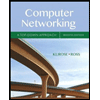
Concept explainers
Write a program named p3.py that starts with an empty list and then asks the user for a positive integer n. Then your program must generate n random integers in the range [1-10] (inclusive) and store them in the list. Your program must then ask the user repeatedly to enter a number and a string to replace all occurrences of that number with the string (e.g., replace 1’s with "hello", 2’s with “hi”) and count the number of times a particular number was replaced by the string.
Hint: use a for (counter controlled) loop inside a while (event-controlled) loop
Sample output: n >> 12
List: [1, 3, 3, 9, 5, 7, 8, 2, 10, 8, 4, 1] number to change ['q' to quit]>> 1 with string >> hello
List: ['hello', 3, 3, 9, 5, 7, 8, 2, 10, 8, 4, 'hello']
The number 1 was replaced 2 times in total
number to change ['q' to quit]>> 8 with string >> hi
List: ['hello', 3, 3, 9, 5, 7, 'hi', 2, 10, 'hi', 4, 'hello']
The number 8 was replaced 2 times in total
number to change ['q' to quit]>> q

Step by stepSolved in 2 steps with 1 images

- Please send me answer of this question immediately and i will give you like sure sirarrow_forwardIn Java: When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by subtracting the smallest value from all the values. The input begins with an integer indicating the number of integers that follow. Assume that the list will always contain less than 20 integers. Ex: If the input is: 5 30 50 10 70 65 the output is: 20 40 0 60 55 For coding simplicity, follow every output value by a space, even the last one. Your program must define and call a method:public static int getMinimumInt(int[] listInts, int listSize) import java.util.Scanner; public class LabProgram {/* Define your method here */ public static void main(String[] args) {/* Type your code here. */}}arrow_forwardA program in javaarrow_forward
- JAVAarrow_forwardplease explain pythonarrow_forwardA user is going to enter numbers one at a time, entering 'q' when finished. Put the numbers in a list, sort it in numerical order, and print out the list. Then print out the middle element of the list. (If the list has an even number of elements, print the one just after the middle.) Remember that a list 1st of numbers can be sorted numerically by calling 1st.sort(), and can be printed with print(1st). You can assume that every entry is either a valid integer or is the letter 'q'. Examples: If the input is 4 3 6 7 3 q The output is [3, 3, 4, 6, 7] 4 If input is 4 3 6 7 3 2 q The output is [2, 3, 3, 4, 6, 7] 4arrow_forward
- Create a program that starts with an empty list, prompts the user three times to enter their favorite game, and then prints out the output using the pop() method. Expected Output What is your favorite game? The Legend What is your second favorite game? Teen What is your third favorite game? Donke One of your favorite games is Donkey Ko One of your favorite games is Teenage M One of your favorite games is The Legenarrow_forwardWrite a program that first gets a list of integers from input. The input begins with an integer indicating the number of integers that follow. Then, get the last value from the input, which indicates a threshold. Output all integers less than or equal to that last threshold value. Assume that the list will always contain less than 20 integers. Ex: If the input is: the output is: 5 50 60 140 200 75 100 The 5 indicates that there are five integers in the list, namely 50, 60, 140, 200, and 75. The 100 indicates that the program should output all integers less than or equal to 100, so the program outputs 50, 60, and 75. For coding simplicity, follow every output value by a comma, including the last one. Such functionality is common on sites like Amazon, where a user can filter results. 5 6 50,60,75, 1 #include 2 3 int main(void) { 4 78991 11 10 } const int NUM_ELEMENTS = 20; int userValues [NUM_ELEMENTS]; /* Type your code here.arrow_forwardWrite a program that first gets a list of integers from input. The input begins with an integer indicating the number of integers that follow. Then, get the last value from the input, which indicates a threshold. Output all integers less than or equal to that last threshold value. Assume that the list will always contain less than 20 integers. Ex: If the input is: 5 50 60 140 200 75 100 the output is: 50,60,75, The 5 indicates that there are five integers in the list, namely 50, 60, 140, 200, and 75. The 100 indicates that the program should output all integers less than or equal to 100, so the program outputs 50, 60, and 75. For coding simplicity, follow every output value by a comma, including the last one. Such functionality is common on sites like Amazon, where a user can filter results. #include <stdio.h> int main(void) { const int NUM_ELEMENTS = 20; int userValues[NUM_ELEMENTS]; // Set of data specified by the user /* Type your code here. */ return 0; }arrow_forward
- Write a program that reads a list of integers, and outputs whether the list contains all even numbers, odd numbers, or neither. The input begins with an integer indicating the number of integers that follow. Ex: If the input is: 5 2 4 6 8 10 the output is: all even Ex: If the input is: 5 1 3 5 7 9 the output is: all odd Ex: If the input is: 5 1 2 3 4 5 the output is: not even or odd Your program must define and call the following two functions. is_list_even() returns true if all integers in the list are even and false otherwise. is_list_odd() returns true if all integers in the list are odd and false otherwise.def is_list_even(my_list)def is_list_odd(my_list) if __name__ == '__main__': both def and if need to be included and functions called from both defs def is_list_even(my_list):for i in range(len(my_list)):if my_list[i] % 2 != 0:return False or Truedef is_list_odd(my_list):for i in range(len(my_list)):if my_list[i] % 2 == 0:return False or Trueif is_list_odd == True:print("all…arrow_forwardWrite a program that first gets a list of integers from input. The input begins with an integer indicating the number of integers that follow. Then, get the last value from the input, which indicates a threshold. Output all integers less than or equal to that last threshold value. Ex: If the input is: 5 50 60 140 200 75 100 the output is: 50,60,75, The 5 indicates that there are five integers in the list, namely 50, 60, 140, 200, and 75. The 100 indicates that the program should output all integers less than or equal to 100, so the program outputs 50, 60, and 75. For coding simplicity, follow every output value by a comma, including the last one. Such functionality is common on sites like Amazon, where a user can filter results. This is my code so far can you let me know what I'm doing wrong please! integers=[]amount_of_integers=int(input())for i in range(amount_of_integers): user_input=int(input()) integers.append(user_input)last_value=integers.pop()for i in…arrow_forwardWrite a program that first gets a list of integers from input. The input begins with an integer indicating the number of integers that follow. Then, get the last value from the input, and output all integers less than or equal to that value. Ex: If the input is: 5 50 60 140 200 75 100 the output is: 50 60 75 The 5 indicates that there are five integers in the list, namely 50, 60, 140, 200, and 75. The 100 indicates that the program should output all integers less than or equal to 100, so the program outputs 50, 60, and 75. For coding simplicity, follow every output value by a space, including the last one. Such functionality is common on sites like Amazon, where a user can filter results. Write your code to define and use two functions:vector<int> GetUserValues()void OutputIntsLessThanOrEqualToThreshold(vector<int> userValues, int upperThreshold) #include <iostream>#include <vector> using namespace std; /* Define your function here */ int main() {/* Type your…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
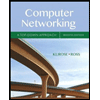
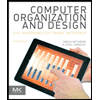
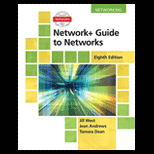
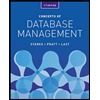
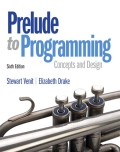
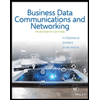