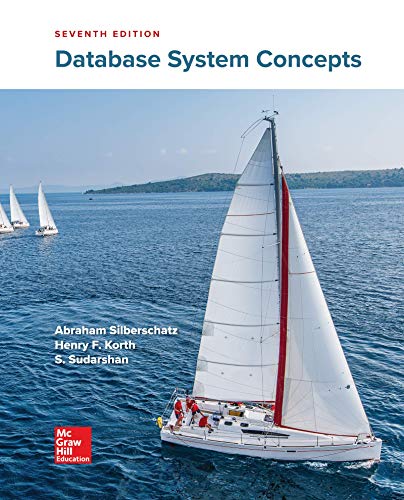
Write a
The numbers function generates 18 random integers, it is required you use the random.randint method to generate these numbers as the unitTest requires this. The range would be 7 to 61 inclusive, (duplicates are okay), and prints them all on one line separated by spaces.
A loop is required for this, and the loop should also total up the integers so the sum can be displayed when the loop ends.
The main() function calls the numbers() function which prints the random numbers and the total as shown below. Do not forget that numbers() function must return variable containing total that was printed.
The output is correct... However my test for the class is coming back with a second error...
Test 1: stimulus:: 0 expected: '7 61 12 56 13 55 14 54 15 53 16 52 17 51 18 50 19 49 ', 'The total is 612' Test 1 Passed.
Test 2 Access numbers function directly
Test 2: stimulus:: expected: 612 Test 2 FailedE
======================================================================
ERROR: test_2_fn_case_1 (__main__.programTestCase)
----------------------------------------------------------------------
Traceback (most recent call last):
AttributeError: module 'program52' has no attribute 'numbers'
Here is my code:
import random
def number():
total: int = 0
num: list = []
for i in range(18):
num = random.randint(7, 61)
print(num, end=" ")
total = total + num
print("")
print("The total is", total)
return total
def main(): # Alternatively if __name__ == '__main__': can be used to begin execution at main.
number()
main()

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Write a program named program52.py that uses main and a void function named numbers that takes no arguments and does not return anything. The numbers function generates 5 random integers, each greater than 10 and less than 30 (duplicates are okay), and prints them all on one line separated by spaces. A loop is required for this, and the loop should also total up the integers so the sum can be displayed when the loop ends. The main function should call the numbers function. SAMPLE OUTPUT12 24 16 21 17The total is 90arrow_forwardIn C++ only Create a function called MultyDieRoll. It should take in an integer and run the function DieRoll that number of times, printing out each result to the console. So for instance, if you pass in a 7, it should output 7 random numbers; if you pass in a 21, it should output 21 random numbers.arrow_forwardPython question, please us a docstring and have comments throughout code Write a function getInteger() that takes two integers num1 and num2 as parameters. If num1 > num2, then the function prints an error message and returns 0. If num1 is equal to num2, the function returns the common value. For cases where num1 < num2, the function prompts the user to enter an integer greater than or equal to num1 and less than or equal to num2 and returns the first valid integer that the user enters. If the user enters something that is not an integer value, the programs should display an error message and re-prompt the user to enter a valid integer Below are some sample executions of the function. Use the while and if-elif statements and a try/except in the solution. Don’t forget to include the docstring and comments. Copy and paste or screen shot the code and the thirteen test cases shown below in your submission. . >>> getInteger(5,4) 5 and 4 are not valid parameters 0…arrow_forward
- Accumulating Totals in a Loop Summary In this lab, you add a loop and the statements that make up the loop body to a Java program that is provided. When completed, the program should calculate two totals: the number of left-handed people and the number of right-handed people in your class. Your loop should execute until the user enters the character X instead of L for left-handed or R for right-handed. The inputs for this program are as follows: R, R, R, L, L, L, R, L, R, R, L, X Variables have been declared for you, and the input and output statements have been written. Instructions Ensure the file named LeftOrRight.java is open. Write a loop and a loop body that allows you to calculate a total of left-handed and right-handed people in your class. Execute the program by clicking Run and using the data listed above and verify that the output is correct.arrow_forwardpython Now write another program that reads the text file created in the program above and calculates the user's weekly pay. The program should begin by prompting the user for the regular hourly pay rate. The program should then loop through the file and report the days and hours worked. The program should finish by calling a custom void function named calc_pay that calculates and prints the weekly pay, including overtime, if applicable. By law, weekly hours in excess of 40 should be paid at 1.5 times the regular hourly rate. The calc_pay function takes two arguments, total hours and hourly pay rate, and it must be imported from a separate module file. Note that three outputs are required for the pay.Sample Output 1Enter your hourly pay rate 25.00Here are your hours this weekMonday hours : 10.0Tuesday hours : 8.0Wednesday hours : 12.0Friday hours : 12.0Saturday hours : 8.0You worked 50.0 hours this weekYou worked 10.0 hours overtimeYour hourly pay rate is $25.00 Regular pay :…arrow_forwardWrite in Python Write a function named max that accepts two float values as arguments and returns the value that is the greater of the two. For example, if 7.2 and 12.1 are passed as arguments to the function, the function should return 12.1. Use the function in a program that prompts the user to enter two float values. The program should display the value that is the greater of the two.arrow_forward
- In python This assignment assess problem solving ability and the use of the modulo operator, functions, lists and nested loops. The Modulo (%) operator returns a remainder of the two numbers divided. e.g. 9 % 2 will yield a 1 because 9/2 = 4 and the remainder is 1. Write one program that will compute both the Least Common Multiple, and the Greatest Common Factor of two random numbers between 2 and 30. The program will display all factors and multiples of the two numbers. When displaying the multiples of the numbers, only display up to the first input times the second input. Use functions to compute the answers. Loop the program so that the user try again. create the loop so that hitting enter will continue. The example is below: Integer1: 12Integer2: 3 The Multiples of 3 are 3, 6, 9, 12, 15, 18, 21, 24, 27, 30, 33, 36, The Multiples of 12 are 12, 24, 36, The lowest common Multiples is 12 The Factors of 3 are 1, 3, The Factors of 12 are 1, 2, 3, 4, 6, 12, The greatest…arrow_forwardInstruction: It should be a C++ PROGRAM INCLUDE THE HEADER FILE, MAIN CPP, AND BSTREE.CPP There is a real program developed by a computer company that reads a report ( running text ) and issues warnings on style and partially corrects bad style. You are to write a simplified version of this program with the following features: Statistics A statistical summary with the following information: Total number of words in the report Number of unique words Number of unique words of more than three letters Average word length Average sentence length An index (alphabetical listing) of all the unique words (see next page for a specific format) Style Warnings Issue a warning in the following cases: Word used too often: list each unique word of more than three letters if its usage is more than 5% of the total number of words of more than three letters Sentence length : write a warning message if the average sentence length is greater than 10 Word length : write a warning message if the…arrow_forwardPython only 1. Define printGrid Use def to define printGrid Use any kind of loop Within the definition of printGrid, use any kind of loop in at least one place. Use any kind of loop Within the loop within the definition of printGrid, use any kind of loop in at least one place. it should look like this when called ; printGrid(['abcd','efgh','ijkl']) Prints: a b c d e f g h i j k l 2. Define getColumns Use def to define getColumns Use any kind of loop Within the definition of getColumns, use any kind of loop in at least one place. Use a return statement Within the definition of getColumns, use return _ in at least one place. it should look like this when called ; getColumns(['abcd','efgh','ijkl']) Out[]: ['aei', 'bfj', 'cgk', 'dhl']arrow_forward
- Code should be in Python. Prompt the user to enter a string of their choosing. Output the string.Ex: Enter a sentence or phrase: The only thing we have to fear is fear itself. You entered: The only thing we have to fear is fear itself. Complete the get_num_of_characters() function, which returns the number of characters in the user's string. We encourage you to use a for loop in this function. Extend the program by calling the get_num_of_characters() function and then output the returned result. Extend the program further by implementing the output_without_whitespace() function. output_without_whitespace() outputs the string's characters except for whitespace (spaces, tabs). Note: A tab is '\t'. Call the output_without_whitespace() function in main().Ex: Enter a sentence or phrase: The only thing we have to fear is fear itself. You entered: The only thing we have to fear is fear itself. Number of characters: 46 String with no whitespace: Theonlythingwehavetofearisfearitself.arrow_forwardYou have been hired by a local organic sod company to write coverage calculator for their website. Organic sod is cut into rectangles 9 square foot in area for lawn installation. Your program will prompt the customer for the length and the width of a rectangular area being covered with sod. Implement the following "pseudocode" to obtain the number of pieces to order. • Compute the area of the rectangle to be covered • Divide the area by 9 • Convert the result to an integer and then add 1 Below is a sample run with Python output in blue and user input in black. Enter length:200 Enter width:40 You should order 889 pieces.arrow_forwardNeed python help running code and others. Functions are ideal for use in menu-driven programs. When the user selects an item from a menu, the program can call the appropriate function. Write a menu-driven program for Burgers. Display the food menu to a user (Just show the 5 options' names and prices - No need to show the details!) Use numbers for the options and the number "6" to exit. If the user enters "6", your code should not ask any other questions, just show a message like "Thank you, hope to see you again!" Ask the user what he/she wants and how many of them. (Check the user inputs) Keep asking the user until he/she chooses the exit option. Use a data structure to save the quantities of the orders. You should use ArrayLists/Array Bags, or LinkedLists/LinkedBags (one or more of them) to save data. Calculate the price. Ask the user whether he/she is a student or a staff. There is no tax for students and a 9% tax for staff. Add the tax price to the total price.…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
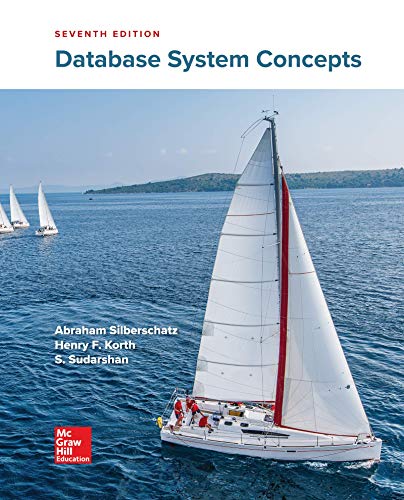
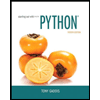
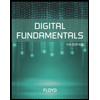
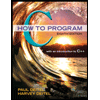
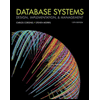
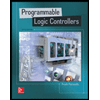