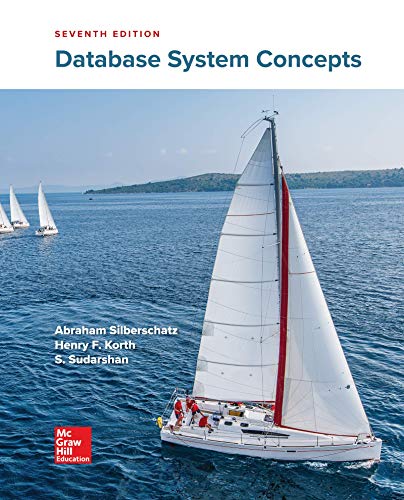
Python!!
Learning Objectives
- Use the while loop to repeat action an unknown number of times
- Practice input() statement inside the loop
- Learn complex string comparison condition inside the the while loop
- Create a function with a given name and parameter and given return values
- Use if/else statements inside the function to achieve different return statements by condition
- Use int() integer conversion function to have the number from string
Instructions
A keyboard can be really tricky! You try to enter a number but it prints a letter again and again! Let's try to fix that.
-
Create a function is_this_digit(input_line) that checks if the string parameter is a digit. The function should return True or False
1.1. String contains only one digit if the string length is 1 and the character inside is digit character. To check last condition use <target_var>.isdigit() function (e.g., "2".isdigit() will return True) or string comparison such as "0" <= target <= "9"
-
Read the input from the user (use input())
-
Continue to repeat reading the input, until user inputs digit
-
When we finally have a digit provided as an input, then output it in the following sentence: Your digit is DDD (where DDD is replaced by the provided digit).
Hints!
- When we cannot predict how many times will we need to repeat the action - that is when we use the while statement.
- Call your function inside the while statement or save your function result each time and use that result in while statement.
The code I should complete:
def is_this_digit(input_line):
# Define your function here
pass
if __name__ == '__main__':
# Type your code here.
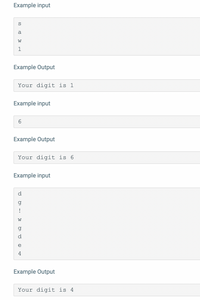

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- True or False: You should be incrementing the control variable of a for loop True or False: you can use the control variable of a while loop after the loop has finished executing True or False: you can nest repetition structures in repetition structures True or False: you can nest selection structure in selection structuresarrow_forwardcalcAverage function: This is function that returns no value and accept no parameters. This function will do the following: Ask the user to enter how many integers to enter. This has to be a positive integer and you need to validate that Write a “for” loop to accept this many integers and write the needed logic to calculate the average of these numbers and print that out Then the function will return back to the main functionarrow_forwardPython Assignment 4.2 Compute how well you did with your budget Create needed variables Input Get the monthly budget Process Get each individual monthly expense amount in a loop structure Total the expenses for the month within the loop Continue getting expenses until the user enters an “n” Once the loop is ended by the user entering an “n”, continue to then output below Output Display the budget amount and amount spent followed by one of the following messages: You are over budget, Plan better You are under budget, Great job You are on budget, Pure luck Sample Input and Output format: Enter your monthly budget: 100 Enter an amount spent: 65.49 Do you want to enter another expense? Enter y for yes, n for no): y Enter an amount spent: 58.21 Do you want to enter another expense? Enter y for yes, n for no): n Here is your budget results =========================== Amount budgeted: $100.00 Amount spent: $123.70 You are over budget by $23.70, Plan betterarrow_forward
- Computer Science 1st year Computer Science Abdulmuttalib T. Rashid Sheet No. 2 Q1: - Design a form and write a code to find the average value of N degrees using For Next Loop. Use InputBox function to read degrees. Q2: - Design a form and write a code to find the largest value of N numbers using Do While Loop. Use InputBox function to read numbers. Q3: - Write VB program using Do Loop While to read 7 marks, if pass in all marks (>=50) print "pass" otherwise print "fail". Use input box to read marks. Q4: - Write VB program using Do Until Loop to find the summation of student's marks, and it's average, assume the student have 8 marks. Use InputBox function to read marks. Q5: - Write VB program to read the degrees of computer science material, and find how many students are success, and how many students are failed. Use Do While Loop. Q6: - Write VB program to calculate the following equation. Use inbut box to read values of x and msgbox function to display the result values of y(x).…arrow_forwardIn C#arrow_forwardLooping Construct with Floating Point Numbers Write a program that utilizes a while-loop to read a set of five floating-point values from user input. Include code to prevent an endless loop. Ask the user to enter the values, then print the following data: Total Average Maximum Minimum Interest on total at 20% Answer:arrow_forward
- Learning Objectives: Python Programming Use loop over a stringReplace characters within a stringFunction (declaration and call)Write code in modular formInstructions Create a function only_chars that takes the line of text and returns the text line excluding the spaces, periods, exclamation points, or commas. Create a program that will read the input line, use the function, and then print the line, without spaces, periods, exclamation marks, or commas and their length. Ex: If the input is: Listen, Mr. Jones, calm down. the output is: ListenMrJonescalmdown21Do not forget if __name__ == "__main__" section!arrow_forwardprogram4_2.pyWrite a program that uses a while loop to enable the user to enter any number of positive integers. The loop should end when a sentinel value of 0 (zero) is entered. The program should then report the sum of the even integers and the sum of the odd integers.arrow_forwardTurtle Drawing with Loops Objective: To make a drawing using turtle graphics and nested loops Requirements: -Your program should ask the user at least two questions, and use the answers to help make the drawing. -Your program must have at least one pair of nested loops (a loop inside of a loop) to do some of the drawing -Your drawing cannot be made up only of rotated polygons coming from the center of the screen like we did in class. -Please write a new program for this assignment - don't use code from any in-class exercises. -For example, your program could draw a field of flowers, or you could draw a repetitive square pattern like you might see on a rug.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
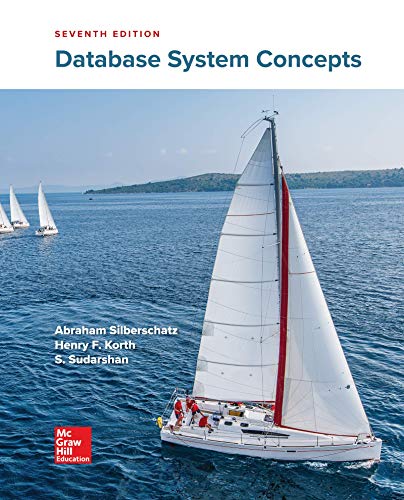
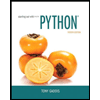
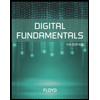
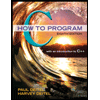
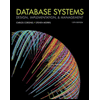
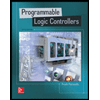