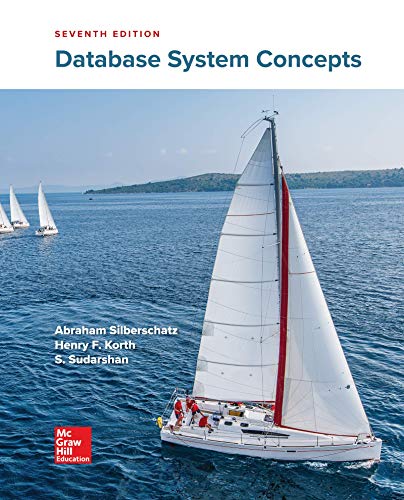
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
![### Understanding the Output of a C++ Program
#### Problem Statement
**Question:** What is the output of the following C++ code if the input is `"1 2 3"`?
```cpp
#include <iostream>
using namespace std;
int main(){
int num1, num2, num3;
double average;
cin >> num1 >> num2 >> num3;
average = (num1 + num2 + num3) / 3.0;
cout << average << endl;
cin.ignore();
return 0;
}
```
#### Explanation
1. **Include Directive**:
- `#include <iostream>` is used to include the standard input-output stream library, which allows the use of `cin` and `cout`.
2. **Namespace**:
- `using namespace std;` allows you to use all elements in the `std` namespace without prefixing them with `std::`.
3. **Variable Declaration**:
- `int num1, num2, num3;` declares three integer variables.
- `double average;` declares a double variable to store the average.
4. **Input Operations**:
- `cin >> num1 >> num2 >> num3;` takes three integer inputs from the user and assigns them to `num1`, `num2`, and `num3`.
5. **Calculation**:
- `average = (num1 + num2 + num3) / 3.0;` calculates the average of the three numbers. The division is done using a floating-point number (`3.0`) to ensure the result is a double.
6. **Output**:
- `cout << average << endl;` outputs the computed average followed by a newline.
7. **Program Termination**:
- `cin.ignore();` is used to ignore any additional input or newline characters.
- `return 0;` signifies the successful completion of the program.
#### Sample Output
For the input `"1 2 3"`, the program will compute the average as:
\[ \text{average} = \frac{1 + 2 + 3}{3.0} = 2.0 \]
**Output:** `2` (formatted as a floating-point number, but displayed without decimal places since the `.0` is not shown by default with standard output settings)](https://content.bartleby.com/qna-images/question/5e343f85-ac09-4dac-ae1f-fa90b444949b/af8414e1-337a-4c55-ab95-dc2fbae4d97c/yy1zyz4_thumbnail.png)
Transcribed Image Text:### Understanding the Output of a C++ Program
#### Problem Statement
**Question:** What is the output of the following C++ code if the input is `"1 2 3"`?
```cpp
#include <iostream>
using namespace std;
int main(){
int num1, num2, num3;
double average;
cin >> num1 >> num2 >> num3;
average = (num1 + num2 + num3) / 3.0;
cout << average << endl;
cin.ignore();
return 0;
}
```
#### Explanation
1. **Include Directive**:
- `#include <iostream>` is used to include the standard input-output stream library, which allows the use of `cin` and `cout`.
2. **Namespace**:
- `using namespace std;` allows you to use all elements in the `std` namespace without prefixing them with `std::`.
3. **Variable Declaration**:
- `int num1, num2, num3;` declares three integer variables.
- `double average;` declares a double variable to store the average.
4. **Input Operations**:
- `cin >> num1 >> num2 >> num3;` takes three integer inputs from the user and assigns them to `num1`, `num2`, and `num3`.
5. **Calculation**:
- `average = (num1 + num2 + num3) / 3.0;` calculates the average of the three numbers. The division is done using a floating-point number (`3.0`) to ensure the result is a double.
6. **Output**:
- `cout << average << endl;` outputs the computed average followed by a newline.
7. **Program Termination**:
- `cin.ignore();` is used to ignore any additional input or newline characters.
- `return 0;` signifies the successful completion of the program.
#### Sample Output
For the input `"1 2 3"`, the program will compute the average as:
\[ \text{average} = \frac{1 + 2 + 3}{3.0} = 2.0 \]
**Output:** `2` (formatted as a floating-point number, but displayed without decimal places since the `.0` is not shown by default with standard output settings)
Expert Solution

arrow_forward
Step 1
The output of the code will be 2.
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

Knowledge Booster
Similar questions
- Find error in the code below. 12/10 should come as 1.2 but it's showing 1.arrow_forwardCreate a flowchart for the C++ code below #include <iostream> using namespace std; int main () { int first_opt, area_opt, perimeter_opt, length, width, radius, side, height, base, a, b, c, A_Rect, A_Square, P_Rect, P_Square, P_Triangle; float A_Circle, A_Triangle, P_Circle; cout<<"Welcome!. Choose what you want to solve"<<"\n"; cout<<"1. AREA"<<"\n"; cout<<"2. PERIMETER"<<"\n"; cin>>first_opt; cout<<"Choose from the following"<<"\n"; switch(first_opt) { case 1: cout<<"1. Area of the Rectangle"<<"\n"; cout<<"2. Area of the Circle"<<"\n"; cout<<"3. Area of the Square"<<"\n"; cout<<"4. Area of the Triangle"<<"\n"; cin>>area_opt; switch(area_opt) { case 1: cout<<"Enter length: "<<"\n"; cin>>length; cout<<"Enter width: "<<"\n"; cin>>width; A_Rect = length*width; cout<<"The area of the rectangle is:…arrow_forwardIn C++Find the output = for( int a = 5; a >0; a -- ) { cout << "value of a =" << a << endl; } *arrow_forward
- ê #include using namespace std; int main() { int count = 5; int num = 2; while (count r && num ){ cout << count << " " << num << endl; count -=1; if (count % 2 == 0) num -=1; } return 0; Output: 52 4 1 3 1 20 10arrow_forwardPlease correct the program in c++arrow_forwardC++ Need Help with 3 part #include <iostream>#include <string>using namespace std; void PrintMenue() {cout << "\nMENU" << endl;cout << "c - Number of non-whitespace characters" << endl;cout << "w - Number of words" << endl;cout << "f - Find text" << endl;cout << "r - Replace all !'s" << endl;cout << "s - Shorten spaces" << endl;cout << "q - Quit" << endl;cout << "\nChoose an option:" << endl;} /* Define your functions here. */ int main() { string text;cout << "Enter a sample text:\n" << endl;getline(cin, text);cout << "You entered: ";cout << text << endl;PrintMenue();return 0;}arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
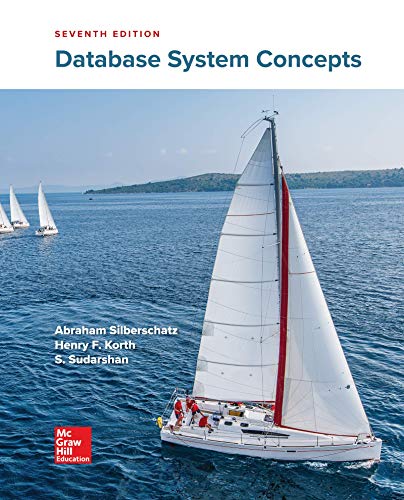
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
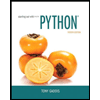
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
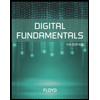
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
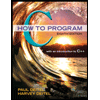
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
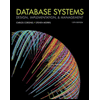
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
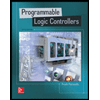
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education