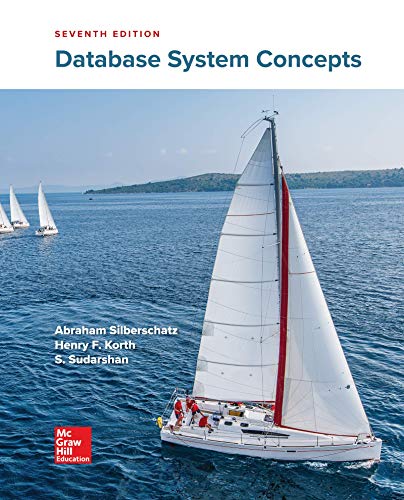
USING SIMPLE JAVA CODE TAKE GIVEN CODE AND MODIFY
MODIFY GIVEN CODE USING JAVA RECURSIVE ACTION
DO THE SAME JOB BUT USING RECURSIVE ACTION INSTEAD OF RECURSIVE TASK
SAMPLE CODE BELOW
*********************************************************************************************************
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.RecursiveTask; //replace with recursive action to do same job below//
public class SumWithPool{
public static void main(String[] args) throws InterruptedException,
ExecutionException {
//get the number of avaialbe CPUs
int nThreads = Runtime.getRuntime().availableProcessors();
System.out.println("Available CPUs: " + nThreads);
//create an array of data
int n = 10; //initital data size
if(args.length > 0) // use the user given data size
n = Integer.parseInt(args[0]);
int[] numbers = new int[n];
for(int i = 0; i < numbers.length; i++) {
numbers[i] = i;
}
ForkJoinPool forkJoinPool = new ForkJoinPool(nThreads);
Sum s = new Sum(numbers,0,numbers.length);
long startTime = System.currentTimeMillis(); //start timer
Long result = forkJoinPool.invoke(s);
long endTime = System.currentTimeMillis();
long timeElapsed = endTime - startTime;
System.out.println("Concurrent executing time: " + timeElapsed + "ms");
System.out.println("The conccurent sum: " + result);
//redo the task sequentially
startTime = System.currentTimeMillis(); //start timer
result = sum(numbers);
endTime = System.currentTimeMillis();
timeElapsed = endTime - startTime;
System.out.println("\nSequential executing time: " + timeElapsed + "ms");
System.out.println("The sequential sum: " + result);
}
/** sequential sum */
private static long sum(int[] arr){
long sum = 0;
for(int i = 0; i < arr.length; i++)
sum += arr[i];
return sum;
}
}
/** create a class that handle a task */
class Sum extends RecursiveTask<Long> {
private int low;
private int high;
private int[] array; //only reference to the given data
/** map the attribute to the given data */
public Sum(int[] array, int low, int high) {
this.array = array;
this.low = low;
this.high = high;
}
/** overriding tehe comput method */
protected Long compute() {
if(high - low <= 10) { //do the sum if the block of data is managable
long sum = 0;
for(int i = low; i < high; ++i)
sum += array[i];
return sum;
} else {
int mid = low + (high - low) / 2;
Sum left = new Sum(array, low, mid);
Sum right = new Sum(array, mid, high);
left.fork();
long rightResult = right.compute();
long leftResult = left.join();
return leftResult + rightResult;
}
}
}

Step by stepSolved in 2 steps with 1 images

- Complete the following program Multiply.java. This program uses recursion to multiply two numbers through repeated addition. The output should look exactly like what is pictured below. The code should be completed where it states "complete here"arrow_forwardWrite java program recursive method to display all odd numbers from the given number to 1 .arrow_forwardImplement the recursive function for the Fibonacci code programming language: java Need full code with explanationarrow_forward
- Recursive definitions Give the recursive definition for the following languages: (a) L2a = {w = {0, 1}* |w has 00 as a substring} (b) L₁₁ = {w= {0,1}* |w all strings with alternating O's and 1's} (c) L2c = {w = {0, 1}* |w has a equal number of 0's and 1's}arrow_forwardjava A time series is represented a list of time/value pairs Write a function that takes two time series and outputs a new series of the summation of themarrow_forwardJava utilises the terms counter controlled loop, flag while loop, instance methods, inner classes, shallow copying, and deep copying.arrow_forward
- n-1 Geometric (n) = i=1 i=1 1 1 * n-1 П %3D Harmonic (n) = i=1 n Let's look at examples. If we use n = 4, the geometric progression would be 1 * 2 * 3 * 4 = 24, and the harmonic 1.1.1 1 progression would be 1* -= 0.04166. 2 3 4 24 Task #1 Tracing Recursive Methods 1. Copy the file Recursion.java (see Code Listing 16.1) from the Student Files or as directed by your instructor. 2. Run the program to confirm that the generated answer is correct. Modify the factorial method in the following ways: a. Add these lines above the first if statement: int temp; System.out.println ("Method call -- " + "calculating " "Factorial of: " + n); + Copyright © 2019 Pearson Education, Inc., Hoboken NJ b. Remove this line in the recursive section at the end of the method: return (factorial (n - 1) * n); c. Add these lines in the recursive section: temp = factorial (n - 1); System.out.println ("Factorial of: " (n - 1) + " is " temp); return (temp * n); 3. Rerun the program and note how the recursive calls…arrow_forwardUsing recursion, write a Java program that takes an input ‘n’ (a number) from a user to calculate and print out the Fibonacci using the following modified definition: F(N) = 1 if n = 1 or n = 2 = F((n+1)/2)2 + F((n-1/2)2 if n is odd = F(n/2 + 1)2 – F(n/2 – 1)2 if n is even Your solution must implement recursion to receive points for this question.arrow_forwardTry to do it asaparrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
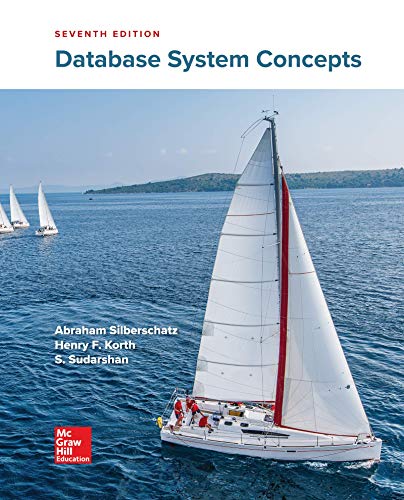
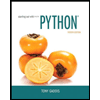
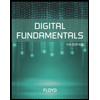
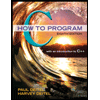
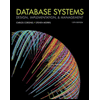
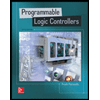