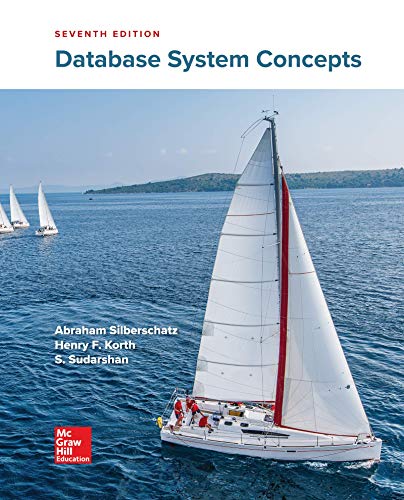
Write a program that creates a 2D array of integers of size nXn initialized with test data.
The program should have the following functions:
● getTotal. This function should accept a 2D array as an argument and return
the total of all the values in the array.
● getAverage. This function should accept a 2D array as an argument and return
the average of all the values in the array.
● getRowTotal. This function should accept a 2D array as the first argument and
an integer as its second argument. The second argument should be the subscript
of a row in the array. The function should return the total of the values in the
specified row.
● getColumnTotal. This function should accept a 2D array as the first argument
and an integer as its second argument. The second argument should be the
subscript of a column in the array. The function should return the total of the
values in the specified column.
● getHighestInRow. This function should accept a 2D array as the first
argument and an integer as its second argument. The second argument should
be the subscript of a row in the array. The function should return the highest
value in the specified row.
● getLowestInRow. This function should accept a 2D array as the first argument
and an integer as its second argument. The second argument should be the
subscript of a row in the array. The function should return the lowest value in the
specified row.
Demonstrate each of the functions in your program using switch structure to design
menu for function call.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Write a program in which the main function reads an integer array data_items of size 10 and an integer variable num. It then passes the array data_items and the num variable to a function called count_divisors which counts the number of elements of array data_items that are divisible by num and returns the count to the main program which prints it. Sample Output: Enter 10 integer for the array: 12 14 16 32 8 33 1 21 44 64 Enter a number to test as a divisor of array elements:8 There are 4 elements divisible by 8arrow_forwardWrite a program that simulates a lottery. The program should have an array of five integers named winningDigits, with a randomly generated number in the range of 0 through 9 for each element in the array. The program should ask the user to enter five digits and should store them in a second integer array named player. The program must compare the corresponding elements in the two arrays and count how many digits match. For example, the following shows the winningDigits array and the Player array with sample numbers stored in each. There are two matching digits, elements 2 and 4. The grand prize winner is determined by all 5 matching digits. The program should use functions for the following processes (3):Random number generation for lotteryPrompt for user data entryMatch Processing and Winner Determinationarrow_forwardUsing C++ Language In this program, you will write a program that uses a function to compute the maximum value in a randomly generated array of integers. Inside your main function, declare an array of size 10. Using a suitable loop, generate seeded randomly generated numbers between 50 and 100 inclusive. Display the randomly generated numbers using a suitable message. Call a function that returns the maximum value of the array. You will need to pass this array to a function. The function does not need to modify the array, hence pass it as a constant array. Use a global constant for the size of the array. You must display the maximum value of the array in the main function.arrow_forward
- an int variable k, an int array currentMembers that has been declared and initialized, an int variable nMembers that contains the number of elements in the array, an int variable memberID that has been initialized, and a bool variable isAMember, Write code that assigns true to isAMember if the value of memberID can be found in currentMembers, and that assigns false to isAMemberotherwise. Use only k, currentMembers, nMembers, and isAMember.arrow_forwardCreate a function using Java: Number of Rows = 3-6 Number of Columns = 3-6 Function Name: winInDiagonalFSParameters: board: 2D integer array, piece: integerReturn: booleanAssume board is valid 2D int array, piece is X==1/O==2.Look at all forward slash / diagonals in the board. If any forward slash / diagonals has at least 3consecutive entries with given type of piece (X/O) (3 in a row XXX, OOO), then return true,otherwise falsearrow_forwardC languagearrow_forward
- Write a function named "changeCase" that takes an array of characters terminating by NULL character (C-string) and a boolean flag of toUpper. If the toUpper flag is true, it will go through the array and convert all lowercase characters to uppercase. Otherwise, it will convert all uppercase to lowercase. For example, if the array is {'H', 'e', 'l', 'l', 'o', '\0'} and the flag is true, then the array will become {'H', 'E', 'L', 'L', 'O', '\0'}. And if the flag is false, the array will become{'h', 'e', 'l', 'l', 'o', '\0'}arrow_forwardCreate a function named "onlyOdd".The function should accept a parameter named "numberArray".Use the higher order function filter() to create a new array that only containsthe odd numbers. Return the new array.// Use this array to test your function:const testingArray = [1, 2, 4, 17, 19, 20, 21];arrow_forwardWrite a function named swapFrontback that takes as input an array ofintegers and an integer that specifies how many entries are in the array. Thefunction should swap the first element in the array with the last elements inthe array. The function should check if the array is empty to prevent errors.The header file for the swapFrontback() is:swapFrontback.h:double swapFrontback (int [] arr,int size);Write a driver (main.c) to test your function with arrays of different lengthand with varrying front and back numbers. Print the array elements beforeand after the swap in the main.c.Sample Run: Original Array: 1 2 3 4 5 6 FrontBack Array: 6 2 3 4 5 1arrow_forward
- Write a function named rockPaperScissors. It should have one input:- An array with 2 elements, each representing a player in the game of Rock Paper Scissors.It should return one output- A scalar with the result of the game.For the purposes of this program, assume the following numerical code:- 1 is rock- 2 is paper- 3 is scissorsIn the game of Rock, Paper, Scissors, rock beats scissors, paper beats rock, and scissors bears paper. Ifplayers play the same object, the result is a tie.Your output should be:- The index of the winning player if there is a winner.- 0 if there is a tie- -1 if a player played an invalid object (a number that is not 1, 2, or 3)For example, if we called our function:result = rockPaperScissors([1 2]);result would be 2, as paper beats rock.If we called our functionresult = rockPaperScissors([3 2]);result would be 1 because scissors beats papearrow_forwardPrompt: In Python language, write a function that applies the logistic sigmoid function to all elements of a NumPy array. Code: import numpy as np import math import matplotlib.pyplot as plt import pandas as pd def sigmoid(inputArray): modifiedArray = np.zeros(len(inputArray)) #YOUR CODE HERE: return(modifiedArray) def test(): inputs = np.arange(-100, 100, 0.5) outputs = sigmoid(inputs) plt.figure(1) plt.plot(inputs) plt.title('Input') plt.xlabel('Index') plt.ylabel('Value') plt.show() plt.figure(2) plt.plot(outputs,'Black') plt.title('Output') plt.xlabel('Index') plt.ylabel('Value') plt.show() test()arrow_forwardUsing either pseudocode of C++ code, write a function that takes three parameters and performs a sequential search. The first parameter is an array of integers. The second parameter is an integer representing the size of the array. The third parameter takes the value to be search form. The function should return the subscripts at which the value is found or -1 is the array does not contain the search term. Please dont use vectors and explain each steparrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
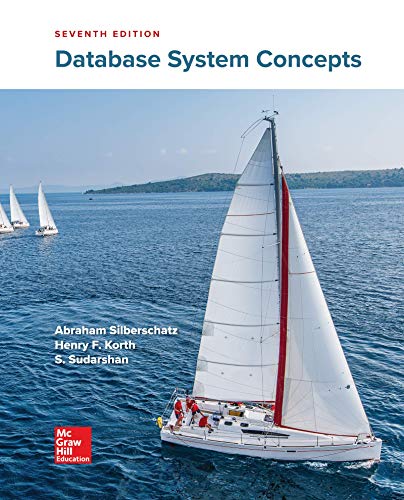
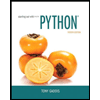
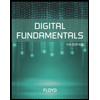
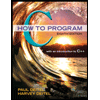
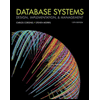
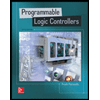