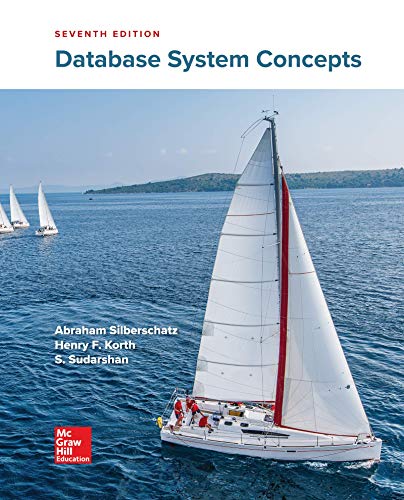
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
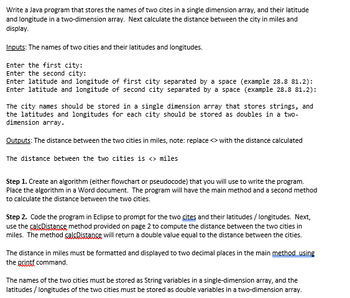
Transcribed Image Text:Write a Java program that stores the names of two cites in a single dimension array, and their latitude
and longitude in a two-dimension array. Next calculate the distance between the city in miles and
display.
Inputs: The names of two cities and their latitudes and longitudes.
Enter the first city:
Enter the second city:
Enter latitude and longitude of first city separated by a space (example 28.8 81.2):
Enter latitude and longitude of second city separated by a space (example 28.8 81.2):
The city names should be stored in a single dimension array that stores strings, and
the latitudes and longitudes for each city should be stored as doubles in a two-
dimension array.
Outputs: The distance between the two cities in miles, note: replace with the distance calculated
The distance between the two cities is <> miles
Step 1. Create an algorithm (either flowchart or pseudocode) that you will use to write the program.
Place the algorithm in a Word document. The program will have the main method and a second method
to calculate the distance between the two cities.
Step 2. Code the program in Eclipse to prompt for the two cites and their latitudes/longitudes. Next,
use the calcDistance method provided on page 2 to compute the distance between the two cities in
miles. The method çalsDistance will return a double value equal to the distance between the cities.
The distance in miles must be formatted and displayed to two decimal places in the main method using
the grintf command.
The names of the two cities must be stored as String variables in a single-dimension array, and the
latitudes / longitudes of the two cities must be stored as double variables in a two-dimension array.
![Use this method to calculate the distance in miles. You will pass the two-dimension array that stores
latitudes / longitudes to the calcDistance method, and the method will return a double.
public static double calcRistance (double [[] list) {
// compute the distance
double Valus
=
(list[0][1] - list[1][1])*55;
double Value = (list[0][0] - list[1][0])*69;
double distance = Math.sect(xValus*xValus + xvalus*xvalue);
return distance;
}
Step 3. Test your program by computing the distance between Orlando and Tampa. The latitudes and
longitudes are provided below. Use the Snip It tool in Windows or a similar tool on the Mac to cut and
paste the Eclipse Console output window into the same Word document as the algorithm in Step 1.
|
Information on how calcDistance calculates the distance in miles.
The math formula for the distance between two points is:
(x1, y1)
(x2, y2)
distance = √(x2-x1)² + (y2-yl)²
difference in longitude (x) difference in latitude (y)
To convert the difference in latitude to miles, multiply by 69
y = (lat1-lat2) * 69;
To convert the difference in longitude to miles, multiply by 55
x = (long1 - long2) * 55;
Once you have calculated the x and y values, which are in miles, use this Java formula to
calculate distance in miles. Note: Math-sout returns a double value.
distance = Math.satt(x*x+y*v)](https://content.bartleby.com/qna-images/question/2f3cf72c-601d-4153-9d70-95173bcd1276/23d8be6e-97a1-4632-b439-c3c740dedeb4/cm3w82u_thumbnail.png)
Transcribed Image Text:Use this method to calculate the distance in miles. You will pass the two-dimension array that stores
latitudes / longitudes to the calcDistance method, and the method will return a double.
public static double calcRistance (double [[] list) {
// compute the distance
double Valus
=
(list[0][1] - list[1][1])*55;
double Value = (list[0][0] - list[1][0])*69;
double distance = Math.sect(xValus*xValus + xvalus*xvalue);
return distance;
}
Step 3. Test your program by computing the distance between Orlando and Tampa. The latitudes and
longitudes are provided below. Use the Snip It tool in Windows or a similar tool on the Mac to cut and
paste the Eclipse Console output window into the same Word document as the algorithm in Step 1.
|
Information on how calcDistance calculates the distance in miles.
The math formula for the distance between two points is:
(x1, y1)
(x2, y2)
distance = √(x2-x1)² + (y2-yl)²
difference in longitude (x) difference in latitude (y)
To convert the difference in latitude to miles, multiply by 69
y = (lat1-lat2) * 69;
To convert the difference in longitude to miles, multiply by 55
x = (long1 - long2) * 55;
Once you have calculated the x and y values, which are in miles, use this Java formula to
calculate distance in miles. Note: Math-sout returns a double value.
distance = Math.satt(x*x+y*v)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a java program (class) called Grades to do the following. Must use an array. Ask the user for 20 grades. Get from the user each grade and fill an array with the grades. Find the average of the elements of the array. Print how many grades are smaller than the average. To get you started, your program code will begin as follows: import java. util. *; //includes Scanner public class Grades { public static void main(String[] args) { //begins main methodarrow_forwardCreate a Java program that will perform the following statements.Perform the following items in a continuous manner. The code solution for the next item will be basedon the code performed on the previous item.1. Create an ArrayList named “apostles” and add the following names to it: Juan, Pedro, Lucas,Judas, Mateo, Marcos and Simon.2. Print the size of the ArrayList to the standard output before and after adding the names.3. Use two methods to print the names in standard output.a. Using For loopb. Using Iterator4. Add the name “Philip” at the end, and add the name “Thomas” between the two names “Juan”and “Pedro”.5. Remove the name “Judas” using its index position.6. Search the names “Marcos”, “James” and “Judas” in the ArrayList “apostles”This part will display the following:Does “apostles” contains Marcos? _______ (it will return a Boolean result)Does “apostles” contains James? _______Does “apostles” contains Judas? _______7. Sort the names of the ArrayList in alphabetical order.8.…arrow_forwardWrite the following Java program Allocate an array a of ten integers. Put the number 17 as the initial element of the array. Put the number 29 as the last element of the array. Fill the remaining elements with –1. Add 1 to each element of the array. Print all elements of the array, one per line. Print all elements of the array in a single line, separated by commas.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
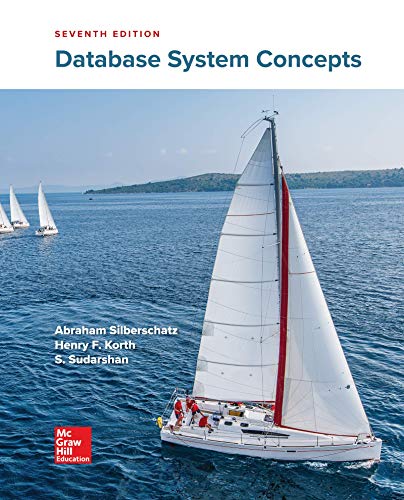
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
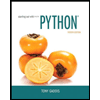
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
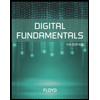
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
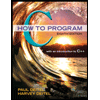
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
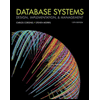
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
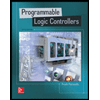
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education