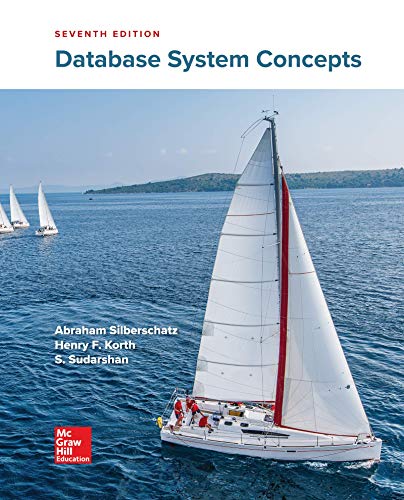
n C# i need to
In your IDE, copy the program BorderDemo2 (in picture)
After doing so, create an overload of the DisplayWithBorder() method accepting a double type variable. Modify this method so that it works exactly like the other two versions. For example, DisplayWithBorder(3.25) would output: *3.25*
In your main method, add in the lines
DisplayWithBorder(3.25);
DisplayWithBorder(2430.49734);
this is my code
using System;
using static System.Console;
class BorderDemo2
{
static void Main()
{
DisplayWithBorder("Ed");
DisplayWithBorder (3);
DisplayWithBorder (456);
DisplayWithBorder (897654);
DisplayWithBorder("Veronica");
DisplayWithBorder(3.25);
DisplayWithBorder(2430.49734);
}
private static void DisplayWithBorder(string word)
{
const int EXTRA_STARS = 4;
const string SYMBOL = "*";
int size = word.Length + EXTRA_STARS;
int x;
for (x = 0; x < size; ++x)
{
Write(SYMBOL);
}
WriteLine();
WriteLine(SYMBOL + " " + word + " " + SYMBOL);
for (x=0; x < size; ++x)
{
Write(SYMBOL);
}
WriteLine("\n\n");
}
private static void DisplayWithBorder(int number)
{
const int EXTRA_STARS = 4;
const string SYMBOL = "*";
int size = EXTRA_STARS + 1;
int leftover = number;
int x;
while(leftover >= 10)
{
leftover = leftover / 10;
++size;
}
for (x=0; x < size; ++x)
{
Write(SYMBOL);
}
WriteLine();
WriteLine(SYMBOL + " " + number + " " + SYMBOL);
for (x=0; x < size; ++x)
{
Write(SYMBOL);
}
WriteLine("\n\n");
}
private static void DisplayWithBorder(double number)
{
const int EXTRA_STARS = 4;
const string SYMBOL = "*";
int size = (int)Math.Floor(Math.Log10(Math.Abs(number))) + EXTRA_STARS + 1;
int x;
for (x=0; x < size; ++x)
{
Write(SYMBOL);
}
WriteLine();
WriteLine(SYMBOL + " " + number + " " + SYMBOL);
for (x=0; x < size; ++x)
{
Write(SYMBOL);
}
WriteLine("\n\n");
}
}
I keep getting the error
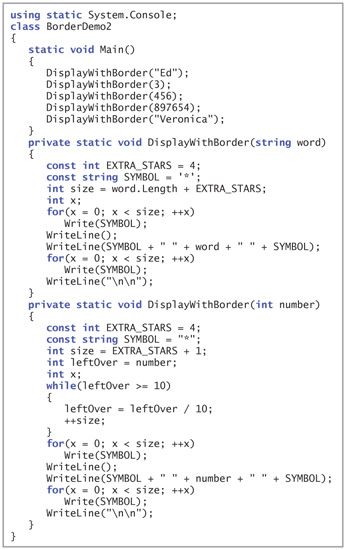

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- Needs to be written in java: Write a program with a main() method that asks the user to input an integer array of 10 elements.Next, create three methods described below. From inside your main() method, call each of thethree methods described below and print out the results of the methods 2, 3, which return values.1. printReverse() - a method that receives an array of integers, then reverses the elements ofthe array and prints out all the elements from inside the method. Print all in one lineseparated by commas (see sample output below).2. getLargest() – a method that receives an array of integers, then returns the largest integervalue in the array. (print result from main())3. computeTwice()- a method that receives the previously reversed array of integers, thenreturns an array of integers which doubles the value of each number in the array (see thesample output below). (print result from main())Sample output:Enter a number:22Enter a number:34Enter a number:21Enter a number:35Enter a…arrow_forwardWrite a program to test your implementation by reading data related to 50 Car objects and store them in an array ARR of Car. Your program should include:a. A method Search() that takes as parameters an array of Car objects ARR and a Car object C. This method should return true if C is inside ARR, false otherwise. b. A method findCylinders that takes as parameters an array of Car objects ARR and number of cylinder engine N. It should display all the cars that have N cylinder engine.arrow_forwardI need help with Chapter 10. Practice exercise 10.9 of the Introduction to Java 11 edition book. I tried using the textbook example but I got a few errors I couldn't figure out how to fix.arrow_forward
- In Java, please. Complete the Course class by implementing the findStudentHighestGpa() method, which returns the Student object with the highest GPA in the course. Assume that no two students have the same highest GPA. Given classes: Class LabProgram contains the main method for testing the program. Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. (Hint: getGPA() returns a student's GPA.) Note: For testing purposes, different student values will be used. Ex. For the following students: Henry Nguyen 3.5 Brenda Stern 2.0 Lynda Robison 3.2 Sonya King 3.9 the output is: Top student: Sonya King (GPA: 3.9) LabProgram.java import java.util.Scanner; public class LabProgram { public static void main(String args[]) { Scanner scnr = new Scanner(System.in); Course course = new Course(); int…arrow_forwardUse Java program to add a method printReceipt to the CashRegister class. The method should print the prices of all purchased items and the total amount due. Hint: You will need to form a string of all prices. Use the concat method of the String class to add additional items to that string. To turn a price into a string, use the call String.valueOf(price). Also, the payment information will need to be the same as the price information. Here is the CashRegister class: /** A cash register totals up sales and computes change due.*/public class CashRegister{ private double purchase; private double payment; /** Constructs a cash register with no money in it. */ public CashRegister() { purchase = 0; payment = 0; } /** Records the sale of an item. @param amount the price of the item */ public void recordPurchase(double amount) { purchase = purchase + amount; } /** Processes a payment received from the customer. @param…arrow_forwardQuestion is in attatchment. Thanks!arrow_forward
- Submit the code (copy&paste) with a screen shot of the same sample run as below. 1. Write a class ArrayMethods, where the main method asks the user to enter the num ber of inputs and stores them in an array called myList. Create a method printArray that prints the array myList. Create another method copyArray that copies the array using System.arraycopy, and multiplies each element in the copied array by 2, then finally returns the array to the main method. Invoke the printarray again to print the array returned by copyArray. In summary, see below. main method does: asks user for inputs and stores in array. Invoke printArray. Invoke copyArray. Invoke printArray again. printArray: void method, parameters passed is an array, prints an array copyArray: array-returningmethod, parameters passedis an array, copies array, multiplies 2 to each element in the copied array, returnsthe copied arrayarrow_forwardI am trying to write a method name findNormalizedHour. I want it to implement the following specification: /** * Given an hour between 0 and 23 (from 24-hour format), * convert it to an hour appropriate for 12-hour format * * @precondition 0 <= hour <= 23 * @postcondition none * * @param hour the hour, in 24-hour format * @return an hour between 1 and 12, as appropriate */The findNormalizedHour() is a method that converts from a 24-hour format to a 12-hour format. Is there anyway I could get some assistance accomplishing this?arrow_forwardplease give me code and output with following direction. thanks!arrow_forward
- 19 We have been dealing with colors as tuples or lists. We could also create a Color class. This would allow us to create new color objects and pass them around. For each method below, write and implementation that matches the content of the method docstring. Recall that individual color channels must have a value between 0 and 255. class Color: """ This class provides a representation of a color """ definit_(self, red, green, blue): Initialize the color with red, green, and blue values 663939arrow_forwardThank you! base on these methods, I want to write one more method Spec for method 5: A method to print a text-based histogram takes one parameter, an array of integers no return value image 2 is the example of expected output. Hint: basically it means base on the file, if number 20 appears once in the file, then I will print one star(*) after the number 20. I think we can get these information from method 4arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
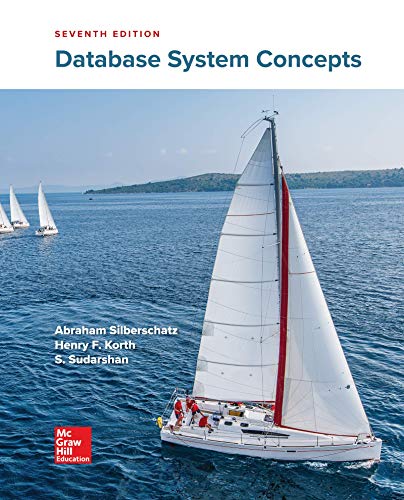
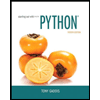
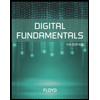
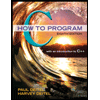
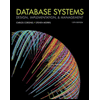
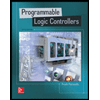