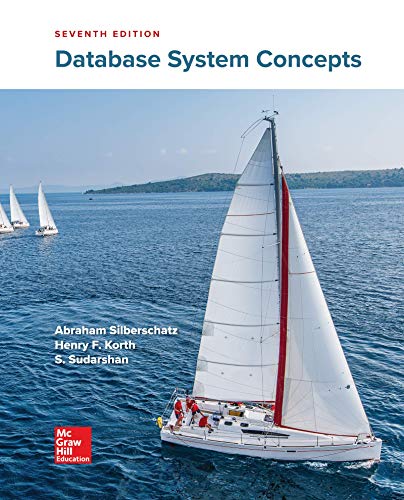
Write a function called ascii_caps() that takes a single list of characters as the input
parameter and returns a new list of integers that contains the ASCII codes of the uppercase
characters in the input list.
Sample run,
>>> print(ascii_caps(['H', 'e', 'l', 'L', 'o', 'W', 'o', 'r', 'l',
'D']))
[72, 76, 87, 68]
Note that the ASCII codes for H', L', 'W', 'D' are 72, 76, 87, and 68, respectively.
Hint: you may want to use ord() and chr() functions in your solutions.
2) Add a function named read_string() that takes a string parameter and prints the
following to the terminal.
• prints if the input string starts with “s” and ends with “e”.
• prints the total number of characters in the string
• prints the total number of words in the string
• prints how many words of the input string contain the letter “a” in it.
• prints all the positions (index values) of the letter ‘a’ in the string, if ‘a’ appears in the
string.

Step by stepSolved in 2 steps with 2 images

- Define a function named get_encrypted_list (word) which takes a word as a parameter. The function returns a list of characters. The first element is the first letter from the parameter word and the rest is as a sequence of '*', each '*' representing a letter in the parameter word. Note: you can assume that the parameter word is not empty. For example: Test Result ['h', '*', **1 guess = get_encrypted_list('hello') print(guess) print (type (guess)) guess = get_encrypted_list('succeed') ['s', '*', print (guess) **¹, ¹*¹] **']arrow_forwardWrite a function called match_words() that takes three input parameters; a list of strings of any length (e.g., ['789', 'del','a', 'do', ‘abc’,'bb','dcc']), a character c and an integer x. This function returns a list of all strings in the 2-D list that starts with the character c and is at most x characters long. Sample run, >>> alist = ['6789', 'black', 'green', 'd', 'blue', 'red', 'beige', 'dddd', 'yellow', 'buff', 'zzzzzzzzz'] >>> print( match_words( alist, ‘b’, 4 )) ['blue’, ‘buff’]arrow_forwardPYTHON Complete the function below, which takes two arguments: data: a list of tweets search_words: a list of search phrases The function should, for each tweet in data, check whether that tweet uses any of the words in the list search_words. If it does, we keep the tweet. If it does not, we ignore the tweet. data = ['ZOOM earnings for Q1 are up 5%', 'Subscriptions at ZOOM have risen to all-time highs, boosting sales', "Got a new Mazda, ZOOM ZOOM Y'ALL!", 'I hate getting up at 8am FOR A STUPID ZOOM MEETING', 'ZOOM execs hint at a decline in earnings following a capital expansion program'] Hint: Consider the example_function below. It takes a list of numbers in numbers and keeps only those that appear in search_numbers. def example_function(numbers, search_numbers): keep = [] for number in numbers: if number in search_numbers(): keep.append(number) return keep def search_words(data, search_words):arrow_forward
- In Python, create a function that takes a string S as a parameter. This function should return a list containing the characters in S that occur the most. Do not use built-in or advanced string/math functions(SUCH AS SORTED()).e.g.yourfxn("hello") returns ['l']yourfxn("acrobatics") returns ['a', 'c']arrow_forwardDefine a function named get_encrypted_list (word) which takes a word as a parameter. The function returns a list of characters. The first element is the first letter from the parameter word and the rest is as a sequence of '*', each '*' representing a letter in the parameter word. Note: you can assume that the parameter word is not empty. For example: Test Result ['h', ¹*¹ **', '*'] *** guess = get_encrypted_list('hello') print (guess) print (type (guess)) guess = get_encrypted_list('succeed') ['s', '*', print (guess) **']arrow_forwardWrite a function that takes in a list of numbers as arguments and returns the productof all the numbers in the list. Use a while loop to multiply each number in the list together.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
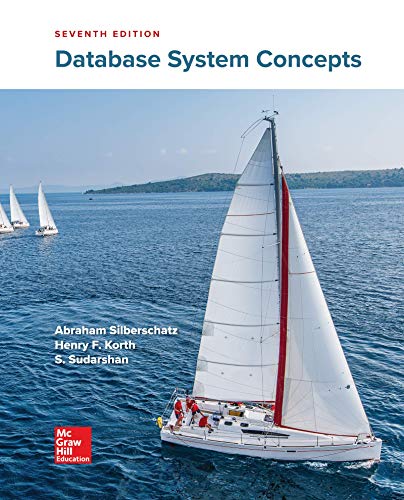
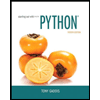
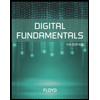
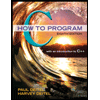
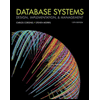
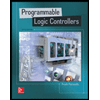