Using C++ write this code Write a function called isValidNote that accepts a string and returns true if that string is a valid note expressed in SPN and false otherwise. See the explanation above for clarity on what is and is not considered valid SPN. Function Specifications: Name: isValidNote() Parameters (Your function should accept these parameters IN THIS ORDER): note (string): The string to be checked Return Value: True or false (bool) The function should return true if the string is a valid note in SPN and false otherwise. The function should not print anything. The function should be case-sensitive, e.g. B0 is valid SPN but b0 is not. Hint: Any note expressed in valid SPN will be exactly 2 characters long. --- Examples --- Sample function call Expected return value isValidNote("y") False isValidNote("D4") True isValidNote("d4") False isValidNote("E72") False isValidNote("I love coding") False Your file should be named isValidNote.cpp and should also include a main function that tests your isValidNote function. Once you have finished developing your solution in VSCode you should head over to the CodeRunner on Canvas and paste only your function isValidNote into the answer box for question 1. You do not need to paste your main function into Coderunner, one has already been provided for you. You will need to include your main and isValidNote functions in the isValidNote.cpp file that you submit to Canvas.
Using C++ write this code
Write a function called isValidNote that accepts a string and returns true if that string is a valid note expressed in SPN and false otherwise. See the explanation above for clarity on what is and is not considered valid SPN.
Function Specifications:
- Name: isValidNote()
- Parameters (Your function should accept these parameters IN THIS ORDER):
- note (string): The string to be checked
- Return Value: True or false (bool)
- The function should return true if the string is a valid note in SPN and false otherwise.
- The function should not print anything.
- The function should be case-sensitive, e.g. B0 is valid SPN but b0 is not.
Hint: Any note expressed in valid SPN will be exactly 2 characters long.
--- Examples ---
Sample function call | Expected return value |
---|---|
isValidNote("y") | False |
isValidNote("D4") | True |
isValidNote("d4") | False |
isValidNote("E72") | False |
isValidNote("I love coding") | False |
Your file should be named isValidNote.cpp and should also include a main function that tests your isValidNote function. Once you have finished developing your solution in VSCode you should head over to the CodeRunner on Canvas and paste only your function isValidNote into the answer box for question 1. You do not need to paste your main function into Coderunner, one has already been provided for you. You will need to include your main and isValidNote functions in the isValidNote.cpp file that you submit to Canvas.
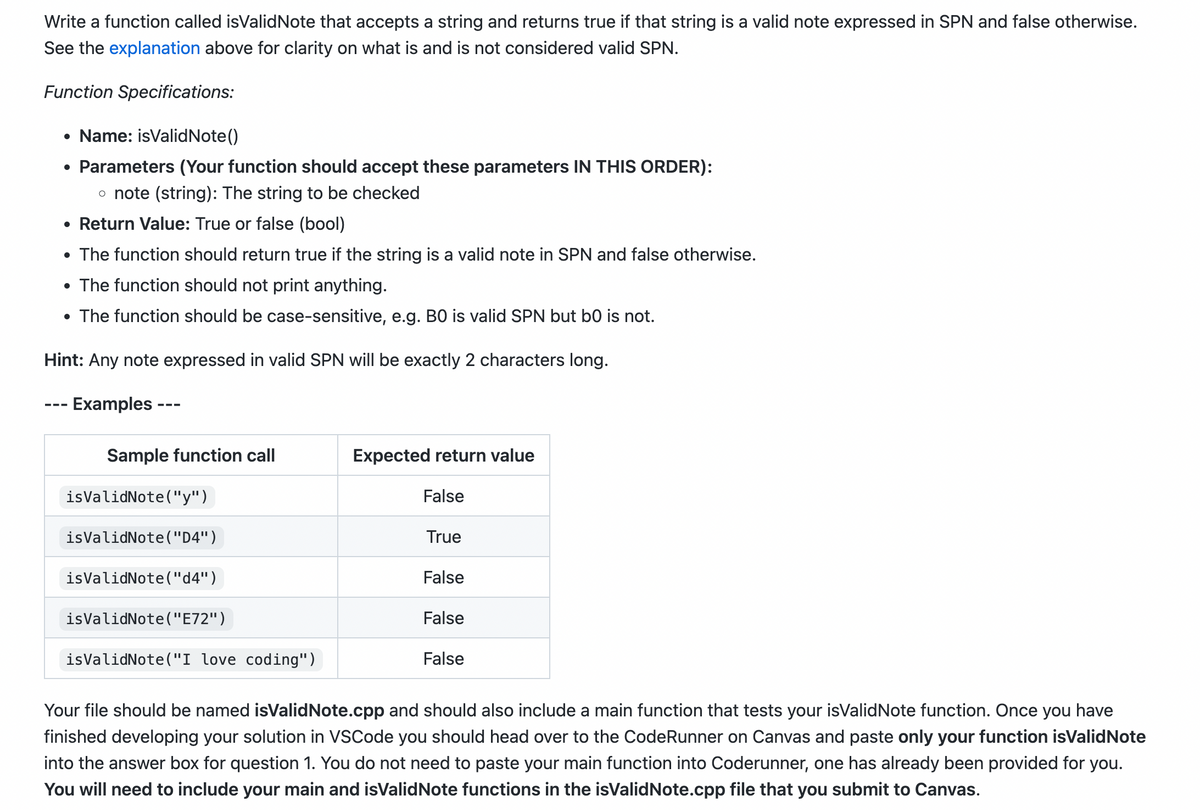

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

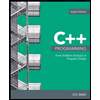
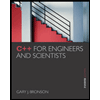
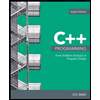
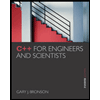