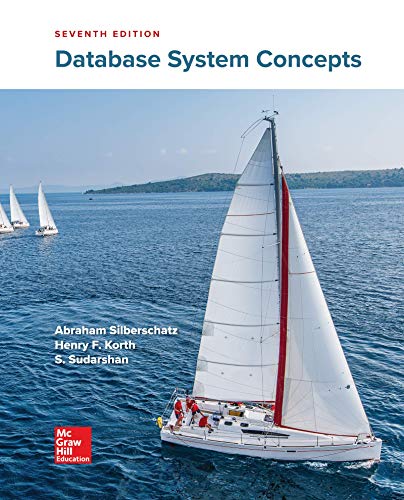
Write a JAVA program that reads a number between 1,000 and 999,999 from the user, where the user enters a comma in the input. Then print the number without a comma. Here is a sample dialog:
Please enter an integer between 1,000 and 999,999: 24,562
24562
Hint: Read the input as a string. Measure the length of the string. Suppose it contains n characters. Then extract the substring consisting of the first n-4 characters and the last three characters

Approach
Here we will first read the input as a string with comma and then we will print out subtring consisting of first n-4 characters (index 0 to length-5) and the last three characters (index length-3 to length-1).
Syntax: string.substring(int startingIndex, int lastIndex);
Here lastIndex is exclusive of the sustring.
Code
import java.util.*;
public class Main
{
public static void main(String[] args) {
Scanner sc= new Scanner(System.in);
System.out.print("Please enter an integer between 1,000 and 999,999: ");
String str= sc.nextLine();
int len = str.length();
if(len<5 || len >7 || str.charAt(len-4)!=','){
System.out.println("Input number entered is not correct");
}
System.out.println(str.substring(0,len-4)+str.substring(len-3,len));
}
}
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- Write a Java program to find the number of words in the user entered String. for example: - i/p : o/p : 3 "Awaskar the vdarada"arrow_forwardWrite a Java Program to take a string from the user and Your task is to find the length of longest palindrome string that can be made from that string.arrow_forwardWrite a program in Java to count the total number of vowels and consonants in a String. The string can contain all the alphanumeric and other special characters as well. However, only the lowercase English alphabets are allowed in the String.arrow_forward
- In JAVA language. Given a string jv = "JAVA", write a program such that the string to be printed in output should be "AVAJJAVA".arrow_forwardWrite a Java Program to take a string from the user and your task is to print the length of the longest palindromic string which can be formed from the user. per string character.arrow_forwardI need help with this Java problem as it's explained in the image below: Palindrome (Deque) A palindrome is a string that reads the same backwards and forwards. Use a deque to implement a program that tests whether a line of text is a palindrome. The program reads a line, then outputs whether the input is a palindrome or not. Ex: If the input is: senile felines! the output is: Yes, "senile felines!" is a palindrome. Ex: If the input is: rotostor the output is: No, "rotostor" is not a palindrome. Ignore punctuation and spacing. Assume all alphabetic characters will be lowercase. Special case: A one-character string is a palindrome. Hint: The deque must be a Deque of Characters, but ordinary chars will be automatically converted to Characters when added to the deque. Java Code: import java.util.Scanner; import java.util.LinkedList; import java.util.Deque; public class LabProgram { public static void main(String[] args) { Scanner scnr = new…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
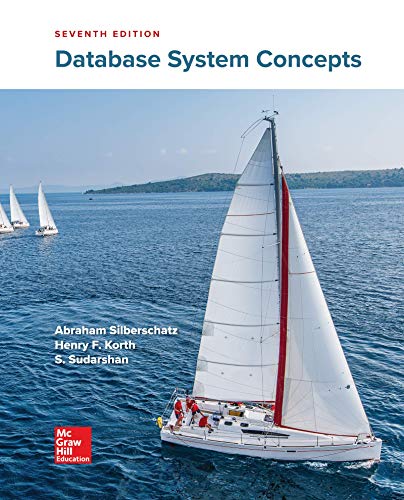
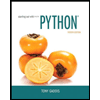
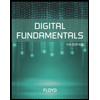
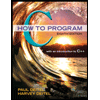
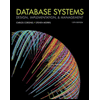
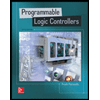