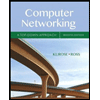
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
c
input code in "enter code here"
![Write a program that sorts a given array by using
Bubble Sort. Then, find out the index of the input number by using
Binary Search.
** Array length is fixed as 10 and each element is integer and in [0,
99]
• Function Prototype
• void bobble_sort(int" randList) - sort an array( randList ) using
bubble sort.
• int binary_search(int num, int" randlist, int low, int high) - If you
found a input number in an array, return the index of that
number. Else return -1.
• void step_printer - print each step of binary search.
You MUST show all the binary search steps on your program as shown
as below.
Example)
> Input array:88 84 48 40 39 37 20 19 13 4
Input number:25
Sorted array:4 13 19 20 37 39 40 48 84 88
Binary Search step:4 13 19 20
Binary Search step:19 20
Binary Search step:20
NOT FOUND!
Input array:4 88 13 84 19 48 20 40 39 37
Input number:19
Sorted array:4 13 19 20 37 39 40 48 84 88
Binary Search step:4 13 19 20
Binary Search step:19 20
19 is in the randList[2]!](https://content.bartleby.com/qna-images/question/300b1fc5-2fff-487f-af38-550515adf9e8/85e4294a-2117-4005-8a8b-3a157d79ce56/719shn_thumbnail.png)
Transcribed Image Text:Write a program that sorts a given array by using
Bubble Sort. Then, find out the index of the input number by using
Binary Search.
** Array length is fixed as 10 and each element is integer and in [0,
99]
• Function Prototype
• void bobble_sort(int" randList) - sort an array( randList ) using
bubble sort.
• int binary_search(int num, int" randlist, int low, int high) - If you
found a input number in an array, return the index of that
number. Else return -1.
• void step_printer - print each step of binary search.
You MUST show all the binary search steps on your program as shown
as below.
Example)
> Input array:88 84 48 40 39 37 20 19 13 4
Input number:25
Sorted array:4 13 19 20 37 39 40 48 84 88
Binary Search step:4 13 19 20
Binary Search step:19 20
Binary Search step:20
NOT FOUND!
Input array:4 88 13 84 19 48 20 40 39 37
Input number:19
Sorted array:4 13 19 20 37 39 40 48 84 88
Binary Search step:4 13 19 20
Binary Search step:19 20
19 is in the randList[2]!
![#include <stdio.h
#define SIZE 10
void bobble_sort(int* randlist)(
for (unsigned int 1 - 8; 1 < SIZE-1; +1) {
for (unsigned int j- B; 1 < SIZE-1-1; +1) {
if (alj] > alj+1]) {
int hold - aljl;
eljl - alj+1];
alj+1] - hold;
void step_printer(int *randlist, int low, int high){
II Enter code here
int binary_search(int num, int+ randList, int low, int high){
/I Enter code here
raturn -1;
int main(){
int num e;
int randlist[SIZE];
int flag:
printf("Input array:");
for( int 1- 0; 1 « SIZE; 1+ )
scanf ("sd", Arandlist(1]):
printf("Input number:");
scanf ("zd", Enum);
bobble_sort( randlist ):
printf("Sorted array:");
for( int 1 - B; 1 < SIZE; 1++ )
printf("zd ", randList[1]);
puts(");
flag - binary_search( num, randlList, 8, SIZE - 1 );
if( flag -1)
printf("NOT FOUNDI");
OSTO
printf("zd 1s in the randList[d]!", num, flag):
return B:](https://content.bartleby.com/qna-images/question/300b1fc5-2fff-487f-af38-550515adf9e8/85e4294a-2117-4005-8a8b-3a157d79ce56/wqd3en4_thumbnail.png)
Transcribed Image Text:#include <stdio.h
#define SIZE 10
void bobble_sort(int* randlist)(
for (unsigned int 1 - 8; 1 < SIZE-1; +1) {
for (unsigned int j- B; 1 < SIZE-1-1; +1) {
if (alj] > alj+1]) {
int hold - aljl;
eljl - alj+1];
alj+1] - hold;
void step_printer(int *randlist, int low, int high){
II Enter code here
int binary_search(int num, int+ randList, int low, int high){
/I Enter code here
raturn -1;
int main(){
int num e;
int randlist[SIZE];
int flag:
printf("Input array:");
for( int 1- 0; 1 « SIZE; 1+ )
scanf ("sd", Arandlist(1]):
printf("Input number:");
scanf ("zd", Enum);
bobble_sort( randlist ):
printf("Sorted array:");
for( int 1 - B; 1 < SIZE; 1++ )
printf("zd ", randList[1]);
puts(");
flag - binary_search( num, randlList, 8, SIZE - 1 );
if( flag -1)
printf("NOT FOUNDI");
OSTO
printf("zd 1s in the randList[d]!", num, flag):
return B:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- C programming Must input code in "enter code here" sectionarrow_forwardString with digit. Using C++ Set hasDigit to true if the 3-character passCode contains a digit. #include <iostream>#include <string>#include <cctype>using namespace std; int main() { bool hasDigit; string passCode; hasDigit = false; cin >> passCode; hasDigit = true; if (hasDigit) { cout << "Has a digit." << endl; } else { cout << "Has no digit." << endl; } return 0;}arrow_forwardIn a C++ program, a semicolon ( ; ) indicate _____ The end of a statement The beginning of a comment The end of the program The beginning of a block of codearrow_forward
- Choose corect option: Ludo is an good example of a) Dfa b) NDFA c) All d) fsmarrow_forwardC program to check whether a number is cute number or not using functionarrow_forwardPYTHON PROGRAMMING EXERCISE-1 Read input two strings x, y from the user and concatenate the first 5 characters of both the strings x, y and display the string formed in the output. You can assume that both the strings are greater than length 5.arrow_forward
- Create the following program that prompts a user to enter 10 integers. Create a user-defined function to print the 10 integers the user enters. C programming languagearrow_forwardC++ Which function is used to check whether a character is tab or a control code?a) isspace()b) isalnum()c) iscntrl()d) isblank()arrow_forwardC program onlyarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
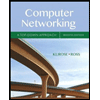
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
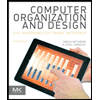
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
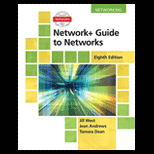
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
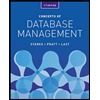
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
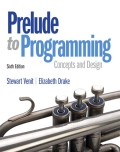
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
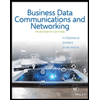
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY