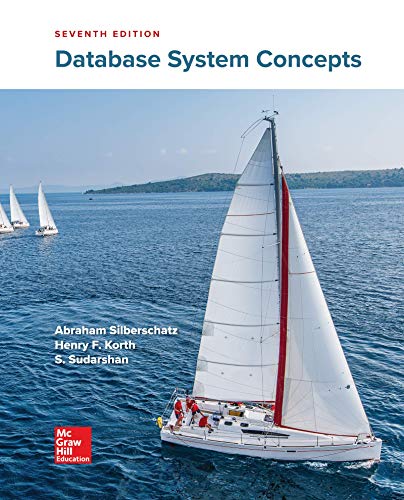
Write a Java program that will ask the user to enter an integer number and then reverses the number. Then check whether the input number and the reversed number are same. If it is display the message that they are same else displays the message that they are not same. Name the program Lab8.java
- Use a one-line comment before each block to describe what you are doing – don’t describe the code syntax, but rather the purpose of the code. These comments should effectively describe the steps in your “
algorithm .”
Sample run:
Please enter a number: 39193
The reversed number is: 39193
The given number and reversed number are same.

Algorithm :
Step 1 : declare variables.
Step 2 : create object of Scanner class.
Step 3 : ask for the number from user.
Step 4 : initialize the variables.
Step 5 : use while loop to check if temp greater than 0.
Step 6 : inside while loop, add the last digit to 10 multiplied by the number to get the reversed number and then remove the last digit from number.
Step 7 : outside while loop print the reversed number.
Step 8 : check if reversed number is equal to number and print the result.
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Write a Java program that allows user to play Rock, Paper and Scissors game with a computer based on the exercise we did in class (which is posted on Moodle). You can refer to Powerpoint Slide #46 to use integers 0, 1, and 2 to represent rock, paper and scissors. At the beginning your program displays a welcome message "Welcome to Rock, Paper and Scissors game with my Computer", it then asks the user to enter his/her choice (only one of the choices: rock, paper and scissors is allowed). You can use a do... while loop to check the validity of the user input. Then your program use a random number generator to pick rock, or paper or scissors for the computer and displays computer’s pick. After that your program displays the result (either you win, or lose or a draw). After one game, your program asks user if he/she wants to continue. If the user decides to stop playing, your program displays how many games you played and how many time you win, lose and tie, and displays a "Thank you for…arrow_forward1. Write Java code to repeatedly print each single digit between 1 and 9 the number of times based upon its numeric value. Thus, you would get a triangle of the shape below where 1 prints once, 2 twice . and 9 prints 9 times. 1 22 333 4444 55555 666666 7777777 88888888 999999999arrow_forwardCreate a Java program that can be used to calculate the average weekly and monthly grocery bill for a family of four: Prompt the user for the coupon amount as a decimal (example, .10). Ensure the value is set to 10% if the value exceeds 100% or is less than or equal to zero. Prompt the user for 2-4 weeks of grocery bills. Calculate the monthly and weekly average for groceries. Display monthly total and weekly average without the coupon. Display monthly total and weekly average with the coupon.arrow_forward
- In Java 8, I want the program to capture the current year and compare it to an input value. Specifically, the user is asked to enter the last two digits of a year that will be output. If those two digits are greater than the last two digits of the current year, the program will print to console a “19” before the two digits the user inputted. If the two digits that the use input are less than or equal to the last two digits of the current year, the program will print to the console a “20” before the two digits the user inputted. For instance, if the current year is 2023, and the user input the integer number “74”, the program would output to the console “1974”. If the current year is 2023, and the user input the integer number “19”, the program would output to the console “2019”. The user is not asked to enter the current year; the program captures that automatically. The user only enter the last two numbers of a year. Hopefully, that's enough to go by and clear enough. I need the code,…arrow_forwardWe all know that one mile is equal to 5,280 feet. Using Eclipse, please write a java program named MilesFeet.java that first asks the program’s user to enter the number of feet he or she wishes to have converted to miles. Then your java program should compute and display the resulting miles on the Eclipse console window along with some appropriate text. Run your code as needed until the program execution is error-free. Save the “ready-to-submit” java code in your CSC111 folder. Sample Console Window Display after program execution:How many feet do you want converted to miles?15840That number ofFeetis equal to 3.0 milesarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
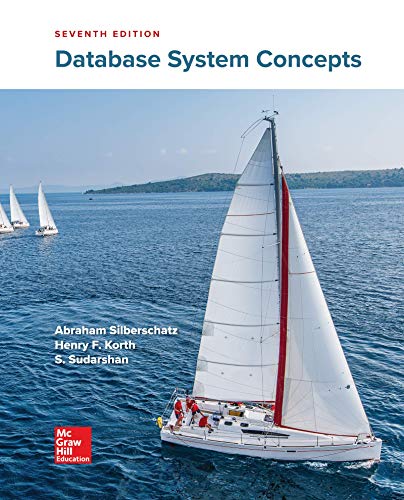
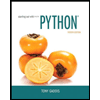
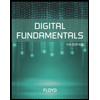
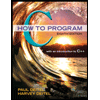
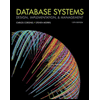
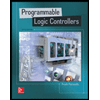