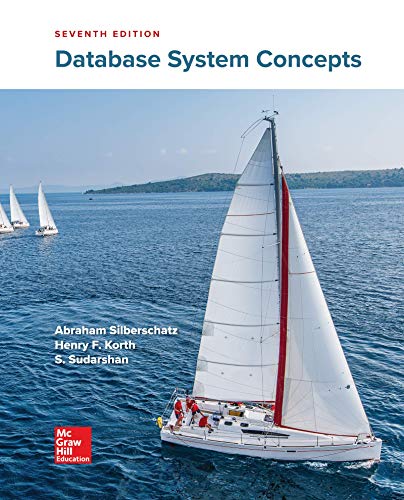
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
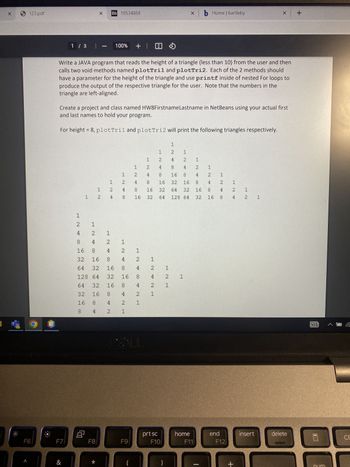
Transcribed Image Text:## JAVA Triangle Program Tutorial
### Overview
This tutorial guides you through writing a JAVA program that reads the height of a triangle (less than 10) from the user. It then calls two void methods named `plotTri1` and `plotTri2`. These methods print triangles of numbers, which are left-aligned.
### Instructions
1. **Project Setup:**
- Create a project and class named `HW8FirstnameLastname` in NetBeans, replacing `Firstname` and `Lastname` with your actual name.
2. **Method Description:**
- Both `plotTri1` and `plotTri2` methods should take a parameter for the triangle's height and use `printf` inside nested For loops.
- The output should produce triangles with the specified height.
3. **Example:**
- For height = 8, the methods `plotTri1` and `plotTri2` will print the following:
#### `plotTri1` Output
```
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
1 5 10 10 5 1
1 6 15 20 15 6 1
1 7 21 35 35 21 7 1
```
#### `plotTri2` Output
```
1
2 1 2
4 2 1 2 4
8 4 2 1 2 4 8
16 8 4 2 1 2 4 8 16
32 16 8 4 2 1 2 4 8 16 32
64 32 16 8 4 2 1 2 4 8 16 32 64
128 64 32 16 8 4 2 1 2 4 8 16 32 64 128
```
### Explanation of the Triangles
- **`plotTri1`:** This triangle mirrors Pascal's Triangle, where each number is
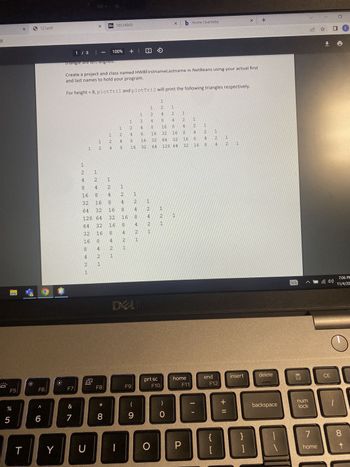
Transcribed Image Text:**Creating Triangles Using Java in NetBeans**
In this exercise, you will create a project and class named `HW8FirstnameLastname` in NetBeans, replacing "FirstnameLastname" with your actual first and last names. The task involves programming code to display two distinct triangular patterns using numbers.
### Task Details
For a given height of 8, the functions `plotTri1` and `plotTri2` will generate and print two triangles as follows:
**Triangle Pattern 1 (`plotTri1`):**
This pattern displays Pascal's Triangle aligned to the left. Each subsequent row shows the binomial coefficients for that particular number:
```
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
1 5 10 10 5 1
1 6 15 20 15 6 1
1 7 21 35 35 21 7 1
```
**Triangle Pattern 2 (`plotTri2`):**
This pattern also shows a numerical series, similar to Pascal's Triangle, but aligned to the right:
```
1
1 1
1 2 1
1 4 6 4 1
1 8 16 32 16 8 1
1 16 32 64 128 64 32 16 1
1 32 64 128 256 256 128 64 32 1
```
### Instructions
1. **Set Up the Project:**
- Open NetBeans and create a new project.
- Name the project and class as described, replacing "FirstnameLastname" with your actual names.
2. **Implement the Plot Functions:**
- Write a method `plotTri1()` to print the first triangle using nested loops.
- Write another method `plotTri2()` to print the second triangle, ensuring proper alignment.
### Learning Outcomes
- Understand how to use loops to generate complex patterns.
- Familiarize yourself with Pascal’s Triangle and the concept of binomial coefficients.
- Gain experience in formatting output for better readability and presentation in code.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a program using while loop and for loops to print first 50 prime numbers in 5 lines, each line containing 10 numbers. 1. Create a program called CountCharacters.java. 2. At the top of your java file add the following documentation comments. Replace the italicized text with the appropriate content. /** @author Your Name CS 110 Section 012 Lab 9 Final Today's Date 3. Import the Scanner class and ask user to enter a line. Implement the while loop so that the user can input any number of lines and one line at a time. To stop entering lines, the user can write "quit. (Hint: Use the string method <str>.equals() in the while condition to stop the while loop OR a break keyword). 4. Implement the for loop inside the while loop to iterate through the line one character at a time to count and output the number of blank spaces, alphabetic characters, digit characters, and other characters in all the lines. You need to use different methods of character class like charAt(), isDigit(),…arrow_forwardQuestion: You are to write a Java program correctly that will prompt for and read 1 string entered by the user which may contain spaces, and display just the vowels in the string in the order they appear, and all other characters in the order they appear. Be sure to use the same format and wording as in the sample runs in the table below. The vowels are a, e, i, o and u. You can use the loop of your choice to solve the problem.arrow_forwardWrite a Java program that: Ask user to enter the last two digits of the Student ID number and store it in a variable of type integer; Use a while loop to print all the positive odd numbers smaller than the inputted number. After the while loop, make a for loop to print all the positive even numbers smaller than the inputted Note: Your answer should have the code as text, as well as a screenshot of the code with the output. The inputted number should be the last two digits of your Student ID number. ID is : 190058401arrow_forward
- The following program is supposed to ask the user for the month as a string and the day of the month as an integer. You need to complete the java program segment at the locations of #### your code here to acquire the input from the user using a Scanner. Assume there is no user input error and both methods, find day() and out weekday(), already exist and work correctly. import java.util.scanner; public class OutDays public static void main(String[] args) V/ #### your code here for .. int year day, weekday; String month; String outday = """; System.out.printf("Enter the monthin") ; f/ #### your code here ... System.out.printf("Enter the day n"); // #### your code here ... weekday = find day (month, day): outday = out weekday(weekday) ; System.out.printf(The day is: $s%n", outday): LGarrow_forwardWrite a program with the most appropriate loop that repeats user entered number of times (say the variable counts holds that number), asking the user counts times to enter numbers. The loop should also calculate the sum and the average of the numbers entered. Be sure to include the following: A class header with an appropriately named class. A main method. Use Scanner class for keyboard input. Declare and initialize any necessary variables. Use appropriate loop statement. Display any necessary input prompt and the output.arrow_forwardWrite a program called Loopmethod that contains a main method and a method called printNums that prints the numbers from a specified start to end value. A method must be used. Refer to the pic and please show your source code and console.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
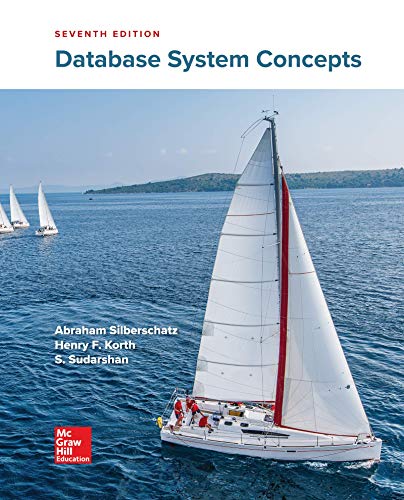
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
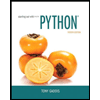
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
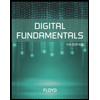
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
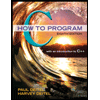
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
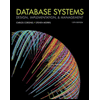
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
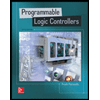
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education