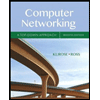
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
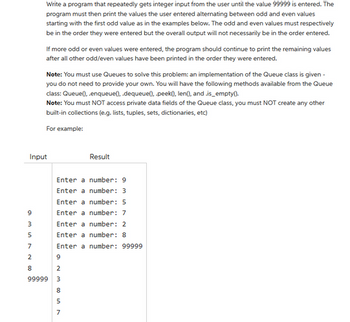
Transcribed Image Text:9
am
Input
3
5
7
~ 00
2
Write a program that repeatedly gets integer input from the user until the value 99999 is entered. The
program must then print the values the user entered alternating between odd and even values
starting with the first odd value as in the examples below. The odd and even values must respectively
be in the order they were entered but the overall output will not necessarily be in the order entered.
8
If more odd or even values were entered, the program should continue to print the remaining values
after all other odd/even values have been printed in the order they were entered.
Note: You must use Queues to solve this problem: an implementation of the Queue class is given -
you do not need to provide your own. You will have the following methods available from the Queue
class: Queue), enqueue(), .dequeue(), peek(), len(), and.is_empty().
Note: You must NOT access private data fields of the Queue class, you must NOT create any other
built-in collections (e.g. lists, tuples, sets, dictionaries, etc)
For example:
Enter a number: 9
Enter a number: 3
Enter a number: 5
Enter a number: 7
Enter a number: 2
Enter a number: 8
Enter a number: 99999
9
WN6
Result
2
99999 3
857
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Similar questions
- use java programingarrow_forwardVoltage readings are obtained from an electrical substation once every hour for six hours (so there are sixreadings). Write a program to perform the following checks on the substation:i) display all voltages that differ from the average by more than 10% of the average.ii) display all pairs of consecutive hours where the change from the voltage at one hourto the next is greater than 15% of the average.arrow_forwardIn various applications, you are often asked to compute the mean and standard deviation of data. The mean is simply the average of the numbers. The standard deviation is a statistic that tells you how tightly all the various data are clustered around the mean in a set of data. For example, what is the average age of the students in a class? How close are the ages? If all the students are the same age, the deviation is 0. Write a program that prompts the user to enter any number of values into a double array, and then calculates and displays the mean and standard deviations of these numbers using the following formulas: mean)? 2i +x2 + .. + xn i=1 i=1 mean = deviation = п - 1arrow_forward
- Write a program that asks the user to enter 2 integers of 4 digits and displays thedifferent digits as X and * if they are the same. If the user enters a number which is notof four digits, then a convenient message is displayed.arrow_forwardCreate a program that allows the user to enter a person's age (in years) and current salary. Both Next, you plan the algorithm and then desk-check it. Figure 8-15 shows the completed IPO algorithm contains two loops. The outer loop keeps track of the three annual raise rates (3%, 4%, chart and desk-check table, which (for simplicity) uses an age of 62 and a salary of $25000. The before retirement at age 65, using annual raise rates of 3%, 4%, and 5%. To calculate then for the input. In this case, the program should display a person's total earnings in Figure 8-15. You begin by analyzing the problem, looking first for the output and In this lab, you will plan and create an algorithm for the problem specification shown Frepure 8-15 Problem specification, IPO chart, and desk-check table for the retirement algorithm (continues) and 5%). The nested loop keeps track of the number of years until retirement. the amounts, the program will need to know the person's age and current salary. The…arrow_forwardWrite code that iterates while userNum is less than 12. Each iteration: Put userNum to output. Then, put "/" to output. Then, assign userNum with userNum multiplied by 3.arrow_forward
- Transient PopulationPopulations are affected by the birth and death rate, as well as the number of people who move in and out each year. The birth rate is the percentage increase of the population due to births and the death rate is the percentage decrease of the population due to deaths. Write a program that displays the size of a population for any number of years. The program should ask for the following data: The starting size of a population P The annual birth rate (as a percentage of the population expressed as a fraction in decimal form)B The annual death rate (as a percentage of the population expressed as a fraction in decimal form)D The average annual number of people who have arrived A The average annual number of people who have moved away M The number of years to display nYears Write a function that calculates the size of the population after a year. To calculate the new population after one year, this function should use the formulaN = P + BP - DP + A - Mwhere N is the…arrow_forwardIn various applications, you are often asked to compute the mean and standard deviation of data. The mean is simply the average of the numbers. The standard deviation is a statistic that tells you how tightly all the various data are clustered around the mean in a set of data. For example, what is the average age of the students in a class? How close are the ages? If all the students are the same age, the deviation is 0. Write a program that prompts the user to enter any number of values into a double array, and then calculates and displays the mean and standard deviations of these numbers using the following formulas: (z - mean) z+z3+ *** +zn deviation = mean = n-1 Required Methods You must write your program so that the following methods are defined/implemented and used (called): • /* Compute the deviation of double values */ public static double deviation (double[] x) • /* Compute the mean of an array of double values */ public static double mean (double[] x) Sample Run (user input…arrow_forwardFor this question, you will write a program that prompts the user to enter an integer value between 1 and 1000 (exclusive). Your program should then print out a sequence of numbers between the given value and 1 (inclusive) following the pattern below:For a given number ai, If ai is even, then ai+1 = floor(ai / 2) If ai is odd, then ai+1 = 3ai + 1 Your program should stop printing numbers once the sequence reaches the value of 1. If the user enters a number that is not between 1 and 1000 (exclusive), print "Invalid input." and prompt the user for a number again. This sequence is referred to as the Collatz sequence. The Collatz Conjecture states that this sequence will eventually reach the value of 1 for any starting number, a fact that has not yet been proven by modern mathematics! Note: floor(x) means that x should be rounded down to the nearest integer.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
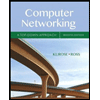
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
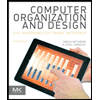
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
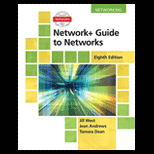
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
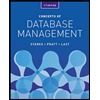
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
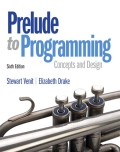
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
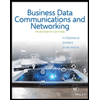
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY