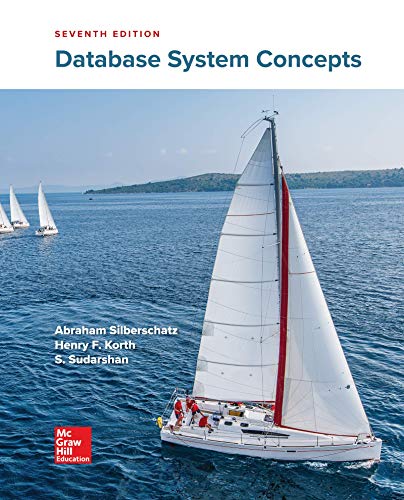
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Write the full C++ code for the expected output
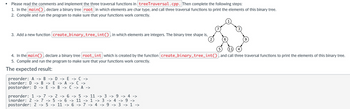
Transcribed Image Text:Please read the comments and implement the three traversal functions in treeTraversal.cpp. Then complete the following steps:
1. In the main(), declare a binary tree root in which elements are char type, and call three traversal functions to print the elements of this binary tree.
2. Compile and run the program to make sure that your functions work correctly.
3
3. Add a new function create_binary_tree_int(), in which elements are integers. The binary tree shape is,
2
5
(11) (4)
4. In the main, declare a binary tree root_int which is created by the function create_binary_tree_int(), and call three traversal functions to print the elements of this binary tree.
5. Compile and run the program to make sure that your functions work correctly.
The expected result:
preorder: A
B
D - E -> C >
inorder: D -> B -> E -> A -> C ->
postorder: D -> E > B > C -> A ->
preorder: 1 -> 7 -> 2 -> 6
inorder: 2 -> 75 -
postorder: 2 -> 5 -> 11
-> 5
<-
6 -> 11
-> 6 ->
-> 11 -> 3
-> 1 - -> 3
-> 9 -> 4 ->
-> 4 -> 9 ->
7 -> 4 -> 9 -> 3 ->
-> 1 ->
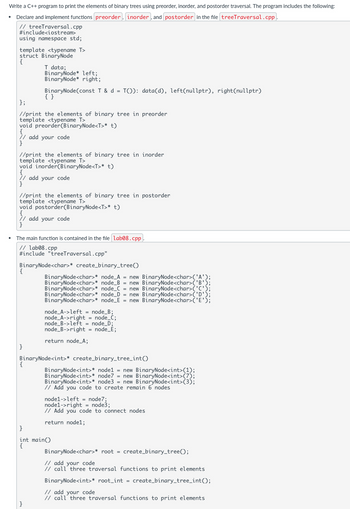
Transcribed Image Text:Write a C++ program to print the elements of binary trees using preorder, inorder, and postorder traversal. The program includes the following:
Declare and implement functions preorder, inorder, and postorder in the file treeTraversal.cpp.
// treeTraversal.cpp
#include<iostream>
using namespace std;
template<typename T>
struct BinaryNode
{
T data;
BinaryNode✶ left;
BinaryNode* right;
BinaryNode(const T & d = T()): data(d), left(nullptr), right(nullptr)
{ }
};
//print the elements of binary tree in preorder
template<typename T>
void preorder(BinaryNode<T>* t)
{
// add your code
}
//print the elements of binary tree in inorder
template<typename T>
void inorder(BinaryNode<T>* t)
{
// add your code
}
//print the elements of binary tree in postorder
template<typename T>
void postorder (BinaryNode<T>* t)
{
// add your code
}
The main function is contained in the file Lab08.cpp
// lab08.cpp
#include "treeTraversal.cpp"
BinaryNode<char>* create_binary_tree()
{
}
BinaryNode<char>* node_A = new BinaryNode<char>('A');
BinaryNode<char>* node_B = new BinaryNode<char>('B');
BinaryNode<char>* node_C = new BinaryNode<char>('C');
BinaryNode<char>* node_D = new BinaryNode<char>('D');
BinaryNode<char>* node_E = new BinaryNode<char>('E');
node_A->left = node_B;
node_A->right = node_C;
node B->left = node_D;
node_B->right = node_Ė;
return node_A;
BinaryNode<int>* create_binary_tree_int()
{
}
BinaryNode<int>* node1 = new BinaryNode<int>(1);
BinaryNode<int>* node = new BinaryNode<int>(7);
BinaryNode<int>* node3 = new BinaryNode<int>(3);
// Add you code to create remain 6 nodes
node1->left = node7;
node1->right = node3;
// Add you code to connect nodes
return node1;
int main()
{
}
BinaryNode<char>* root = create_binary_tree();
// add your code
// call three traversal functions to print elements
BinaryNode<int>* root_int = create_binary_tree_int();
// add your code
// call three traversal functions to print elements
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- Question. Explain why the following code does not conform to the C++ standard. (There's a possiblity this does compile on your machine due to some non-standard settings.) #include int main() { int length; std::cin >> length; double data[length]; return 0; Your answer may include a compiler error, but that alone is not a sufficient answer. Your explanation should consist of a few sentences, and must use the vocabulary we have de- veloped during the course regarding memory allocation. You probably want to ponder array-problems.cpp and the lecture notes from Week 7.arrow_forwardI am currently coding in C++, using Dev C++ application. I keep getting the error " 'null' was not declared in this scope." The line of code that it is refrencing is " std::srand(std::time(null));". I have no idea what is happening or how to fix it.arrow_forwardWhich of the following are advantages of inline function declarations in C++ or the "Pragma Priority (x)" in Ada? they do not save push/pop variable overhead on the stack when it is called there is a lookup time required at runtime which is the same as an external function call during compile tim. Please type answer no write by hend.arrow_forward
- this shows me i need the C++ code Your answer could not be processed because it contains errors: line 3: a function-definition is not allowed here before '{' tokenarrow_forward1. Write a c++ code that takes two integer numbers as inputs, then adds them using a function called add(x,y), then subtracts them using a function called subtract(x,y), and outputs the results in an understandable way.arrow_forwardPlease use easy logic with proper indentations and comments for understanding!. Coding should be in C++. I am providing an algorithm that you will need to answer this coding question. I am also providing this solved program but it's not printing each line separately as it supposed to (Output is given in the picture). The file has 3 separate sentences on 3 lines. Our program supposed to print three separate lines as exactly shown in the sample output. Please review my code and provide a correction to it. Thank you 1 2 3 #include <iostream> 4 #include <fstream>5 #include <cstdlib>6 7 using namespace std; 8 9 void status(string st,int &vowel,int &consonant, int &other)10 {11 12 //if it is vowel13 if ((st[0]=='a' || st[0] == 'e' || st[0] == 'i' || st[0] == 'o' || st[0]=='u') || (st[0]=='A' || st[0] == 'E' || st[0] == 'I' || st[0] == 'O' || st[0]=='U') )14 vowel++; //incrementing vowels by 115 else if(isalpha(st[0]))16 consonant++;…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
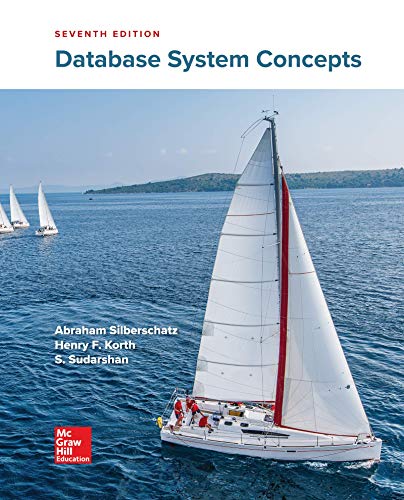
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
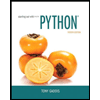
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
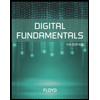
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
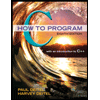
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
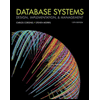
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
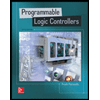
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education