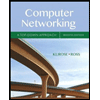
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Using Single-Row and Aggregate (Multi-Row) Functions
*/
--==============================================
-- SECTION 1
--
--
-- Single-Row Functions
--==============================================
/*
1. Use the CONCAT function (not the || operator) to concatenate the customers’
last and first names into one column.
Leave a ', ' between names.
Give the column an alias: "Customer Full Name".
Sort your results by the column alias.
*/
/*
2. Write a query using the SUBSTR function to display a list
of distinct area codes (first 3 digits of phone number) for employees.
Name the column “Area Codes”.
Sort your results by the column alias.
*/
/*
3. Write a query using the LENGTH function to display a list of books with
titles
that are longer than 50 characters.
Name the column “Long Titles”.
Also, display the book titles of these books.
Sort your results by longest title first and then by title.
*/
/*
4. Write a query using the MOD function to display a list of employees with
salaries that are odd numbers.
[You may need to look up Oracle's MOD() function.]
For these employees, display
Last name
First name
Current salary
Using Oracle NVL() function
[Look up the Oracle NVL() function to find out how it works.]
Display Phone first and name the column as "Phone First"
Display Email first and name the column as "Email First"
Sort results by last and first names.
*/
/*
5. Write a query to calculate years of service for each employee.
*/
--==============================================
-- SECTION 1
--
--
-- Single-Row Functions
--==============================================
/*
1. Use the CONCAT function (not the || operator) to concatenate the customers’
last and first names into one column.
Leave a ', ' between names.
Give the column an alias: "Customer Full Name".
Sort your results by the column alias.
*/
/*
2. Write a query using the SUBSTR function to display a list
of distinct area codes (first 3 digits of phone number) for employees.
Name the column “Area Codes”.
Sort your results by the column alias.
*/
/*
3. Write a query using the LENGTH function to display a list of books with
titles
that are longer than 50 characters.
Name the column “Long Titles”.
Also, display the book titles of these books.
Sort your results by longest title first and then by title.
*/
/*
4. Write a query using the MOD function to display a list of employees with
salaries that are odd numbers.
[You may need to look up Oracle's MOD() function.]
For these employees, display
Last name
First name
Current salary
Using Oracle NVL() function
[Look up the Oracle NVL() function to find out how it works.]
Display Phone first and name the column as "Phone First"
Display Email first and name the column as "Email First"
Sort results by last and first names.
*/
/*
5. Write a query to calculate years of service for each employee.
Calculate years of service in two ways and display them as two separate
columns.
1. SYSDATE-HIRE_DATE and name the column "Years of Service 1"
2. MONTHS_BETWEEN() function and name the column "Years of Service 2"
BE SURE YOUR RESULTS ARE IN YEARS.
Round each result to integers (whole numbers).
Display
Years of Service 1
Years of Service 2
Last Name
First Name
Sort results so the employee with most years of service displays first.
*/
/*
6. Write a SQL statement using CASE to classify employees into salary bands
based on their salary:
• LOW = 0 – 5000
• MID = 5000 – 10000
• HIGH = 10001 – 50000
Name the result of the CASE statement "Salary Band"
Your results should display the employees’ name, salary, and salary band.
Sort your results by salary descending and and salary band, then last and first
names.
*/
--==============================================
-- SECTION 2
--
-- Aggregate (Multi-Row) Functions
--==============================================
/*
/*
1. Write a query using the COUNT function to display the number of employees in
each department.
These employees should have been hired between
January 1, 1990 and December 31, 1995.
Your results should be listed and sorted by department name (not
Department_ID).
Display
Department Name
Employee Count
*/
/*
2. Write a query using the MIN, MAX, and AVG functions to display
the minimum, maximum, and average salaries of all employees.
Your results should return 3 numbers in 1 row.
*/
/*
columns.
1. SYSDATE-HIRE_DATE and name the column "Years of Service 1"
2. MONTHS_BETWEEN() function and name the column "Years of Service 2"
BE SURE YOUR RESULTS ARE IN YEARS.
Round each result to integers (whole numbers).
Display
Years of Service 1
Years of Service 2
Last Name
First Name
Sort results so the employee with most years of service displays first.
*/
/*
6. Write a SQL statement using CASE to classify employees into salary bands
based on their salary:
• LOW = 0 – 5000
• MID = 5000 – 10000
• HIGH = 10001 – 50000
Name the result of the CASE statement "Salary Band"
Your results should display the employees’ name, salary, and salary band.
Sort your results by salary descending and and salary band, then last and first
names.
*/
--==============================================
-- SECTION 2
--
-- Aggregate (Multi-Row) Functions
--==============================================
/*
/*
1. Write a query using the COUNT function to display the number of employees in
each department.
These employees should have been hired between
January 1, 1990 and December 31, 1995.
Your results should be listed and sorted by department name (not
Department_ID).
Display
Department Name
Employee Count
*/
/*
2. Write a query using the MIN, MAX, and AVG functions to display
the minimum, maximum, and average salaries of all employees.
Your results should return 3 numbers in 1 row.
*/
/*
3. The company is performing a budget analysis.
They need to determine how much they currently spend on employee salaries in
each department.
For each department, use the appropriate aggregate functions
to display the following:
Department Name
Department Manager ID
Minimum Salary
Average Salary
Maximum Salary
Total Salary
Number of Department Employees
Sort your results to display the department with the highest total salary
first.
*/
/*
4. Revise Q3 to select only those departments with 3 or more employees.
*/
/*
5. Write a query using the COUNT function to display the number of customers
that placed orders.
(NOT the number of Orders).
Your results should return 1 number.
*/
/*
6. VERY DIFFICULT
Write a query to calculate the order total for each order. There should be 14
Orders.
IMPORTANT NOTE: You CANNOT use Order_Total in HOL_ORDERS because it contains
NULL values.
You will need to determine another way to calculate Order Total.
Your results should display
Order number
Order date
Customer last name
Customer first name
Order total
Display the order total as “Order Total”.
You will need to use SUM and GROUP BY.
Sort your results so the highest order total displays first.
*/
They need to determine how much they currently spend on employee salaries in
each department.
For each department, use the appropriate aggregate functions
to display the following:
Department Name
Department Manager ID
Minimum Salary
Average Salary
Maximum Salary
Total Salary
Number of Department Employees
Sort your results to display the department with the highest total salary
first.
*/
/*
4. Revise Q3 to select only those departments with 3 or more employees.
*/
/*
5. Write a query using the COUNT function to display the number of customers
that placed orders.
(NOT the number of Orders).
Your results should return 1 number.
*/
/*
6. VERY DIFFICULT
Write a query to calculate the order total for each order. There should be 14
Orders.
IMPORTANT NOTE: You CANNOT use Order_Total in HOL_ORDERS because it contains
NULL values.
You will need to determine another way to calculate Order Total.
Your results should display
Order number
Order date
Customer last name
Customer first name
Order total
Display the order total as “Order Total”.
You will need to use SUM and GROUP BY.
Sort your results so the highest order total displays first.
*/
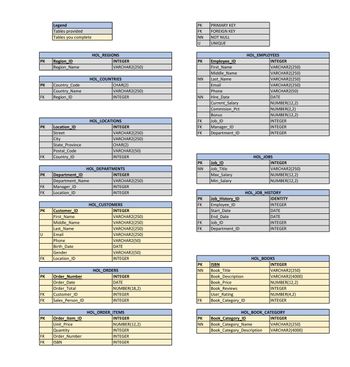
Transcribed Image Text:PK
PK
FK
PK
FK
FK
FK
PK
U
PK Department_ID
FK
PK
Legend
Tables provided
Tables you complete
Region_ID
Region_Name
PK
Country_Code
Country Name
Region_ID
Location ID
Street
City
State Province
Postal Code
Country_ID
Department_Name
Manager_ID
Location_ID
Customer ID
First Name
Middle Name
Last Name
Email
Phone
Birth Date
Gender
Location ID
Order Number
Order Date
Order Total
Customer ID
FK
FK Sales_Person_ID
Order Item ID
Unit Price
Quantity
Order Number
FK
FK ISBN
HOL_REGIONS
INTEGER
VARCHAR2(250)
HOL_COUNTRIES
CHAR(2)
VARCHAR2(250)
INTEGER
HOL_LOCATIONS
INTEGER
VARCHAR2(250)
VARCHAR2(250)
CHAR(2)
VARCHAR2(50)
INTEGER
HOL_DEPARTMENTS
INTEGER
VARCHAR2(250)
INTEGER
INTEGER
HOL_CUSTOMERS
INTEGER
VARCHAR2(250)
VARCHAR2(250)
VARCHAR2(250)
VARCHAR2(250)
VARCHAR2(50)
DATE
VARCHAR2(50)
INTEGER
HOL_ORDERS
INTEGER
DATE
NUMBER(18,2)
INTEGER
INTEGER
HOL_ORDER_ITEMS
INTEGER
NUMBER(12,2)
INTEGER
INTEGER
INTEGER
PK
FK
NN
U
PK
NN
NN
FK
FK
FK
PK
NN
FK
FK
PK
NN
PRIMARY KEY
FOREIGN KEY
NOT NULL
UNIQUE
FK
Employee_ID
First Name
Middle_Name
Last Name
Email
Phone
Hire Date
Current_Salary
Commision Pct
Bonus
Job ID
Manager_ID
Department_ID
PK Job_History_ID
FK
Employee_ID
Start Date
End Date
Job ID
Department_ID
Job ID
Job Title
Max_Salary
Min_Salary
-
ISBN
Book Title
Book Description
Book_Price
Book Reviews
User Rating
Book_Category_ID
HOL_EMPLOYEES
INTEGER
VARCHAR2(250)
VARCHAR2(250)
VARCHAR2(250)
VARCHAR2(250)
VARCHAR2(50)
DATE
NUMBER(12,2)
NUMBER(2,2)
NUMBER(12,2)
INTEGER
INTEGER
INTEGER
HOL_JOBS
INTEGER
VARCHAR2(250)
NUMBER(12,2)
NUMBER(12,2)
HOL_JOB_HISTORY
IDENTITY
INTEGER
DATE
DATE
INTEGER
INTEGER
HOL BOOKS
INTEGER
VARCHAR2(250)
VARCHAR2(4000)
NUMBER(12,2)
INTEGER
NUMBER(4,2)
INTEGER
HOL_BOOK_CATEGORY
PK Book_Category_ID
INTEGER
NN Book_Category_Name
VARCHAR2(250)
Book_Category_Description VARCHAR2(4000)
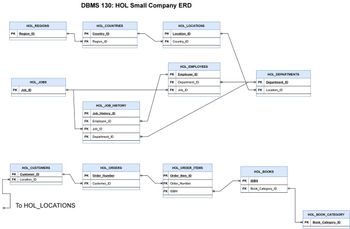
Transcribed Image Text:H
HOL_REGIONS
PK Region ID
HOL_JOBS
PK Job ID
HOL_CUSTOMERS
PK Customer ID
KFK Location_ID
开
H+
++
To HOL_LOCATIONS
DBMS 130: HOL Small Company ERD
HOL_COUNTRIES
PK Country_ID
FK Region_ID
HOL_JOB_HISTORY
PK Job History_ID
FK Employee_ID
FK Job_ID
FK Department_ID
HOL_ORDERS
PK Order Number
FK Customer_ID
H+
++
HOL_LOCATIONS
PK Location ID
FK Country_ID
HOL_EMPLOYEES
HPK Employee_ID
FK Department_ID
FK Job_ID
HOL_ORDER_ITEMS
PK Order Item ID
FK ISBN
KFK Order_Number
H
+ PK
HOL_DEPARTMENTS
HPK Department_ID
FK Location_ID
HOL_BOOKS
ISBN
FK Book_Category_ID
+H
HOL_BOOK_CATEGORY
KPK Book_Category_ID
Expert Solution

arrow_forward
Introduction
Hi. As per my company rule i can answer the first 3 questions only. You can post remaining as new question
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- matlab code pleasearrow_forwardComp Architecture Problem 7 and 8arrow_forwardConsider a DRAM chip of capacity 256 KB and each memory location contains 8 bits. The memory chip is organized in matrix form with equal number of rows and column for each memory location of 8 bits. This DRAM chip has a refresh interval of 64 ms, memory bus runs at 200 MHz, and the refresh cycle takes 4 clock cycle. a) Time required to refresh the DRAM chip. b) What is the minimum size of the refresh counter?arrow_forward
- Convert the following a sequential circuit in pipelined design Calculate the throughput (T) and latency (L) What is the bottleneck block in the given design? Explain circuit interleaving and apply it into the given circuit for solving the bottleneck issue.arrow_forwardHelp me with this computer science homework Not gradedarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
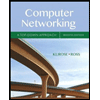
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
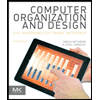
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
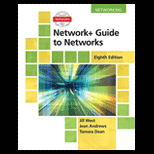
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
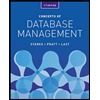
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
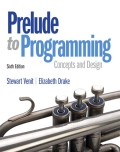
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
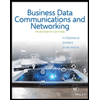
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY