Write a function named pi_multiples() that takes an integer parameter num. This function repeatedly asks the user to enter an integer between 2 and 50. Assume the user will always enter an integer, but if the number must end. So, for any integer x entered within the range, your function must do the following: if x is divisible by num (the parameter), it must multiply x by the value of pi (call it result) and maintain a sum of these results. In every iteration of the loop your function must print x and the result. And when the loop terminates your function should return the sum. outside this range, the loop
Write a function named pi_multiples() that takes an integer parameter num. This function repeatedly asks the user to enter an integer between 2 and 50. Assume the user will always enter an integer, but if the number must end. So, for any integer x entered within the range, your function must do the following: if x is divisible by num (the parameter), it must multiply x by the value of pi (call it result) and maintain a sum of these results. In every iteration of the loop your function must print x and the result. And when the loop terminates your function should return the sum. outside this range, the loop
Chapter8: Arrays
Section: Chapter Questions
Problem 8PE
Related questions
Question
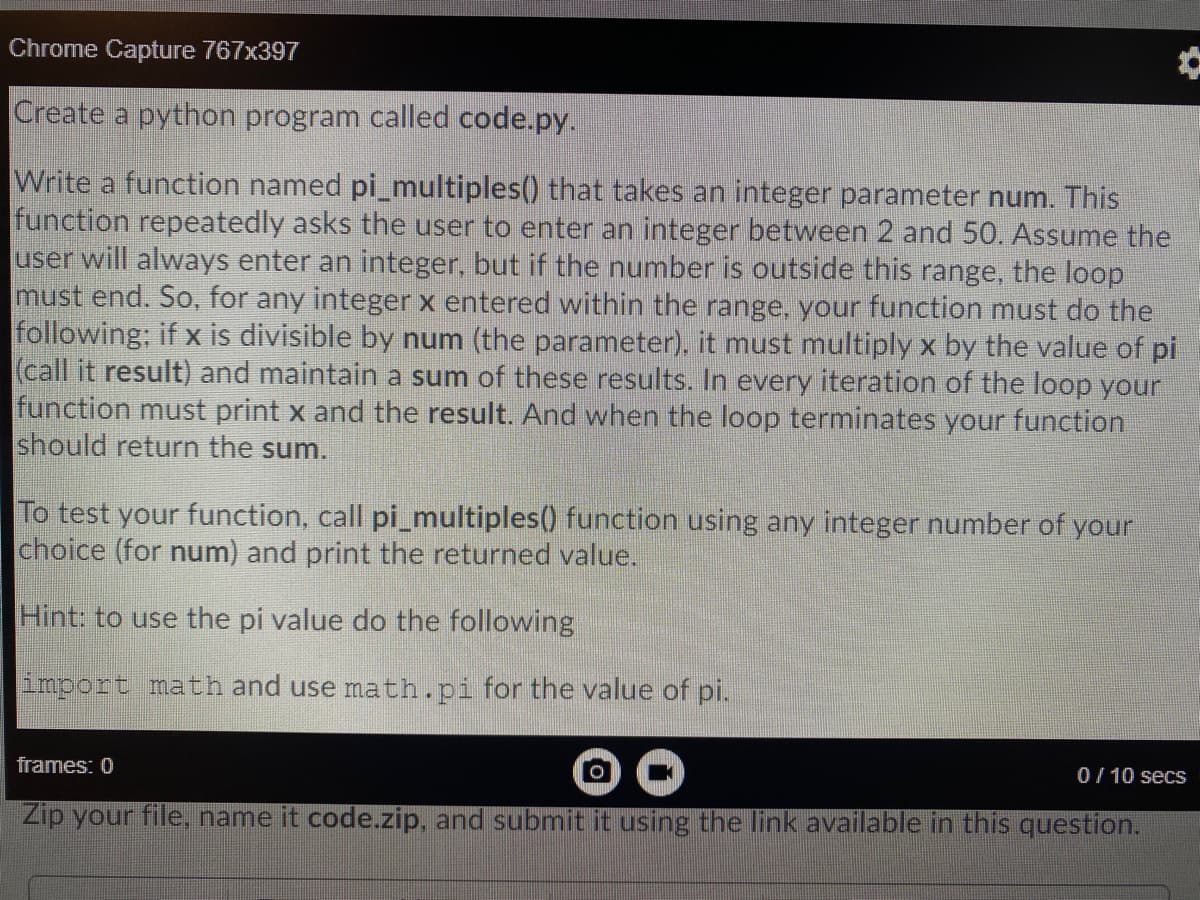
Transcribed Image Text:Chrome Capture 767x397
Create a python program called code.py.
Write a function named pi_multiples() that takes an integer parameter num. This
function repeatedly asks the user to enter an integer between 2 and 50. Assume the
user will always enter an integer, but if the number is outside this range, the loop
must end. So, for any integer x entered within the range, your function must do the
following; if x is divisible by num (the parameter), it must multiply x by the value of pi
(call it result) and maintain a sum of these results. In every iteration of the loop your
function must print x and the result. And when the loop terminates your function
should return the sum.
To test your function, call pi_multiples() function using any integer number of your
choice (for num) and print the returned value.
Hint: to use the pi value do the following
import math and use math.pi for the value of pi.
frames: 0
0/ 10 secs
Zip your file, name it code.zip, and submit it using the link available in this question.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
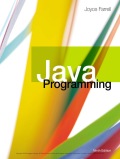
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
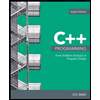
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
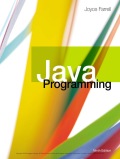
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
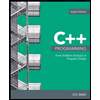
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning