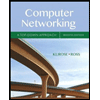
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Write a c++ program.
You will create a class “Node” with the following private data attributes:
- line – line from a file (string)
- next - (pointer to a Node)
Put your class definition in a header file and the implementation of the methods in a .cpp file.
Follow the style they use in the book of having a "#include" for the implementation file at the bottom of the header file.
You will have the following public methods:
- Accessors and mutators for each attribute
- Constructor that initializes the attributes to nulls (empty string and nullptr)
LinkedList class
You will create a class “LinkedList” with the following private data attributes:
- headPtr – raw pointer to the head of the list
- numItems – number of items in the list
Put your class definition in a header file and the implementation of the methods in a .cpp file.
Follow the style they use in the book of having a "#include" for the implementation file at the bottom of the header file.
You will have the following public methods:
- Accessor to get the current size (numItems)
- Constructor that initializes numItems to zero and headPtr to nullptr
- Add a node – this method will take as input one string value. It will then create a node object and set the attribute. Then it will put it in the linked list at the correct position – ascending order – avoiding duplicates. You will do all of the work while building the linked list.
- toVector – returns
vector with the contents of the list. You will use only the “push_back” method to get the strings into the vector.
Client program
Your program will ask the user for the file name, open the text file , read each line and invoke the addNode method. Make sure the file opened correctly before you start processing it. You do not know how many lines are in the file so your program should read until it gets to the end of the file.
It will display the contents of the list (the lines that were read from the file in sorted order with no duplicates) using the LinkedList class method “toVector” that puts all of the lines into a vector and returns it to the program that will display it.
Your client program will do the following:
- Read file name from user
- Display the number of lines that were read out of the file and were passed to the addNode method.
- Display the lines in your linked list using the class method toVector to get the lines.
Display the number of lines in the vector.
I have attached three files you can use to test your program. Blackboard handles files in a strange way sometimes. You may not be able to directly download the files, but you can open them, copy and paste the contents into a notepad file and save that file.
You will write all of the code for processing the linked list - do not use any predefined objects in C++. You do not need to offer a user interface – simply display the number of lines read from the file, the list using the “toVector” method and the number of lines in the vector.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- In C++ Write a class, named "TwoOrLess" with a header file (two_or_less.hpp) and an implementation file (two_or_less.cpp). This is a class that acts much like a set, except it can hold 0, 1, or 2 duplicates of an int. You need to support the insert, count, and size methods with the same parameters and return types as the set<int> class (see test cases).arrow_forwardWritten in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwardUsing C++ Without Using linked lists: Create a class AccessPoint with the following: x - a double representing the x coordinate y - a double representing the y coordinate range - an integer representing the coverage radius status - On or Off Add constructors. The default constructor should create an access point object at position (0.0, 0.0), coverage radius 0, and Off. Add accessor and mutator functions: getX, getY, getRange, getStatus, setX, setY, setRange and setStatus. Add a set function that sets the location coordinates and the range. Add the following member functions: move and coverageArea. Add a function overLap that checks if two access points overlap their coverage and returns true if they do. Add a function signalStrength that returns the wireless signal strength as a percentage. The signal strength decreases as one moves away from the access point location. Represent this with bars like, IIIII. Each bar can represent 20% Test your class by writing a main function that…arrow_forward
- Assignment-3 With the help of a C program show how to typecast a void * to an int * . Attach the code and output and upload the file on classroom.arrow_forwardWritten in Python with docstring please if applicable Thank youarrow_forwardWrite Java code Objectives •Use an abstract data type for a list •Use a method that contains an Object type as a formal parameter •Use methods in an existing class •Access data members within a class Required Files: •ListInterface.java •ListException.java •ListOutOfBoundsException.java •ListArrayBased.java Description A list is an abstract data type used to store ordered data, and contains operations that fall into the following categories: (1) add data to a data collection, (2) remove data from a data collection, and (3) ask questions about data in a data collection. The operations which ask questions about the data in a data collection may include operations that determine if a data collection is empty, determine the size of a data collection, and retrieve the item at a given position in a data collection. A list should be able to contain any type of object. The methods for the List support any object as specified in the interface. In this assignment, the objects will be S trings.…arrow_forward
- Write a program to test the Book class using C++. Create objects and use the print function in the objects to display information.arrow_forwardWrite a C++ program, you will create a class called Books to hold the information about the books in a library. The information required for each book is as follows Book Title Author name Publisher Publish year Subject Book ID (identical 5 digits’ number) Implement the following getBookInfo () member function that receives a pointer to a book and fills it up with user data. User input validation is necessary. The user should be properly prompted for each field. void getBookInfo (Books *b) Implement the following printBookInfo () member function. void printBookInfo (Books *b) Your function should print the book in the following format: Book Title : Object Oriented Programming Using C++ Author : Chris M. Szalwinski Publisher : Amazon Year : 2016 Subject : Computer Science Book Id : 00034 Note: You need to add required constructors/destructors, member functions or data members/variables in your program to complete its execution. //Missing lines of codes to be completed by the student }…arrow_forwardIn C++ Create a new project named lab8_1. You will be implementing two classes: A Book class, and a Bookshelf class. The Bookshelf has a Book object (actually 3 of them). You will be able to choose what Book to place in each Bookshelf. Here are their UML diagrams Book class UML Book - author : string- title : string- id : int- count : static int + Book()+ Book(string, string)+ setAuthor(string) : void+ setTitle(string) : void+ print() : void+ setID() : void And the Bookshelf class UML Bookshelf - book1 : Book- book2 : Book- book3 : Book + Bookshelf()+ Bookshelf(Book, Book, Book)+ setBook1(Book) : void+ setBook2(Book) : void+ setBook3(Book) : void+ print() : void Some additional information: The Book class also has two constructors, which again offer the option of declaring a Book object with an author and title, or using the default constructor to set the author and title later, via the setters . The Book class will have a static member variable…arrow_forward
- Please answer in C++ and give explanation LAB 17.1-B Linked List Read the internal documentation for class ItemList carefully. Fill in the missing code in the implementation file, being careful to adhere to the preconditions and postconditions. Compile your program. ------------------------------------------------------------------------------------------------------------------------------- class ItemList { private: struct ListNode { int value; ListNode * next; }; ListNode * head; public: ItemList(); // Post: List is the empty list. bool IsThere(int item) const; // Post: If item is in the list IsThere is // True; False, otherwise. void Insert(int item); // Pre: item is not already in the list. // Post: item is in the list. void Delete(int item); // Pre: item is in the list. // Post: item is no…arrow_forwardin c++ codearrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
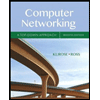
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
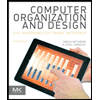
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
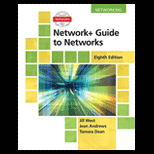
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
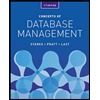
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
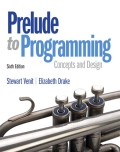
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
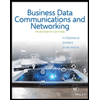
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY