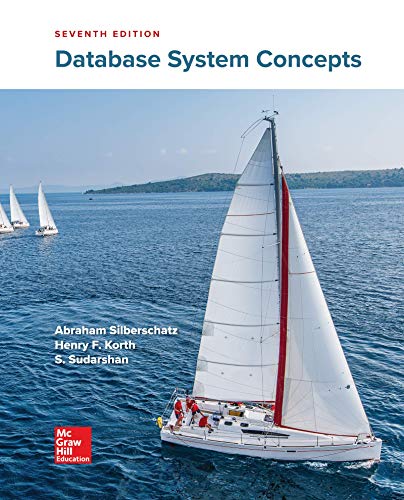
Write a
Game company
Type of Game (Action, Adventure, Sports etc.)
Year of Sale
Sale Price
The program should use an array of at least 3 structures (3 variables of the same structure). It should let the user enter data into the array, change the contents of any element and display the data stored in the array. The program should have a menu driven interface.
Input Validation: When the data for a new game sale is entered, be sure the user enters data for all the fields. No negative amounts should be entered for a “Sale Price” of the Game
An example:
You will use 1 structure only and then manipulate that for working with the information.
The program should use an array of at least 3 structures (3 variables of the same structure).
An example of the structure:
struct Game
{
string name;
string type;
int year;
double price;
};
So far I have written that ...
![File
Edit
View
Project
Build
Debug
Test
Analyze
Tools
Extensions
Window
Help
Search (Ctrl+Q)
ConsoleApplication23
BM
Debug
x86
> Local Windows Debugger - Auto
IA Live Share
AT
ConsoleApplication23.cpp* + X
E ConsoleApplication23
(Global Scope)
O main)
43
for (int i = 0; i < sz; i++)
{
cout << "\nGame name:
44
« arr[i].name <« endl;
cout <« "Game type" <« arr[i].type <« endl;
cout <« "Game Year" << arr[i].year << endl;
cout <« "Game Price" <« arr[i].price << endl;
45
" «
46
47
48
49
50
cin.ignore();
cout <« "\nPress enter to continue..." << endl;
51
52
53
54
55
int main()
{
56
57
58
int ch = 0;
int i = 0, f = 0;
59
60
61
int num = 0;
62
Game cus_acc_arr[3];
menuInterface(();
cout <« "Please enter a choice: ";
cin >> ch;
63
64
65
66
67
68
if (ch == 1)
{ NewGame (cus_acc_arr, i);
i++;}|
69
70
71
72
else if (ch == 2)
{(cus_acc_arr, num, i); }
73
74
75
100 %
2
Ln: 71
Ch: 18
SPC
CRLF
Output
Show output from: Debug
12:41 PM
P Type here to search
11/20/2020
Diagnostic Tools
近](https://content.bartleby.com/qna-images/question/9a97ba81-ff9a-4f61-847e-dc642b7f64cf/18267e22-a999-4a79-a1c8-789039b622e5/xda7f6_thumbnail.png)
![File
Edit
View
Project
Build
Debug
Test
Analyze
Tools
Extensions
Window
Help
Search (Ctrl+Q)
ConsoleApplication23
BM
7 2 - S - Debug
> Local Windows Debugger - Auto
x86
IA Live Share
T
ConsoleApplication23.cpp* + x
A ConsoleApplication23
(Global Scope)
O main)
10
double price;
11
12
13
Evoid menuInterface ()
14
15
cout « "Hello please choose one of the following options" <« endl;
cout <« "1. Enter Game Information" << endl:
cout <« "2. Change Game Information" << endl;
cout <« "3. Show All Games" << endl:
16
17
18
19
cout << "4. Exit" << endl;
20
21
22
Evoid NewGame (struct Game arr[], int ind)
{
cout <« "\nPlease Enter Game Name: ";
cin.ignore();
getline(cin, arr[ind].name);
cout <« "Enter Game Type ";
23
24
25
26
27
getline(cin, arr[ind].type);
cout <« "Enter Year Game Was Sold";
cin >> arr[ind].year;
cout <« "Enter Price Of Game";
cin >> arr[ind].price;
28
29
30
31
32
33
34
35
Evoid EditGame (struct Game arr[], int ind)
{
36
37
38
cout
39
40
Evoid GameInfo(struct Game arr[], int sz)
{
41
42
100 %
01
A
2
Ln: 71
Ch: 18
SPC
CRLF
Output
Show output from: Debug
12:41 PM
P Type here to search
11/20/2020
Diagnostic Tools
近](https://content.bartleby.com/qna-images/question/9a97ba81-ff9a-4f61-847e-dc642b7f64cf/18267e22-a999-4a79-a1c8-789039b622e5/cluj49xb_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 6 images

- The logic will allow the user to: Load a single dimensional array of size 50 with a random number The random number will range from 1 to 1,000 (you may have duplicate values) Find the highest value and the index location that it was in Find the smallest value and the index location that it was in Display the array’s contents. Display the highest value and its index location Display the lowest value and its index location Allow the user to execute this application multiple times (some sort of loop?) You will need one for loop to load the array as well as one or two for loops to search that array. The rand also has a “nasty” tendency to create the same results repeatedly in an exe. To avoid that, we have to “shuffle the deck” every time. This ensures that all numbers are an equal probability of appearing and not the same set of values (would create a boring game). // Add this to "shuffle the deck" every time to ensure that // different values could occur else the exe produces the…arrow_forwardC# application that will allow users to enter values store all the expenses on that month in an array clothes, food, cool drinks, water, meatarrow_forwardC# program in Visual Studio Code. I need to be able to enter five integer values and then have the Unique values entered display. Private member variable to store 5 unique values (hint: use an Array or a List) Public function to get 5 unique values from the user and store them in the member variable loop to get the numbers, if a number is already stored, ignore it and keep looping until you have 5 unique numbers if a number is out of range, don't store the value, throw an exception and handle it in such a way that you don't break the loop but do message the user that the value was out of rangearrow_forward
- A data structure in which all elements have the same type is called an ______arrow_forwardC++arrow_forwardC++ Visual Studio 2019 Complete #7 Customer Accounts and #8 Search Function for Customer Accounts Program. Create the array with only 2 elements. 7.Customer Accounts Write a program that uses a structure to store the following data about a customer account: Name Address City, State, and ZIP Telephone Number Account Balance Date of Last Payment The program should use an array of at least 10 structures. It should let the user enter data into the array, change the contents of any element, and display all the data stored in the array. The program should have a menu-driven user interface. Input Validation: When the data for a new account is entered, be sure the user enters data for all the fields. No negative account balances should be entered. 8. Search Function for Customer Accounts Program Add a function to Programming Challenge 7 (Customer Accounts) that allows the user to search the structure array for a particular customer's account. It should accept part of the customer's name as an…arrow_forward
- In visual basic Create and initialize an array that will hold four popular cities in Texas.arrow_forwardThe following code segment is valid vector v; v[0] = 10; v[1] = 20; %3D I %3D O True Falsearrow_forwardWeather Statistics": Problem Statement: Write a program that uses a structure to store the following weather data for a particular month: Total Rainfall High Temperature Low Temperature Average Temperature The program should have an array of 12 structures to hold weather data for an entire year. When the program runs, it should ask the user to enter data for each month. (The average temperature should be calculated.) Once the data are entered for all the months, the program should calculate and display the average monthly rainfall, the total rainfall for the year, the highest and lowest temperatures for the year (and the months they occurred in), and the average of all the monthly average temperatures. Input Validation: Only accept temperatures within the range between -100 and +140 degrees Fahrenheit.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
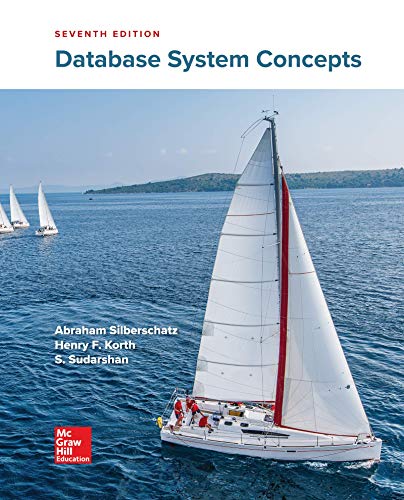
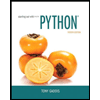
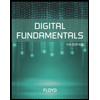
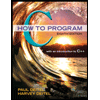
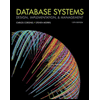
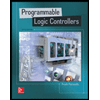