE ***
BASIC VERSION PART 1
import multiprocessing
import random
def sum_range(arr, start, end):
#Calculates the sum of a range of values in an array.
return sum(arr[start:end])
def sum_array(arr, num_processes):
#Sums the values in an array using multiple processes.
pool = multiprocessing.Pool(num_processes)
chunk_size = len(arr) // num_processes
results = []
for i in range(num_processes):
start = i * chunk_size
end = start + chunk_size if i < num_processes - 1 else len(arr)
result = pool.apply_async(sum_range, args=(arr, start, end))
results.append(result)
total = sum(result.get() for result in results)
pool.close()
pool.join()
return total
if __name__ == '__main__':
num_processes = 4
arr = [random.randint(1, 100) for _ in range(1000)]
total = sum_array(arr, num_processes)
print(f'Total: {total}')
***MORE ADVANCE VERSION PART 2 BELOW***
import multiprocessing
import random
def sum_range(arr, start, end):
#Calculates the sum of a range of values in an array.
return sum(arr[start:end])
def sum_array(arr, num_processes):
#Sums the values in an array using a multi-level process tree.
if num_processes == 1:
return sum_range(arr, 0, len(arr))
else:
pool = multiprocessing.Pool(num_processes)
chunk_size = len(arr) // num_processes
results = []
for i in range(num_processes):
start = i * chunk_size
end = start + chunk_size if i < num_processes - 1 else len(arr)
result = pool.apply_async(sum_range, args=(arr, start, end))
results.append(result)
subtotals = [result.get() for result in results]
pool.close()
pool.join()
return sum_array(subtotals, num_processes // 2)
if __name__ == '__main__':
num_processes = 7
arr = [random.randint(1, 100) for _ in range(1000)]
total = sum_array(arr, num_processes)
print(f'Total: {total}')

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Write a java program named EmployeeRecords that simulates a company’s HR system. Theprogram reads a list of employee ids, names, and salaries from a file a3q1.txt, and stores theinformation in parallel array lists ids, names, and salaries.Sample input (a3q1.txt)132 Dumbledore 90000465 Lupin 60000443 McGonagall 75000124 Snape 70000337 Sprout 62000Your program will prompt the user to make a selection: retrieve an employee record by employeeID or display a salary report.You must include 2 methods:●retrieveEmployeeRecord(...) that accepts the array list of employee IDs and anemployee ID to find, and returns the index of the entry.arrow_forwardUsing multithreading, write a program that will add new items to your inventory, remove items that have been sold and view a list of all items in your inventory.arrow_forwardIn pythonarrow_forward
- In Python: Write a program that performs the following: Defines two sets s1, and s2, where each of them contain the following: s1 = {1,2,4,5,6} s2= {1,3,4,7} Provides a menu with the following options: Union Intersection Difference Symmetric difference Display sets Exit The program stops once the user enters 6 (chooses to exit). When the user selects any of the other options, the corresponding operation is performed and the result is displayed. Please refer to the attached code which contains an example on how to implement a menu. Notes: I will leave the code organization for you, at this stage, you should be able to organize your program into functions in a proper way For the third operation (difference) find s1-s2. For the remaining operations it does not matter (for example, s1 union s2 is same s2 union s1)arrow_forward2. The data array has the following numbers: 45,1,23,5,34,67 main bubble Up() OTTO A Yes Start k 0 firstUnsorted - 1 Loop first Unsorted-length_ of(data) - 1 No bubbleUp() firstUnsorted - firstUnsorted + 1 PUT K End What will be the output of this program? Yes swap() Start 16 Yes indexlength_of(data) Loop index=firstUns orted No data[index]arrow_forwardWrite a block of code that uses a dynamic allocated array named Array to (1) Load ten integers from the user into the memory, (2) Find the number of negative integers in the array, and (3) Print a message with the number of negatives. Whenever no numbers are negative print an additional message "No negatives!" (Assume all libraries are included)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
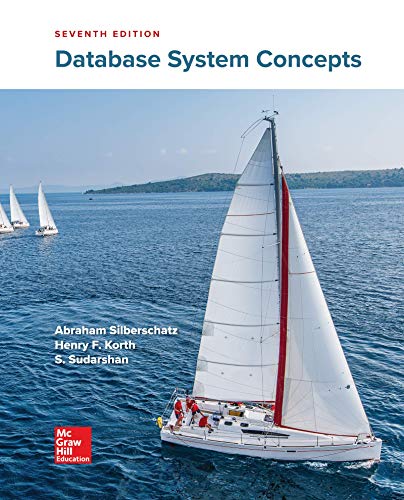
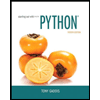
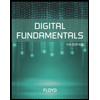
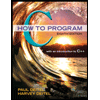
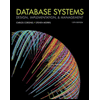
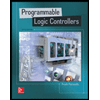