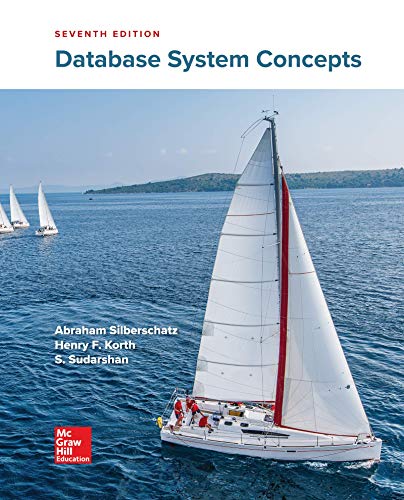
Using OpenGL I need help making a 3d pyramid In C++ using my existing code and without adding any additional libs. Currently, I have a 3d Cube and I need to convert this code into a 3d pyramid.
Your pyramid should use the indices and the Vertex Array Object, with each vertex on the pyramid being assigned a color of your choice. Employ the Model View Projection matrix to display the pyramid in a perspective angle.
#include <iostream> // cout, cerr
#include <cstdlib> // EXIT_FAILURE
#include <GL/glew.h> // GLEW library
#include <GLFW/glfw3.h> // GLFW library
// GLM Math Header inclusions
#include <glm/glm.hpp>
#include <glm/gtx/transform.hpp>
#include <glm/gtc/type_ptr.hpp>
using namespace std; // Standard namespace
void UCreateMesh(GLMesh &mesh)
{
// Specifies screen coordinates (x,y) and color for triangle vertices
GLfloat verts[]=
{
// Vertex Positions // Colors
0.5f, 0.5f, 0.0f, 1.0f, 0.0f, 0.0f, 1.0f, // Top Right Vertex 0
0.5f, -0.5f, 0.0f, 0.0f, 1.0f, 0.0f, 1.0f, // Bottom Right Vertex 1
-0.5f, -0.5f, 0.0f, 0.0f, 0.0f, 1.0f, 1.0f, // Bottom Left Vertex 2
-0.5f, 0.5f, 0.0f, 1.0f, 0.0f, 1.0f, 1.0f, // Top Left Vertex 3
0.10f, 0.10f, 0.0f, 1.0f, 0.0f, 0.0f, 1.0f, // Top Right Vertex 0
0.5f, -0.5f, 0.0f, 0.0f, 1.0f, 0.0f, 1.0f, // Bottom Right Vertex 1
-0.5f, -0.5f, 0.0f, 0.0f, 0.0f, 1.0f, 1.0f, // Bottom Left Vertex 2
-0.5f, 0.5f, 0.0f, 1.0f, 0.0f, 1.0f, 1.0f // Top Left Vertex 3
};
const GLuint floatsPerVertex = 3;
const GLuint floatsPerColor = 4;
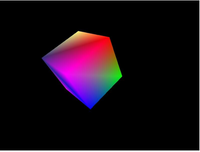
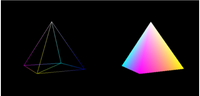

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Write a WebGL program that produces a Colored Cube.arrow_forwardUse Python Codehow to filter dataframes using python . For example I have a student grade csv table, first, I need to read the csv using pandas library then I want to visualize my student scores (using matplotlib, seaborn, etc) but I only want to visualize those whose scores are 60 and above. so how do i do the code in python.Exampe of Student grades table (csv) : Student name Score Jojo 90 Lili 65 Bibi 50 Sarah 40 Rajah 100 Albert 80 Cherry 75 Jamie 85 So , based on the table above, what doesn't need to be visualized is Bibi and Sarah . Hope you can help me :)arrow_forwardUsing comments within the code itself, can you provide an line by line explanation of the below JavaScript file? The file itself deals with WebGl and if that helps you. Please & thank you JavaScript File: function inverse2(m) { var a = mat2(); var d = det2(m); a[0][0] = m[1][1]/d; a[0][1] = -m[0][1]/d; a[1][0] = -m[1][0]/d; a[1][1] = m[0][0]/d; return a; } function inverse3(m) { var a = mat3(); var d = det3(m); var a00 = [ vec2(m[1][1], m[1][2]), vec2(m[2][1], m[2][2]) ]; var a01 = [ vec2(m[1][0], m[1][2]), vec2(m[2][0], m[2][2]) ]; var a02 = [ vec2(m[1][0], m[1][1]), vec2(m[2][0], m[2][1]) ]; var a10 = [ vec2(m[0][1], m[0][2]), vec2(m[2][1], m[2][2]) ]; var a11 = [ vec2(m[0][0], m[0][2]), vec2(m[2][0], m[2][2]) ]; var a12 = [ vec2(m[0][0], m[0][1]), vec2(m[2][0], m[2][1]) ]; var a20 = […arrow_forward
- Build on our PBM image creator from (code shown below) to create a fully functional image editor (in C++), using 2D arrays to store our changes. To make it more interesting by switching to the Portable Gray Map standard. 8 bit PGM files have color values ranging from 0 (black) to 255 (white), with the values in-between being shades of gray. The header is very similar to PBM, and looks like this: P2 WIDTH HEIGHT 255 IMAGE_DATA The P2 tells image readers that it is a PGM file, and the 255 indicates the largest possible color value is 255. You will first prompt the user to enter a width, height, and initial color value to fill the image. You should then create a 2D array with those dimensions and initialize each cell with that color value. You will then use a loop to create a menu that repeatedly prompts the user to make one of the following choices: Fill in a pixel with a new color ◦ Prompt the user to enter a row and column value and a new color value. If the array has that row and…arrow_forwardOpenGL Programming Help Model the “block IU” logo. Where the "I" is in front of the "U" Use appropriate colors and style. You arerequired to use display lists to create these letters. Animate your scene so that when the user clicks the RMB (right mouse button) andselects the menu option to spin the “I”, the “I” spins vertically. Depending on how youspecified your vertices, this could require a translation to the origin, a rotation, and a translation back. When the user clicks on the RMB and selects the menu option to spin the “U”, the “I” should stop spinning and the “U” should spin in the same manner. At no point should both letters spin at the same time. The program should quit when the user presses either “q” or “Q” or selects the RMB menu option to quit.arrow_forwardThe Koch snowflake is a fractal shape. At level 0, the shape is an equilateral triangle. At level 1, each line segment is split into four equal parts, producing an equilateral bump in the middle of each segment. Figure 7-15 shows these shapes at levels 0, 1, and 2. . Python Turtie Graphics O0. Python Turtle Graphics O0. Python Turtie Graphics Figure 7-15 First three levels of a Koch snowflake Figure 7-15 First three levels of a Koch snowflake At the top level, the script uses a function drawFractalLine to draw three fractal lines. Each line is specified by a given distance, direction (angle), and level: • The initial angles are 0, -12O, and 120 degrees. • The initial distance can be any size, such as 200 pixels. • If the level is O, then the turtle moves the given distance in the given direction. Otherwise, the function draws four fractal lines with % of the given distance, angles that produce the given effect, and the given level minus 1. The function drawFractalLine is recursive.…arrow_forward
- Hello, I am stuggling with this specfic problem and the specific parts as well, can you please help me get a solution for this problem. I only need help with part B and part C because I don't understand how to do those problems. Can you please show the regular expression to NFAs in a visual representation thank you. Again I only need help with part B and C and can you label the parts as well, so I know which part is part B and which one is part C.arrow_forwardHow to use API methods to write a polygon transformation?arrow_forwardPlease helpme to write the OpenGl glut codes to design a polygon. The codes need to have a function for applying arrow keys to move the polygon, to left, right, up, and down.and also apply keyboard function to change polygon in three different colours and quit program.arrow_forward
- Please anwser the following question regarding ios app development.arrow_forwardOpenGL Programming (Show screenshot to prove it works)arrow_forwardWhich function is used to draw OpenGL primitives? giLoadidentity): glutinit glvertex gIMatrixMode What is transformation? O A function supplied by OpenGL that can morph one polygon to another. Switching between different buffers. The mathematical operation used to create the desired behavior for points and objects.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
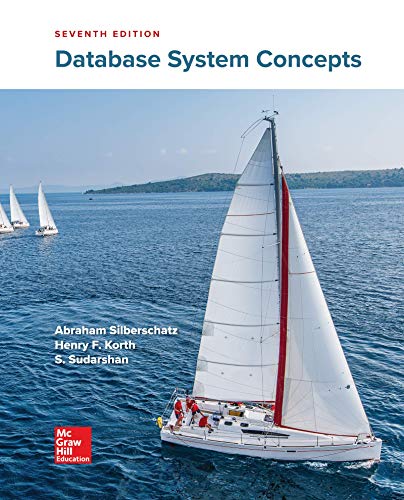
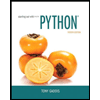
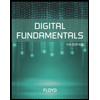
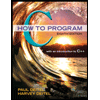
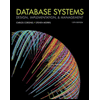
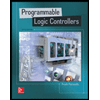