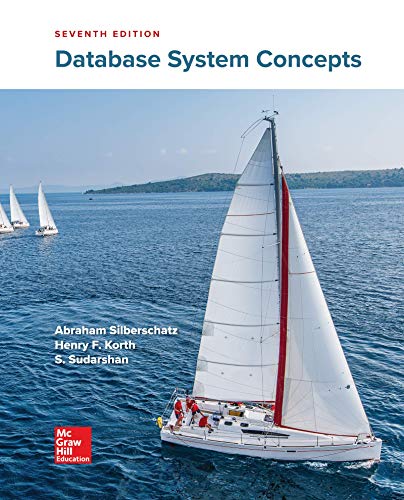
*You need to have at least one user define function
*Language: C
Write a program to find whether a given number is a Palindrome number (number that is same when you read from forward or backward). You need to take a long integer number from user as input and verify whether it’s a number and positive or not. If the input is not valid (e.g., string or float) or positive, then notify the user and ask to input valid positive number again. If the number is valid then check whether it’s a palindrome number or not. [Hint: For splitting the digits from the given number, you need to use mod % and div / operators. E.g., 123/10 = 12 & 123%10 = 3]
Input: Key in a number to check: -23
Output: The number -23 is not valid
Key in a number to check: 123454321
The number 123454321 is a PALINDROME
Do you want to continue (y/n)? y
Key in a number to check: 456734
The number 456734 is NOT a PALINDROME
Do you want to continue (y/n)? n
Good bye!!

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Driving costs - functions Learning Objectives Create a function to match the specifications Use floating-point value division Instructions Driving is expensive. Write a program with a car's miles/gallon and gas dollars/gallon (both floats) as input, and output the gas cost for 10 miles, 50 miles, and 400 miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print(f'{your_value:.2f}') Ex: If the input to your program is: 20.0 3.1599 the output is: The gas cost for 10 miles is $1.58 The gas cost for 50 miles is $7.90 The gas cost for 400 miles is $63.20 Your program must define and call the driving_cost() function. Given input parameters driven_miles, miles_per_gallon, and dollars_per_gallon, the function returns the dollar cost to drive those miles. Ex: If the function is called with: 50 20.0 3.1599 the function returns: 7.89975 def driving_cost(driven_miles, miles_per_gallon, dollars_per_gallon) Your program should…arrow_forwardAlert dont submit AI generated answer. Please code in C language.arrow_forwardCreate a string variable containing your full name (with spaces) 2) print the string as shown below 3) Create a blank 5x10 character array 4) Create a function printArray() that will print every row and column of the array 5) Create a function getNextCharacter() that gets passed the name string and returns the next non-blank character. If you have reached the end of the string then continue at the beginning. You may need to use a global variable 6) Create a function fillArray() that will fill the 2 dimensional array with the letters of your name skipping blanks. Use the above getNextCharacter() function to fill the array with only the characters in your last name. 7) Print the entire array. 8) Request a row number to print and use a sentinal loop to make sure the row number is valid. If not then request a row number again 9) Create a function printIter() that prints only the passed row using iteration 10) Create a function printRecur() that prints only the passed row using recursion…arrow_forward
- C#(Sharp): I made my code and design below. Anybody can help me some correction and add some design button and code. "Need to make C# step by step design and code "Calculator where make a calculator program that can do add, subtract, multiply, divide and square root. It should have a memory save/restore function for one number. There should be a way to set the number of fraction digits displayed". Code: using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace AktCalc { public partial class Form1 : Form { string last = ""; bool minus = false; bool plus = false; bool divide = false; bool multiply = false; string memory = ""; public Form1() { InitializeComponent(); } private void button2_Click(object sender, EventArgs e) { if (textBox1.Text.Contains(",")) { return; } else { textBox1.Text += ","; } } private void…arrow_forwardC Programarrow_forwardPlease fill in the blanks. /* This function asks for 2 strings from the user and asks the user to choose what they want to do with them. Inputs: 2 strings, and a character for choice. Output: execute that choice. Choices can be: 'E'/'e': call checkEqual() function 'C'/'c': call concatStrings() function Everything else: invalid and exit */ #include<__1__> //library to use printf and scanf #include<__2__> //library to use boolean #include<__3__> //library to use exit(0) #define len 1000 /*This function just concatenates 2 strings using a */ __4__ concatStrings(__5__ w1[], __6__ w2[], __7__ w3[]) { int w3_idx = 0; //index for w3 //Copy word1 w1 over to the combined string for(int i = 0; __8__ != __9__; i++) //loop runs as long as string is not done { __10__[__11__] = __12__[__13__]; //copy a character from w1 to w3 w3_idx++; //update…arrow_forward
- Void functions do not return any value when they are called.True or falsearrow_forwarder 6 Functions Programming Exercises te 1. Rectangle Area ngle Area The area of a rectangle is calculated according to the following formula: Area = Width X Length Design a function that accepts a rectangle's width and length as arguments and returns the rectangle's area. Use the function in a program that prompts the user to enter the rectangle's width and length, and then displays the rectangle's area.arrow_forwardDrawa structured flowchart , C++, write pseudocode that describes the process of guessing a number between 1 and 100. After each guess, the player is told that the guess is too high or too low. The process continues until the player guesses the correct number. Pick a number and have a fellow student try to guess it following your instructions.arrow_forward
- C++ Programarrow_forwardCreate the following program that prompts a user to enter 10 integers. Create a user-defined function to print the 10 integers the user enters. C programming languagearrow_forwardPART I: Functions – Math Test Write the Flowchart and Python code for the following programming problem based on the pseudocode below. Write a program that will allow a student to enter their name and then ask them to solve 10 mathematical equations. The program should display two random numbers that are to be added, such as: 247 + 129 The program should allow the student to enter the answer. The program should then display whether the answer was right or wrong, and accumulate the correct values. After the 10 questions are asked, calculate the average correct. Then display the student name, the number correct, and the average correct in both decimal and percentage format. In addition to any system functions you may use, you might consider the following functions: A function that allows the student to enter their name. A function that gets two random numbers, anywhere from 1 to 500. A function that displays the equation and asks the user to enter their answer. A function that…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
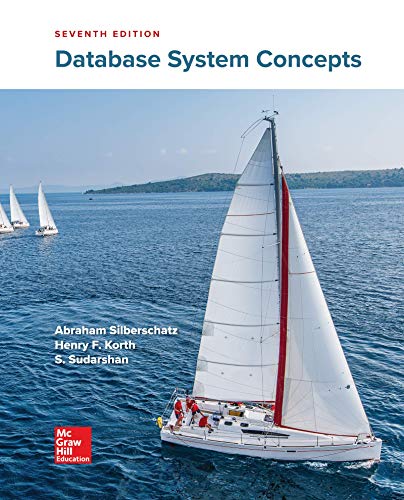
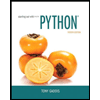
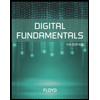
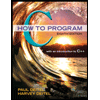
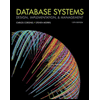
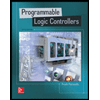