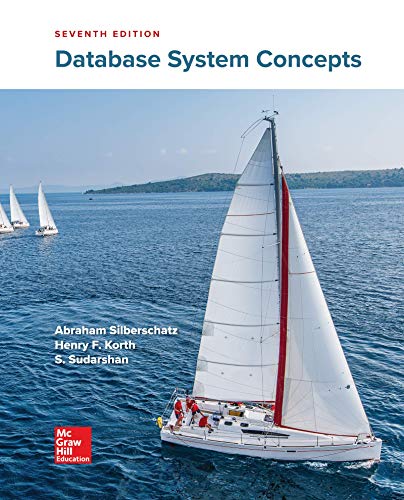
5.22 LAB: Array palindrome (JAVA)
Write a program in java that reads a list of integers from input and determines if the list is a palindrome (values are identical from first to last and last to first). The input begins with an integer indicating the length of the list that follows. Assume that the list will always contain fewer than 20 integers. Output "yes" if the list is a palindrome and "no" otherwise. The output ends with a newline.
Ex: If the input is:
6 1 5 9 9 5 1the output is:
yesEx: If the input is:
5 1 2 3 4 5the output is:
noimport java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int[] userValues = new int[20]; // List of integers from input
/* Type your code here. */
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- 7.19 LAB: Adjust list by normalizing When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by dividing all values by the largest value. The input begins with an integer indicating the number of floating-point values that follow. Assume that the list will always contain less than 20 floating-point values. For coding simplicity, follow every output value by a space, including the last one. And, output each floating-point value with two digits after the decimal point, which can be achieved as follows:printf("%0.2lf ", yourValue); Ex: If the input is: 5 30.0 50.0 10.0 100.0 65.0 the output is: 0.30 0.50 0.10 1.00 0.65 The 5 indicates that there are five floating-point values in the list, namely 30.0, 50.0, 10.0, 100.0, and 65.0. 100.0 is the largest value in the list, so each value is divided by 100.0.…arrow_forwardPython 12.10 LAB: Sorting TV Shows (dictionaries and lists) Write a program that first reads in the name of an input file and then reads the input file using the file.readlines() method. The input file contains an unsorted list of number of seasons followed by the corresponding TV show. Your program should put the contents of the input file into a dictionary where the number of seasons are the keys, and a list of TV shows are the values (since multiple shows could have the same number of seasons). Sort the dictionary by key (greatest to least) and output the results to a file named output_keys.txt. Separate multiple TV shows associated with the same key with a semicolon (;), ordering by appearance in the input file. Next, sort the dictionary by values (in reverse alphabetical order), and output the results to a file named output_titles.txt. Ex: If the input is: see imagesarrow_forward5.7 Generate a text-based histogram for a quiz given to a class of students. The quiz isgraded on a scale from 0 to 5. Write a program that allows the user to enter gradesfor each student. As the grades are being entered, the program should count, usingan array, the number of 0s, the number of 2s, the number of 4s. The program should be capable ofhandling an arbitrary number of student grades.You can do this by making an array of size 6, where each array element is initializedto zero. Whenever a zero is entered, increment the value in the array at index 0.Whenever a one is entered, increment the value in the array at index 1, and so on,up to index 5 of the array. SAMPLE RUN #1: ./5-7 Interactive Session Standard Input Standard Error (empty) Standard Output Hide Invisibles Highlight: NoneStandard Input OnlyPrompts OnlyStandard Output w/o PromptsFull Standard OutputAllShow Highlighted Only Enter·student's·grade·0-5·or·outside·this·range·to·end:3↵…arrow_forward
- 5.18 LAB: Output numbers in reverse Write a program that reads a list of integers, and outputs those integers in reverse. The input begins with an integer indicating the number of integers that follow. For coding simplicity, follow each output integer by a comma, including the last one. Ex: If the input is: 5 2 4 6 8 10 the output is: 10, 8, 6, 4, 2, To achieve the above, first read the integers into a vector. Then output the vector in reverse. 464730 3214874 qx3zqy7 LAB ACTIVITY 5.18.1: LAB: Output numbers in reverse 1 #include 2 #include 3 using namespace std; 4 int BD78 main.cpp // Must include vector library to use vectors vectorarrow_forward8.12 LAB: Binary search Binary search can be implemented as a recursive algorithm. Each call makes a recursive call on one-half of the list the call received as an argument. Complete the recursive method binarySearch() with the following specifications: Parameters: a target integer an ArrayList of integers lower and upper bounds within which the recursive call will search Return value: the index within the ArrayList where the target is located -1 if target is not found The template provides main() and a helper function that reads an ArrayList from input. The algorithm begins by choosing an index midway between the lower and upper bounds. If target == integers.get(index) return index If lower == upper, return -1 to indicate not found Otherwise call the function recursively on half the ArrayList parameter: If integers.get(index) < target, search the ArrayList from index + 1 to upper If integers.get(index) > target, search the ArrayList from lower to index - 1…arrow_forward3.8 LAB: Read values into a list Write a program that reads a list of integers into a list as long as the integers are greater than zero, then outputs the values of the list. Ex: If the input is: 10 IN N WSP 5 3 21 2 -6 the output is: [10, 5, 3, 21, 2] You can assume that the list of integers will have at least 2 values.arrow_forward
- (python) 8. Create a new method called groupingEvens. This method will pass in 1 parameter and return only the even values in the list of values. This method will group together all of the even numbers in a list. INPUT: list of values OUTPUT: No output RETURN: any even numbers, as a list. *The list should be sorted*arrow_forward3.9. TRUE or FALSE (Circle your answer) True / False We can tell if a problem is hard rather than easy based on the number of lines of code required to solve it. True / False Booleans in Python are objects True / False List comprehension is the only way to filter a list True / False Strings are mutable True / False You can perform reduce operation with a list comprehension Looking for even numbers in a list of N numbers can be accomplished in O(N) True / False range(5, 35, 5) will generate a sequence of 7 elements True / False If I double the number of items in a sorted list of numbers, all algorithms will take twice as long to find a number in the new list respect to the original list True / False Subaru is to car like object is to class True/False You can write a function that modifies a list but has no return statement True / False In red-green-blue color notation, we represent black as (255, 255, 255) You can instantiate many classes using an object True False True / False TikTok…arrow_forward13arrow_forward
- javaarrow_forwardI/ implement copyToVec such that: // 1) receives three parameters, an array, the array's size, and a vector // 2) copy elements from the array to the vector. Copy elements from 0 to size-1 // 3) using the RANGE-BASED for Loop ONLY, add 0.9 to every element in the vector /* implement main such that: 1) crate an array of size 100 and type float 2) prompt the user to enter 50 values 3) create a new instance of type vector that can store float values 4) invoke copyVec using this exact statement: copyToVec(arrFloat, 30, vecFloat); 5) Using the RANGE-BASED for Loop. print the values from the vector such that that the output is formatted as shown below. Use the following input test data: 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 vecFloat[0] vecFloat[1] = 1.290e+01 vecFloat[2] vecFloat[3] vecFloat[4] vecFloat[5] = 1.690e+01 vecFloat[6] = 1.790e+01 vecFloat[7] vecFloat[8] = 1.990e+01…arrow_forward2.15 LAB: Descending selection sort with output during execution Write a program that takes an integer list as input and sorts the list into descending order using selection sort. The program should use nested loops and output the list after each iteration of the outer loop, thus outputting the list N-1 times (where N is the size of the list). Important Coding Guidelines: Use comments, and whitespaces around operators and assignments. Use line breaks and indent your code. Use naming conventions for variables, functions, methods, and more. This makes it easier to understand the code. Write simple code and do not over complicate the logic. Code exhibits simplicity when it’s well organized, logically minimal, and easily readable. Ex: If the input is: 20 10 30 40 the output is: [40, 10, 30, 20] [40, 30, 10, 20] [40, 30, 20, 10] Ex: If the input is: 7 8 3 the output is: [8, 7, 3] [8, 7, 3] Note: Use print(numbers) to output the list numbers and achieve the format shown in the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
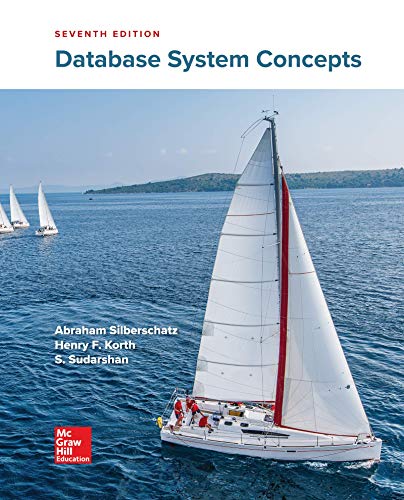
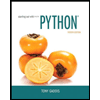
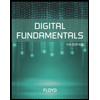
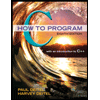
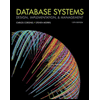
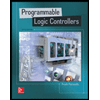