Write a JAVA program that implements the NumberFormatter interface. public interface NumberFormatter { String format(int n); } Include three classes that implement this interface. The DefaultFormatter class formats an integer in the usual way. The DecimalFormatter formats an integer with decimal separators (a comma). The AccountingFormatter formats negative values by surrounding the value with parentheses (not a minus sign). The main method should instantiate an instance of each of the formatters and use it to format a large value (e.g., 2 million), a negative value (e.g., minus 1365), and a small positive integer (e.g., 256). The results should be right aligned and look like the example below. For example, using the above values, the DefaultFormatter would result in: 2000000 -1365 256 The DecimalFormatter would result in: 2,000,000 -1,365 256 The AccountingFormatter would result in: 2000000 (1365) 256
Write a JAVA program that implements the NumberFormatter interface.
public interface NumberFormatter {
String format(int n);
}
Include three classes that implement this interface. The DefaultFormatter class formats an integer in the usual way. The DecimalFormatter formats an integer with decimal separators (a comma). The AccountingFormatter formats negative values by surrounding the value with parentheses (not a minus sign).
The main method should instantiate an instance of each of the formatters and use it to format a large value (e.g., 2 million), a negative value (e.g., minus 1365), and a small positive integer (e.g., 256). The results should be right aligned and look like the example below.
For example, using the above values, the DefaultFormatter would result in:
2000000
-1365
256
The DecimalFormatter would result in:
2,000,000
-1,365
256
The AccountingFormatter would result in:
2000000
(1365)
256

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

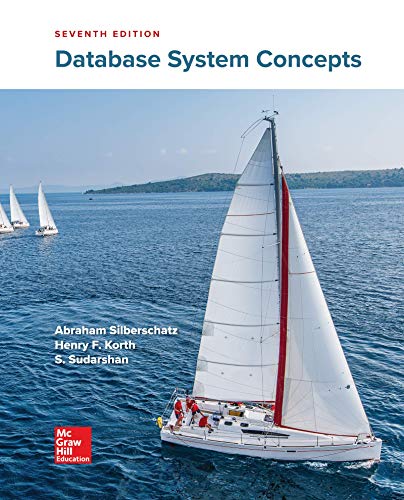
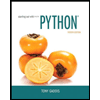
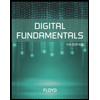
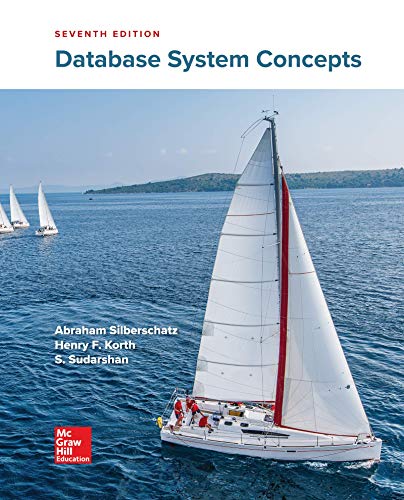
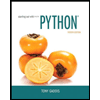
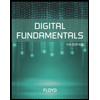
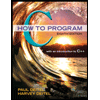
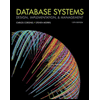
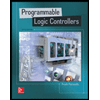