Write a function called remove_punct() that accepts an array of characters and the number of items in the array as parameters, removes the punctuation (',', '!', '.') characters from the array, and returns the number of punctuation characters removed. For example, if the array contains ['C', 'p', 't', 'S', ',', '1', '2', '1', '.', 'i', 's', 'f', 'u', 'n', '!'], then the function should remove the punctuation characters. The function must remove the characters by shifting all characters to the right of each punctuation character, left by one spot in the array. This will overwrite the punctuation characters, resulting in: ['C', 'p', 't', 'S', '1', '2', '1', 'i', 's', 'f', 'u', 'n']. In this case, the function returns 3. Note: if the array does not contain any punctuation characters, then the array is unchanged and the function returns 0.
Write a function called remove_punct() that accepts an array of characters and the number of items in the array as parameters, removes the punctuation (',', '!', '.') characters from the array, and returns the number of punctuation characters removed.
For example, if the array contains ['C', 'p', 't', 'S', ',', '1', '2', '1', '.', 'i', 's', 'f', 'u', 'n', '!'], then the function should remove the punctuation characters. The function must remove the characters by shifting all characters to the right of each punctuation character, left by one spot in the array. This will overwrite the punctuation characters, resulting in: ['C', 'p', 't', 'S', '1', '2', '1', 'i', 's', 'f', 'u', 'n']. In this case, the function returns 3. Note: if the array does not contain any punctuation characters, then the array is unchanged and the function returns 0.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

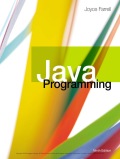
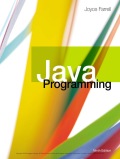