Write a class called String Classifier that displays information about the keystrokes in a string. The class should have the following members: StringClassifier Class: Attributes: -string:String -int:keyStrokes -int:letters -int:digits -int:uppers -int:lowers -int:odds -int:evens Methods: +StringClassifier(String) +getString():String +setString(String):void +classify():void +toString():String The string attribute will contain a string value. It can be anything - numbers, letters, or special characters. fa In the mutator method, the string attribute is only set if there are characters in the programmer-supplied string. The letters and digits attributes will store the number of letters and digits in the string. The uppers and lowers attributes will each store the number of upper-case and lowercase letters in the string. The evens and odds attributes will each store the number of even digits and odd digits in the string. None of these data members require accessor/mutator methods, since they will be given their values in the classify() method, and their values can be viewed in the toString() method. The classify() method will examine the string attribute and store the following information in the other instance variables: • The number of keystrokes in the string. The number of letters in the string. The number of upper-case and lower-case letters in the string. The number of digits in the string. The number of even and odd digits in the string. The toString() method will return a string that shows the information about your string (see sample code and screen shots below for format). The following program can be used to test your class: import javax.swing.JOptionPane; public class TestClassifier ( public static void main(String[] args) ( String str= JOption Pane.showInput Dialog(null, "Enter a sentence to classify.", "Sentence Input" JOption Pane.QUESTION_MESSAGE); String Classifier classifier = new String Classifier(str); JOptionPane.showMessageDialog(null, classifier.toString(), "String Information", JOptionPane.INFORMATION_MESSAGE); } } A sample run of this program would produce the following dialog A sample run of this program would produce the following dialogs: Sentence Input X ? Enter a sentence to classify. where the input is giong to be populated OK Cancel (java programming)
Write a class called String Classifier that displays information about the keystrokes in a string. The class should have the following members: StringClassifier Class: Attributes: -string:String -int:keyStrokes -int:letters -int:digits -int:uppers -int:lowers -int:odds -int:evens Methods: +StringClassifier(String) +getString():String +setString(String):void +classify():void +toString():String The string attribute will contain a string value. It can be anything - numbers, letters, or special characters. fa In the mutator method, the string attribute is only set if there are characters in the programmer-supplied string. The letters and digits attributes will store the number of letters and digits in the string. The uppers and lowers attributes will each store the number of upper-case and lowercase letters in the string. The evens and odds attributes will each store the number of even digits and odd digits in the string. None of these data members require accessor/mutator methods, since they will be given their values in the classify() method, and their values can be viewed in the toString() method. The classify() method will examine the string attribute and store the following information in the other instance variables: • The number of keystrokes in the string. The number of letters in the string. The number of upper-case and lower-case letters in the string. The number of digits in the string. The number of even and odd digits in the string. The toString() method will return a string that shows the information about your string (see sample code and screen shots below for format). The following program can be used to test your class: import javax.swing.JOptionPane; public class TestClassifier ( public static void main(String[] args) ( String str= JOption Pane.showInput Dialog(null, "Enter a sentence to classify.", "Sentence Input" JOption Pane.QUESTION_MESSAGE); String Classifier classifier = new String Classifier(str); JOptionPane.showMessageDialog(null, classifier.toString(), "String Information", JOptionPane.INFORMATION_MESSAGE); } } A sample run of this program would produce the following dialog A sample run of this program would produce the following dialogs: Sentence Input X ? Enter a sentence to classify. where the input is giong to be populated OK Cancel (java programming)
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
please answer within 30 minutes.
![Write a class called StringClassifier that displays information about the
keystrokes in
a string. The class should have the following members:
Class: StringClassifier
Attributes:
-string:String
-int:keyStrokes
-int:letters
-int:digits
-int:uppers
-int:lowers
-int:odds
-int:evens
Methods:
+StringClassifier(String)
+getString(): String
+setString(String):void
+classify():void
+toString():String
The string attribute will contain a string value. It can be anything - numbers,
letters, or
special characters. fa In the mutator method, the string attribute is only set if
there are
characters in the programmer-supplied string.
The letters and digits attributes will store the number of letters and digits in the
string.
The uppers and lowers attributes will each store the number of upper-case and
lowercase letters in the string. The evens and odds attributes will each store the
number of even digits and odd digits in the string. None of these data members
require accessor/mutator methods, since they will be given their values in the
classify() method, and their values can be viewed in the toString() method.
The classify() method will examine the string attribute and store the following
information in the other instance variables:
• The number of keystrokes in the string.
• The number of letters in the string.
• The number of upper-case and lower-case letters in the string.
• The number of digits in the string.
• The number of even and odd digits in the string.
The toString() method will return a string
that shows the information about your string
(see sample code and screen shots below for format). The following program
can be
used to test your class:
import javax.swing.JOptionPane;
public class TestClassifier
{
public static void main(String[] args)
{
String str = JOption Pane.showInput Dialog(null,
"Enter a sentence to classify.",
"Sentence Input" JOptionPane.QUESTION_MESSAGE);
String Classifier classifier = new String Classifier(str);
JOptionPane.showMessageDialog(null, classifier.toString(),
"String Information",
JOption Pane.INFORMATION_MESSAGE);
}
}
A sample run of this program would produce the following dialog
A sample run of this program would produce the following dialogs:
Sentence Input
X
?
Enter a sentence to classify.
where the input is giong to be populated
OK
Cancel
(java programming)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa7b3ae88-5fa8-4d37-9c2b-c3989c43976d%2F76adc4d3-fa97-4f0d-895d-b8ef17e0dc4e%2Fl0vng9r_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Write a class called StringClassifier that displays information about the
keystrokes in
a string. The class should have the following members:
Class: StringClassifier
Attributes:
-string:String
-int:keyStrokes
-int:letters
-int:digits
-int:uppers
-int:lowers
-int:odds
-int:evens
Methods:
+StringClassifier(String)
+getString(): String
+setString(String):void
+classify():void
+toString():String
The string attribute will contain a string value. It can be anything - numbers,
letters, or
special characters. fa In the mutator method, the string attribute is only set if
there are
characters in the programmer-supplied string.
The letters and digits attributes will store the number of letters and digits in the
string.
The uppers and lowers attributes will each store the number of upper-case and
lowercase letters in the string. The evens and odds attributes will each store the
number of even digits and odd digits in the string. None of these data members
require accessor/mutator methods, since they will be given their values in the
classify() method, and their values can be viewed in the toString() method.
The classify() method will examine the string attribute and store the following
information in the other instance variables:
• The number of keystrokes in the string.
• The number of letters in the string.
• The number of upper-case and lower-case letters in the string.
• The number of digits in the string.
• The number of even and odd digits in the string.
The toString() method will return a string
that shows the information about your string
(see sample code and screen shots below for format). The following program
can be
used to test your class:
import javax.swing.JOptionPane;
public class TestClassifier
{
public static void main(String[] args)
{
String str = JOption Pane.showInput Dialog(null,
"Enter a sentence to classify.",
"Sentence Input" JOptionPane.QUESTION_MESSAGE);
String Classifier classifier = new String Classifier(str);
JOptionPane.showMessageDialog(null, classifier.toString(),
"String Information",
JOption Pane.INFORMATION_MESSAGE);
}
}
A sample run of this program would produce the following dialog
A sample run of this program would produce the following dialogs:
Sentence Input
X
?
Enter a sentence to classify.
where the input is giong to be populated
OK
Cancel
(java programming)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
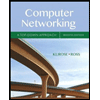
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
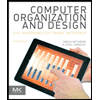
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
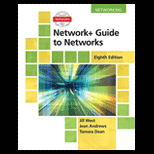
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
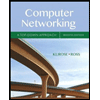
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
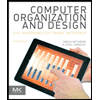
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
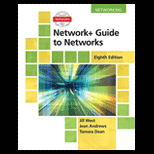
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
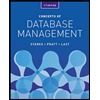
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
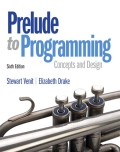
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
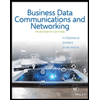
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY