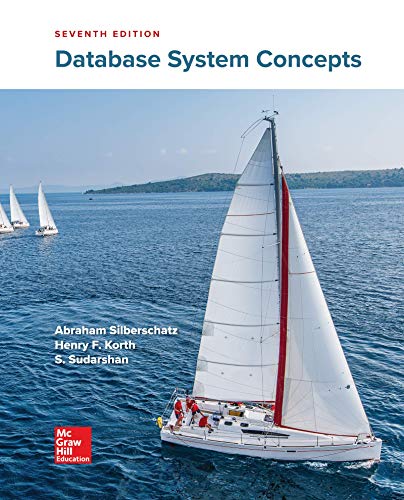
write a computer
Input: Truth values for two statement letters A and B
Output: Corresponding truth values (appropriately
labeled, of course) for
A Λ B, A ∨ B, A → B, A ↔ B, A′

Objective: This program prints the truth table of certain logic gates.
Programming language: since no language is mentioned, C++ is used.
Answer:
#include <iostream>
#include <stdlib.h>
using namespace std;
void printAND(int A[],int B[])
{
int Z;
printf("AND gate\n");
printf("A |\t |B\tZ=A AND B");
for(int i = 0; i <4;i++) {
//computing AND gate values
Z = A[i] & B[i];
printf("\n %d |\t %d|\t%d",A[i],B[i],Z);
}
}
void printOR(int A[],int B[])
{
int Z;
printf("OR gate\n");
printf("A |\t |B\t|Z=A OR B");
for(int i = 0; i <4;i++) {
//computing AND gate values
Z = A[i] | B[i];
printf("\n %d |\t %d|\t%d",A[i],B[i],Z);
}
}
void printNOT(int B[])
{
int Z;
printf("NOT gate\n");
printf("B\t|Z=NOT B");
for(int i = 0; i <2;i++) {
//computing NOT gate values
Z = !B[i];
printf("\n%d|\t%d",B[i],Z);
}
}
void printIMPLY(int A[],int B[])
{
int Z;
printf("IMPLES THAT\n");
printf("A |\t |B\t|Z=A -> B");
for(int i = 0; i <4;i++) {
//computing IMPLIES THAT
if(A[i]==1 && B[i]==0)
Z = 0;
else
Z = 1;
printf("\n %d |\t %d|\t%d",A[i],B[i],Z);
}
}
void printEQUI(int A[],int B[])
{
int Z;
printf("EQUIVALENCE\n");
printf("A |\t |B\t|Z=A <-> B");
for(int i = 0; i <4;i++) {
//computing IMPLIES THAT
if(A[i]==B[i])
Z = 1;
else
Z = 0;
printf("\n %d |\t %d|\t%d",A[i],B[i],Z);
}
}
int main()
{
int A[5] = { 0, 0, 1, 1};
int B[5] = { 0, 1, 0, 1};
int Z;
printAND(A,B);
cout<<"\n";
printOR(A,B);
cout<<"\n";
printNOT(B);
cout<<"\n";
printIMPLY(A,B);
cout<<"\n";
printEQUI(A,B);
}
Step by stepSolved in 3 steps with 1 images

- subject : thoery of compuer sciencearrow_forwardIn MATLAB, primes(N) provides all the prime numbers less than or equal to N. E.g. primes(15) = [2 3 5 7 11 13]. The sum of the first digit of each prime is 19 (i.e. 2+3+5+7+1+1 = 19). What is the sum of the first digit of all primes less than or equal to 55555? You may want to use the num2str() function. Please use matlabarrow_forwardProvide Python code for the given Lab problem.arrow_forward
- Select the truth assignment that proves that the argument below is not valid: P→r r-q זי P p: T, q: F, r: T O p: T, q: T, r: T p: T, q: T, r: F Op: F. q: T, r: Farrow_forwardcan you show me the code for this in python 3 , please provide explanationsarrow_forwardwrite a computer program that produces the desired output from the given input. Input: Truth values for two statement letters A and BOutput: Corresponding truth values for the wffs A → B′ and B′ Λ [A V (A Λ B)]arrow_forward
- In MATLAB, primes(N) provides all the prime numbers less than or equal to N. E.g. primes(15) = [2 3 5 7 11 13]. The sum of the first digit of each prime is 19 (i.e. 2+3+5+7+1+1 = 19). What is the sum of the first digit of all primes less than or equal to 6666? You may want to use the num2str() function.arrow_forwardIf w is TRUE, x is TRUE, and y is FALSE, what is ((w AND x AND y') OR (w' AND x AND y')) AND ((w AND x AND y') AND (w' AND x AND y'))'?arrow_forwardDetermine the truth value of each of these statements if the domain of each variable consists of all real numbers. ∃x∃y(x + y ≠ y + x) ∀x(x ≠ 0 → ∃y(xy = 1)).arrow_forward
- Computer Science- AI- NLP - You are given a training set of 30 numbers that consists of 21 zeros and 1 each of the other digits 1-9. Now we see the following test set: 0 0 0 0 0 3 0 0 0 0. What is the unigram perplexity?arrow_forwardWrite a Python program that implements the Taylor series expansion of the function (1+x) for any x in the interval (-1,1], as given by: 1(1+x) = x-x²/2 + x³/3 - x^/4 + x³/5. The program prompts the user to enter the number of terms n. If n> 0, the program prompts the user to enter the value of x. If the value of xis in the interval (-1, 1], the program calculates the approximation to l(1+x) using the first n terms of the above series. The program prints the approximate value. Note that the program should validate the user input for different values. If an invalid value is entered, the program should output an appropriate error messages and loops as long as the input is not valid. Sample program run: Enter number of terms: 0 Error: Zero or negative number of terms not accepted Enter the number of terms: 9000 Enter the value of x in the interval (-1, 1]: -2 Error: Invalid value for x Enter the value of x in the interval (-1, 1]: 0.5 The approximate value of ln (1+0.5000) up to 9000 terms…arrow_forwardThe truth table shown below is supposed to reflect the function F(x, y, z) = y(x + z') but some e lines are in error. Identify the line numbers of the wrong lines and provide correct values. Y F 1 1 4 1 1 5 1 6 1 1 1 7 1 1 1 8 1 1 1 1 2.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
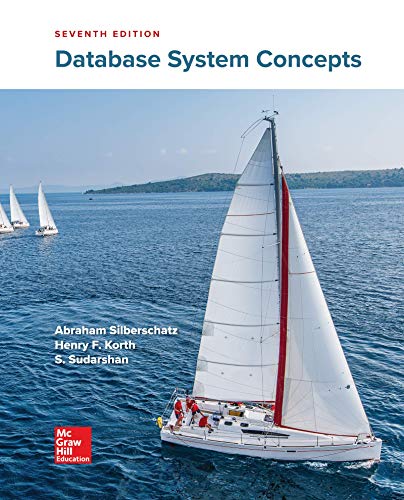
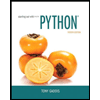
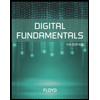
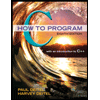
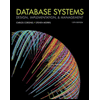
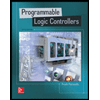