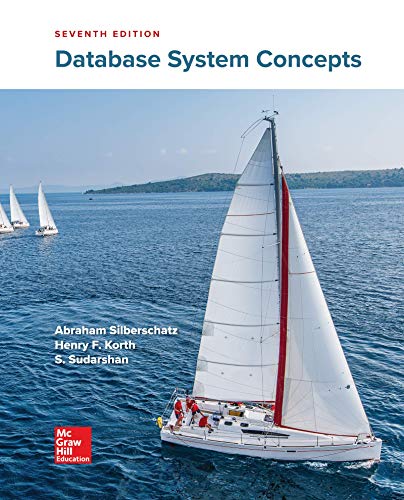
Use the logic in the program below to design a Recursive Descent parser in C
for the following grammar:
S →aAB
A →Abc | b
B →d
The program below is designed to parse the grammar below. Use the program below as a template and rewrite it so it can parse the grammar above
/*Recursive Descent Parser for the expression grammar
S -> (L) | a
L' -> ,SL | ε
L -> SL'
*/
#include <stdio.h>
#include <string.h>
int S(), Ldash(), L();
char *ip;
char string[50];
int main()
{
printf("Enter the string\n");
scanf("%s", string);
ip = string;
printf("\n\nInput\t\tAction\n ------------------------------\n");
if (S())
{
printf("\n------------------------------------------------\n");
printf("\n String is successfully parsed\n");
}
else
{
printf("\n ------------------------------------------------\n");
printf("Error in parsing string\n");
}
}
int L()
{
printf("%s\t\tL →SL' \n", ip);
if (S())
{
if (Ldash())
{
return 1;
}
else
return 0;
}
else
return 0;
}
int Ldash()
{
if (*ip == ',')
{
printf("%s\t\tL' →, SL' \n", ip);
ip++;
if (S())
{
if (Ldash())
{
return 1;
}
else
return 0;
}
else
return 0;
}
else
{
printf("%s\t\tL' →ε \n", ip);
return 1;
}
}
int S()
{
if (*ip == '(')
{
printf("%s\t\tS →(L) \n", ip);
ip++;
if (L())
{
if (*ip == ')')
{
ip++;
return 1;
}
else
return 0;
}
else
return 0;
}
else if (*ip == 'a')
{
ip++;
printf("%s\t\tS →a \n", ip);
return 1;
}
else
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 4 images

- S -> aSa | aXa X ->aXa | bYb Y -> bYb | empty where S is the start symbol, non-terminals = {S, X, Y}, terminals = {a, b} Convert this grammar to Chomsky Normal Form. Show the steps in this conversion.arrow_forwardSubject: Compiler Design Consider the following grammar and input string, S → S+S S → S*S S → (S) S → a Input string: a1*(a2+a3) Find out the parsing table using shift reduce parsing.arrow_forwardTopic: Parsing Problem 2. Consider the following context-free grammar What is the language L described by the grammar? Compute the first sets for S, X, and Y. You must show the iterations of the algorithm separately Compute the follow sets for S, X, and Y. You must show the iterations of the algorithm separately Construct the LL(1) parsing table for the grammar. It is enough to just show the table Show the steps in parsing the strings from Larrow_forward
- Design a DFA for a and c a) All words on Σ = {?, ?} that the last two letters are different. Example: ?????, ????? c) All words on Σ = {0, 1} that the remainder of the represented binary number by 8 is 5. Convert the DFAs you designed in part a and c of the first question to an equivalent regular grammar.arrow_forwardHere's the code: /* Recursive Descent Parser for the Expression Grammar:S → (L) |aL' →,SL'|εL → SL'Valid inputs: (a,(a,a)) and (a,((a,a),(a,a)))Invalid inputs:(aa,a)*/#include <stdio.h>#include <string.h>int S(), Ldash(), L();char *ip;char string[50];int main() {printf("Enter the string\n");scanf("%s", string);ip = string;printf("\n\nInput\t\tAction\n ------------------------------\n");if (S()){printf("\n------------------------------------------------\n");printf("\n String is successfully parsed\n");}else{printf("\n ------------------------------------------------\n");printf("Error in parsing string\n");}}int L(){printf("%s\t\tL →SL' \n", ip);if (S()){if (Ldash()){return 1;}elsereturn 0;} elsereturn 0;}int Ldash(){if (*ip == ','){printf("%s\t\tL' →, SL' \n", ip);ip++;if (S()){if (Ldash()){return 1;}elsereturn 0;}else return 0;}else{printf("%s\t\tL' →ε \n", ip);return 1;}}int S(){if (*ip == '('){ printf("%s\t\tS →(L)…arrow_forwardpart 4 and 5arrow_forward
- 3) To enforce the right associativity for a math operator in grammar, what property must production rules associated with that operator have? Choose one of the following options: a) Right recursive b) Left recursive c) Any form of recursion d) No recursionarrow_forwardfill out those two table?arrow_forwardhelp fill out the tablearrow_forward
- The correct statements are: OS→ Ta is allowed in a regular grammar. SaSa is allowed in a regular grammar. Sa → T is allowed in a regular grammar. OT→ aT is allowed in a regular grammar.arrow_forwardWhat language does the following grammar generate? Use set notation, English or regular expression to describe it. S->bA | b A->abA | abSarrow_forwardI need help developing a parser that can take a sentence generated by the context-free grammar and parse it into a parse tree, which can be used to represent the structure of the sentence. Like the example givenarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
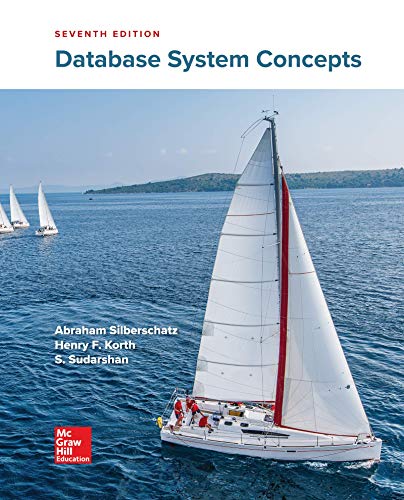
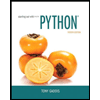
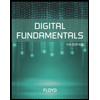
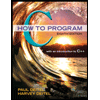
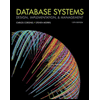
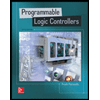