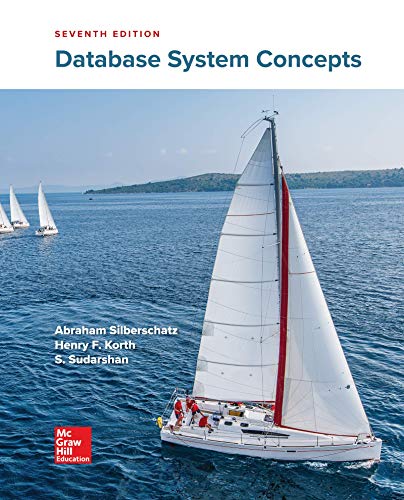
Concept explainers
Write a parallel
#include<stdio.h>
#include<stdlib.h>
#include <string.h>
#define ASCIIs 127 //ASCII characters from 0 to 127
define atmost 1000
char letters[atmost + 1]; //Extra location for the string terminator '\0'
int total_count[ASCIIs];
void find(int count[],
int start, int end);
int main()
{
int i, processes, n, start, end;
int count[ASCIIs], my_rank, segment, p;
printf("Enter a line not larger than 1000 characters.\n");
fgets(letters, atmost,stdin);
printf("How many segments?: ");
scanf("%d", &processes);
//To make the length of the divisible by processes:
while(strlen(letters) % processes != 0) strcat(letters, " ");
n = strlen(letters);
for(i = 0; i < ASCIIs; i++)
total_count[i] = 0;
for(p = 0; p < processes; p++)
{
my_rank = p;
segment = n / processes;
start = my_rank * segment;
end = start + segment;
find(count, start, end);
for(i = 0; i <= ASCIIs; i++)
total_count[i] += count[i];
}
for(i = 33; i < ASCIIs; i++)
if(total_count[i] != 0)
printf("Number of %c is: %d\n", i, total_count[i]);
return 0;
}
void find(int count[], int start, int end)
{
int i;
for(i = 0; i < ASCIIs; i++)
count[i] = 0;
for(i = start; i < end; i++)
count[(int)letters[i]]++;
}
or reload the browserDisable in this text fieldRephraseRephrase current sentence2Edit in Ginger×

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Implementing matrix addition is pretty simple; see the program below. However, the given addition function matrix_add_double() does not run at full speed. On the instructors computer, which has 16GB of RAM, the program below shows that the call to matrix_add_double takes 38571 ms, while another, better implementation achieves the same result in 3794.7ms, which is 10.2 times faster! Your job for this exercise is: • to explain what in the Operating System and/or CPU and/or other part of the system makes the implementation below go so slowly. • to fix the code to achieve full speed. Hint: There are two reasons why the code runs slowly. The one reason is related to how virtual addresses get translated to physical addresses, the other reasons is related to another effect in the hardware. Only the performance for the matrix_add_double function() counts for your exam results. You can leave the other parts of the code untouched, but you may also change them.…arrow_forwardSuppose Program A, when run on a certain computer, records a user CPU time of 3 seconds, an elapsed wallclock time of 4 seconds, and a system performance of 10 MFLOP/sec. The computer is capable of either doing calculations in the CPU or doing I/O at any given time, but not both. If the processor is replaced with one that is six times faster without affecting the I/O speed, what will be the new user CPU time, the wallclock time, and the MFLOP/sec performance? User CPU time: 0.5 seconds, Wallclock time: 1.5 seconds, Performance: 60 MFLOP/sec User CPU time: 0.5 seconds, Wallclock time: 1.5 seconds, Performance: 40 MFLOP/sec User CPU time: 0.5 seconds, Wallclock time: 1.5 seconds, Performance: 20 MFLOP/sec ○ User CPU time: 0.5 seconds, Wallclock time: 1.5 seconds, Performance: 40 MFLOP/secarrow_forwardWrite a python program. Add a function called reverse() that outputs a DNA strand in reverse. Example: reverse(AATC) returns CTAA Note: you should not use the built-in reverse method.arrow_forward
- Write a program in python with a function timestable(n), which prints a multiplication table of size n. For example, timestable(5) would print: 12345 2 3 4 5 4 6 8 10 6 9 12 15 8 12 16 20 10 15 20 25Use the function in a program where you ask the user for n and you print the corresponding table.arrow_forwardwrite a python program that prints a 3 column table showing the circumference and area of ten circles of a specified random radius. see sample output. the area and circumference are calculated and printed by a custom function that takes radius as its only arguement. the low and high values for the range of random radii are specifiedby user inputs in main. the main function should then use these high and low values and the random module in a loop to genrrate random integers and execute the custom function with the random integers as radii. in the table, the radius column should be 6 charas. wide with 1 decimal, the circumference column should be 12 charas. wide with 3 decimals, and the area column should be 11 charas. wide with 2 decimals.arrow_forwardWrite a program to handle a user's rolodex entries. (A rolodex is a system with tagged cards each representing a contact. It would contain a name, address, and phone number. In this day and age, it would probably have an email address as well.) Typical operations people want to do to a rolodex entry are: 1) Add entry 2) Edit entry 3) Delete entry 4) Find entry 5) Print all entries 6) Quit You can decide what the maximum number of rolodex entries is and how long each part of an entry is (name, address, etc.). When they choose to edit an entry, give them the option of selecting from the current rolodex entries or returning to the main menu — don't force them to edit someone just because they chose that option. Similarly for deleting an entry. Also don't forget that when deleting an entry, you must move all following entries down to fill in the gap. If they want to add an entry and the rolodex is full, offer them the choice to return to the main menu or select a person to overwrite. When…arrow_forward
- Write a program that prompts the user to enter the marks of 2 quizzes for 10 students. Your program should compute and store the average quiz mark for each studentuse C++arrow_forwardIn Python, Given the 2D list below, convert all values to 255 if they are above a threshold or to 0if they are below. The threshold value is given as input by the user a =[[77,68,86,73],[96,87,89,81],[70,90,86,81]]arrow_forwardWrite a C++ program for the given instructions: Summary Suppose that the first number of a sequence is x, where x is an integer. Define: a0 = x; an+1 = an / 2 if an is even; an+1 = 3 X an + 1 if an is odd. Then there exists an integer k such that ak = 1. Instructions Write a program that prompts the user to input the value of x. The program outputs: The numbers a0, a1, a2, . . . , ak. The integer k such that ak = 1 (For example, if x = 75, then k = 14, and the numbers a0, a1, a2, ..., a14, respectively, are 75, 226, 113, 340, 170, 85, 256, 128, 64, 32, 16, 8, 4, 2, 1.) Test your program for the following values of x: 75, 111, 678, 732, 873, 2048, and 65535.arrow_forward
- Please write a program using python to show the movement of polymerase in transcription elongation model. For example, there are some nucleotides, 'ATCCTGCAAGTC...'. If polymerase chooses the correct complementary nucleotides, it moves forward. if it chooses a wrong nuceotide, it moves backward.arrow_forwardC Programming 1. There is a checkerboard, the size is 8x8 2. Enter the queen's position x,y 3. Output the path the queen can take, up, down, left, right and diagonalarrow_forwardWrite an assembly language program in MIPS that repeatedly asks the user for a scale F or a C (for"Fahrenheit" or "Celsius") on one line followed by an integertemperature on the next line. It then converts the given temperature tothe other scale. Use the formulas:F = (9/5)C + 32C = (5/9)(F - 32)2. Exit the loop when the user types "Q/q". Assume that all input is correct.For example:Enter Scale : FEnter Temperature: 32Celsius Temperature: 0CEnter Scale : CEnter Temperature: 100Fahrenheit Temperature: 212FEnter Scale : Qdoneprovide a picture of the output.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
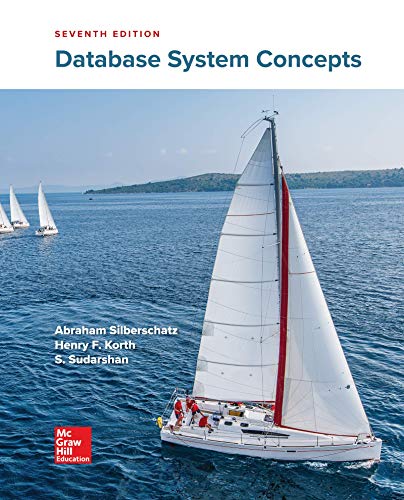
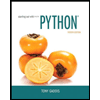
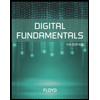
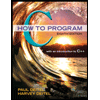
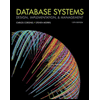
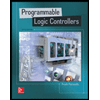