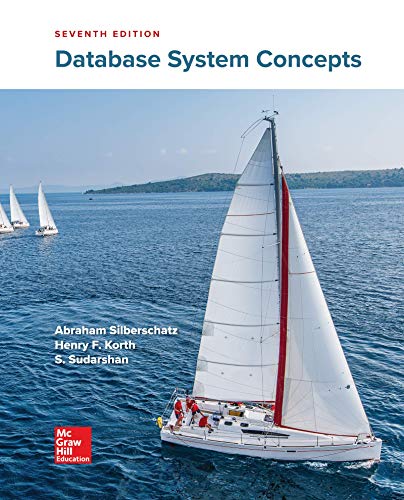
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Write a program that allows the user to sort using the Heap Sort and Bin/Radix Sort. The program should be able to read in data from a binary file. The first element of the binary file will be used to tell how many elements to read in. Once all the data has been read in, the program should sort the data. The user should be able to choose which algorithm to use to sort the data. The program should print the time before and after the sort. The last part of the program should prompt the user for a lower and upper bound. These two values should then be used to decide how much and which part of the array will be display.
Note: The binary files may have duplicate numbers in them. Make sure your sorts are able to handle these cases.
Reminder: The implementation of your algorithms must follow the format we cover in class. Bin sort must be constructed yourself using pointers. No vectors , queues, etc..
Expert Solution

arrow_forward
Step 1: Algorithm:
Heap Sort Algorithm:
Heap Sort is a comparison-based sorting algorithm that uses a binary heap data structure to sort elements in an array.
- Build a max-heap from the array.
- Starting from the middle of the array (index
n/2 - 1
), perform a heapify operation on each element in reverse order, i.e., from right to left.
- Starting from the middle of the array (index
- Extract elements from the max-heap and place them at the end of the array.
- Swap the root of the heap (the maximum element) with the last element in the heap.
- Reduce the size of the heap by 1.
- Perform a heapify operation on the root to maintain the max-heap property.
- Repeat these steps until the heap is empty.
- The array is now sorted in ascending order.
Radix Sort Algorithm:
Radix Sort is a non-comparative sorting algorithm that works on integers by processing individual digits.
- Find the maximum element in the array to determine the number of digits in it.
- Initialize a bucket for each digit (0-9).
- Starting from the least significant digit (rightmost), perform the following steps for each digit position:
- Distribute: Place each element in the appropriate bucket based on the current digit.
- Collect: Collect the elements from the buckets back into the original array in the order they were placed.
- Repeat these steps for each digit position, moving from right to left.
- After processing all digit positions, the array is sorted in ascending order.
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- PROBLEM 1: Have you ever wondered how websites validate your credit card number when you shop online? They do not check a large database of numbers. Most credit providers rely on a checksum formula for distinguishing valid numbers from random collections of digits (or typing mistakes). The objective of this lab you will implement a program that read a file that contains a table with two columns: A column of customer names and a column of credit card numbers. For each customer, print the validity of the credit card number and name of the corresponding credit card company (if the number is valid), For our purpose, the algorithm that valid credit cards is the following: Double the value of every second digit beginning from the right. That is, the last digit is unchanged; the second-to-last digit is doubled; the third-to-last digit is unchanged; and so on. For example, [1,3,8,6] becomes [2,3,16,6] Add the digits of the doubled values and the undoubled digits from the original number. For…arrow_forwardplease help in this ASAParrow_forwardPlease follow the instructions The function first_words in python takes one parameter, fname, the name of a text file, and returns a list containing the first word from each line of the file. For example, if the file contents are: apples are red bananas are yellow limes are green then the list ["apples", "bananas", "limes"] should be returned.NOTE: You may assume the file will contain no blank lines.BIG HINT: If line is a string representing a line of text (inside of a for loop!), then L = line.split() creates a list of the words in the line. For example: Test Result L = first_words("snakes.txt") print(L) ['Cottonmouth', 'Timber', 'Black', 'Tiger', 'Copperhead', 'Eastern', 'Western', 'Eastern', 'Prairie', 'Mojave']arrow_forward
- The function print_last_line in python takes one parameter, fname, the name of a text file. The function should open the file for reading, read the lines of the file until it reaches the end, print out the last line in the file, report the number of lines contained in the file, and close the file. Hint: Use a for loop to iterate over the lines and accumulate the count. For example: Test Result print_last_line("wordlist1.txt") maudlin The file has 5 lines.arrow_forwardIn this lab, we will practice the use of arrays. We will write a program that follows the steps below: Input 6 numbers from the user user inputs numbers one at a time Loop continuously until the exit condition below: ask the user to search for a query number if the query number is in the list: reports the first location of the query number in the list, change the number in that position to 0, show the updated list if the query number is not in the list: exit the loop You must write at least one new function: findAndReplace(array1,...,query) – take in an array and query number as input (in addition to any other needed inputs); it will return the first location of the query number in the list (or -1 if it is not in the list) and will change the number at that location to 0. You may receive the number inputs and print all outputs in int main if you wish. You may report location based on 0-indexing or 1-indexing (but you need 0-indexing to access the array elements!).arrow_forwardPython please: Given the input file input1.csv write a program that first reads in the name of the input file and then reads the file using the csv.reader() method. The file contains a list of words separated by commas. Your program should output the words in a sorted list. The contents of the input1.csv are: hello,cat,man,hey,dog,boy,Hello,man,cat,woman,dog,Cat,hey,boy Example: If the input is input1.csv the output is: ['Cat', 'Hello', 'boy', 'boy', 'cat', 'cat', 'dog', 'dog', 'hello', 'hey', 'hey', 'man', 'man', 'woman'] Note: There is a newline at the end of the output.arrow_forward
- The program will take in a year as an input from the user and output the popular dance and popular slang from that decade. Years, slang, and dances are stored in parallel lists in the starter code. The decade 1920 is located at index 0 and accompanies the 1920’s slang and dance also located at index 0, the decade 1930 is located at index 1 and accompanies the 1930’s slang and dance also located at index 1, etc. Sample Input/Output: >> Year: 1934 In the 1930's, The Jitterbug was the hip dance craze! >> Year: 1989 In the 1980's, The Moonwalk was the gnarly dance craze! Assume the user enters a year between 1920 and 2022, inclusive. To find the decade, round the year down to the nearest decade.arrow_forwardcan you do it pythonarrow_forwardJava Your program must read a file called personin.txt. Each line of the file will be a person's name, the time they arrived at the professor's office, and the amount of time they want to meet with the professor. These entries will be sorted by the time the person arrived. Your program must then print out a schedule for the day, printing each person's arrival, and printing when each person goes in to meet with the professor. You need to print the events in order of the time they happen. In other words, your output will be sorted by the arrival times and the times the person goes into the professor's office. In your output you need to print out a schedule. In the schedule, new students go to the end of the line. Whenever the professor is free, the professor will either meet with the first person in line, or meet with the first person in line if nobody is waiting. Assume no two people arrive at the same time. You should solve this problem using a stack and a queue. You can only…arrow_forward
- Counting the character occurrences in a file For this task you are asked to write a program that will open a file called "story.txt" and count the number of occurrences of each letter from the alphabet in this file. At the end your program will output the following report: Number of occurrences for the alphabets: a was used – times. b was used – times. c was used – times.... .and so, on Assume the file contains only lower-case letters and for simplicity just a single paragraph. Your program should keep a counter associated with each letter of the alphabet (26 counters) [Hint: Use array] Your program should also print a histogram of characters count by adding a new function print Histogram (int counters []). This function receives the counters from the previous task and instead of printing the number of times each character was used, prints a histogram of the counters. An example histogram for three letters is shown below) [Hint: Use the extended asci character 254]: A…arrow_forwardComputer Science Using Java, write a simple Insertion Sort program that can read in integers from a text file (line by line) and sort them into another text file. Use inFile and outFile for the input and output files, respectively. Also make sure that the algorithm keeps track of the comparisons and exchanges performed by the sort so that they may be printed out in the console after the sort is completedarrow_forwardOne of the things discussed in this chapter is the sorting of data. Imagine that a large medical center hires you to write a program that displays a list of potential organ recipients. The hospital's transplant team will consult this list if they have an organ available for transplant. The hospital administrators have instructed you to sort potential recipients by last name and display them in alphabetical order. If more than 10 patients are waiting for a particular organ, the first 10 patients will be displayed; a doctor can either select one or move on to view the next 10 patients. You are concerned that this program will unfairly select patients whose last names begin near the front of the alphabet. It's critical that the hospital has a program written quickly, as the hospital currently has no method of going through potential organ recipients. Based on this, answer the following questions in detail: Would you write and install the program? If yes, would you change anything about…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
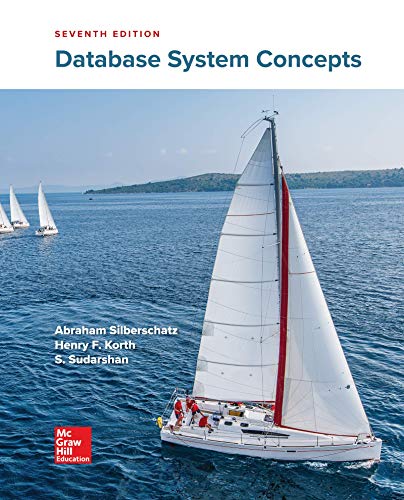
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
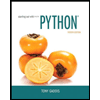
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
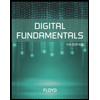
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
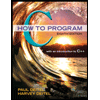
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
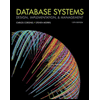
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
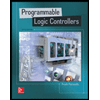
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education