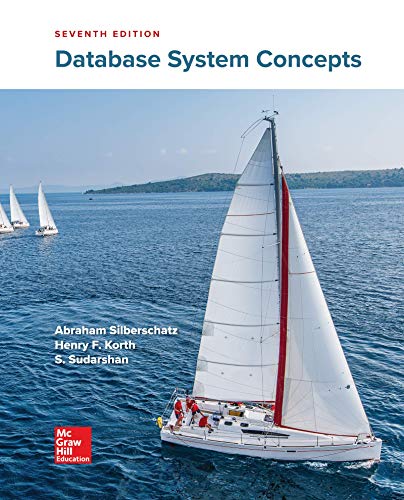
What is a nested function?
and what part in the following code is considered a nest function??
int main( )
{
Begin:arraysize= readNum()
Ok=verifySize(arraySize)
if (ok==1)
creatArray(array-size)
else
Go to Begin
printArray(arraySize)
reverseArray(arraySize)
printArray (arraySize)
}
// ------------------------------------------------------------
int readNum()
{
input an integer
return the integer to main
}
// ------------------------------------------------------------
int versifySize( int arraySize)
{
if (0<arraySize< 20)
return 1
else
return 0
}
// ------------------------------------------------------------
void createArray(int arraySize)
{
counter = 0
making_array: arrayEntry = readNum()
ok = checkNumPositive(arrayEntry)
if (ok == 1)
validNum = divisibleBy3(arrayEntry)
if (validNum==1)
insert arrayEntry into the array
counter = counter + 1
else
go to making_array
else
go to making_array
if (counter < arraySize)
go to making_array
}
// ------------------------------------------------------------
void reverseArray(int arraySize)
{
head = first index of the array
tail = last index of the array
swap: if (head <=tail)
{
swap the content of head and tail (array[head] and array[tail] with each other
increment the head value by one word
decrement tail by one word
go to swap
}
}
// ------------------------------------------------------------
void printArray( int arraySize)
{
..
..
}
// ------------------------------------------------------------
int divisibleBy3(int arryEntry)
{
…
…
}
// ------------------------------------------------------------
int checkNumPositive(int arrayEntry)
{
…
…
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- use c code to develop the condition and function for the following items and then redevelop the code by adding the function as shown below, the original code has been given below. Hint: a)the function need to be made and develop and than add to the code 1. print Reverse 2. void reverseIt 3. void search Array 4.void search array 5. void array copy and void arrayDiff) b) the answer must be include and output and c code void printReverse(int array[], size); //prints the array reverselyvoid reverseIt(int array[], size); //reverses an arrayvoid searchArray(int array[], int size, int num);// returns the first index of the array whose value is num.//returns -1 if the number was not found in the array// optional for lab 6:void searchnArray(int array[], int size, int num, int n);// returns the nth index of the array whose value is num.//returns -1 if the number was not found in the arrayvoid arrayCopy (int arDest[], int arSource[], int commonSize);// copies cells from arSourc to arDestvoid…arrow_forwardMatrix Multiplication• Write a multiplication function that accepts two 2D numpy arrays,and returns the product of the two. This function should test that thearrays are compatible (recall that the number of columns in the firstmatrix must equal the number of rows in the second matrix). Thisfunction should test the array sizes before attempting themultiplication. If the arrays are not compatible, the function shouldreturn -1. solve in pythonarrow_forward//Required Functionvoid func(int array_1[],int array_2[],int size){ for(int i=0;i<size;i++){ if(array_2[i]>array_1[i]){ array_1[i]=array_2[i]; } } return;} //main function to test the function.int main(){ int size=6; int a[]={1,5,9,8,7,6}; int b[]={1,4,10,12,7,15}; printf("First array before function call: "); for(int i=0;i<size;i++){ printf("%d ",a[i]); } func(a,b,size); //call to the created function printf("\nFirst array after function call: "); for(int i=0;i<size;i++){ printf("%d ",a[i]); } return 0;} 1. Write the statements to do the following: write a prototype for your function in the previous problem write a main function which includes the following steps declare two arrays of ints with 25 elements each prompt the user and read values into both arrays call your function from the previous problem print both arraysarrow_forward
- Assume the following main module is in a program that includes the binarysearch function that was shown in a chapter. Why doesn't the pseudocode in the main module work?arrow_forwardThis program will display an array being passed to a function, show. The show function will display the elements of the given array. The main program controls the size and the inputs of an array. // function prototype void show(int [], int); int main(){ const int arraysize=5; int numbers[arraysize]={3,6,12,7,5}; show(numbers,arraysize); // (A) show(numbers,arraysize+1); return 0;} //function body void show(int nums[], int size){ for (int index=0;index<size; index++) cout<<nums[index]<<endl; } What is the output of this program? What is the output if modification (A) has been made?arrow_forwardTwo dimension array in C:Create a two dimension array of integers that is 5 rows x 10 columns.Populate each element in the first 2 rows (using for loops) with the value 5.Populate each element of the last three rows (using for loops) with the value 7.Write code (using for loops) to sum all the elements of the first three columns and output thesum to the screen. without using #definearrow_forward
- 1- Write a user-defined function that accepts an array of integers. The function should generate the percentage each value in the array is of the total of all array values. Store the % value in another array. That array should also be declared as a formal parameter of the function. 2- In the main function, create a prompt that asks the user for inputs to the array. Ask the user to enter up to 20 values, and type -1 when done. (-1 is the sentinel value). It marks the end of the array. A user can put in any number of variables up to 20 (20 is the size of the array, it could be partially filled). 3- Display a table like the following example, showing each data value and what percentage each value is of the total of all array values. Do this by invoking the function in part 1.arrow_forwardProgram in C++arrow_forward/* (name header) #include // Function declaration int main() { int al[] int a2[] = { 7, 2, 10, 9 }; int a3[] = { 2, 10, 7, 2 }; int a4[] = { 2, 10 }; int a5[] = { 10, 2 }; int a6[] = { 2, 3 }; int a7[] = { 2, 2 }; int a8[] = { 2 }; int a9[] % { 5, 1, б, 1, 9, 9 }; int al0[] = { 7, 6, 8, 5 }; int all[] = { 7, 7, 6, 8, 5, 5, 6 }; int al2[] = { 10, 0 }; { 10, 3, 5, 6 }; %3D std::cout « ((difference (al, 4) « ((difference(a2, 4) <« ((difference (a3, 4) « ((difference(a4, 2) <« ((difference (a5, 2) <« ((difference (a6, 2) <« ((difference (a7, 2) <« ((difference(a8, 1) « ((difference (a9, 6) « ((difference(a10, 4) " <« ((difference(al1, 7) « ((difference (al2, 2) == 10) ? "OK\n" : "X\n"); << "al: << "a2: %3D 7)? "OK" : "X") << std::endl == 8) ? "OK\n" : "X\n") 8) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 1) ? "OK\n" : "X\n") == 0) ? "OK\n" : "X\n") 0) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 3) ? "OK\n" : "X\n") == 3) ? "OK\n" : "X\n") == << "a3: == << "a4: <<…arrow_forward
- (_9.). Given an array of integers, write a PHP function to find the maximum element in the array. PHP Function Signature: phpCopy code function findMaxElement($arr) { // Your code here } Example: Input: [10, 4, 56, 32, 7] Output: 56 You can now implement the findMaxElement function to solve this problem. Expect.arrow_forwardWhat does the phrase "base address of an array" relate to, and how does it come to be utilized in a call to a function?arrow_forwardQ_8. Decription:- Given an array of integers, write a PHP function to find the maximum element in the array. PHP Function Signature: phpCopy code function findMaxElement($arr) { // Your code here } Example: Input: [10, 4, 56, 32, 7] Output: 56 You can now implement the findMaxElement function to solve this problems. .arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
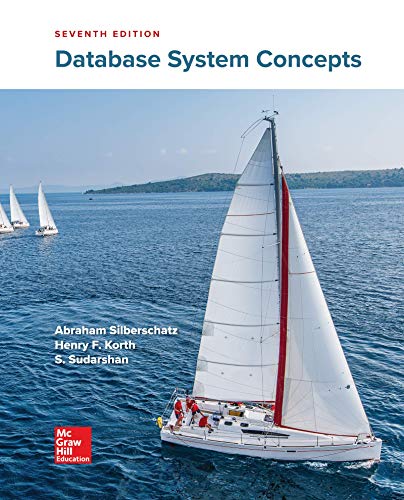
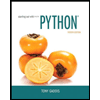
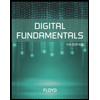
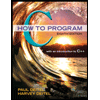
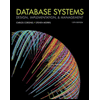
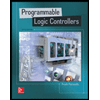