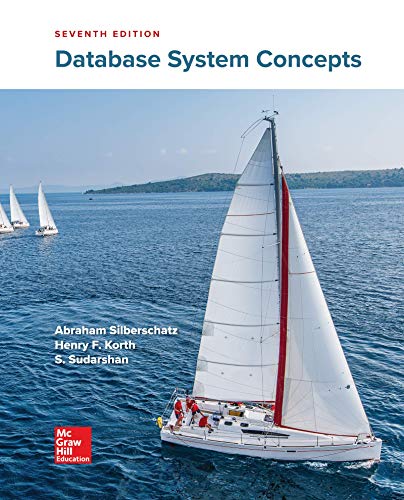
C
Write a program to perform the following:
- Create an array of 7 double random values (the price of items) between 1.0 and 100.0.
- Create an array of 7 integer random values(units of items sold) between 0 and 100.
- Create an array called “total sale” which contains the total sale for each item.
- Calculate the total sale for this store and display it
- If the number of items sold is more than 50, print that item#? needs to be reordered.
Display Example; -------------------------------------------
item # | Price (random) | Units Sold (random) | Total Sale (price*units sold) |
1 | 25.23 | 2 | 50.46 |
2 | 3.45 | 100 | 345.00 |
3 | 40.56 | 34 | 1,379.04 |
: | : | : | : |
7 | 12.35 | 48 | 592.80 |
The total sale of this store is ???.
Item #3 needs to be reordered.
------------------------------------------------------------
1. Must create functions to generate random numbers for each array
2. Must use a function to calculate the sale of each item
3. Do not use Exit statement.
4. Do not use global variables.
5. Single return from a function.

Program code:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
double doublerand(double low, double high ) /* function to generate double type random number */
{
srand((unsigned int)clock());
return ( (double)rand() * ( high - low ) ) / (double)RAND_MAX + low;
}
int intrand(int lower, int upper) /* function to generate integer type random number */
{
return (rand() % (upper - lower + 1)) + lower;
}
double sales(int unit, double price) /* function to compute sales */
{
return unit*price;
}
int main()
{
double Price[7];
int Units[7];
double totalSales[7], storeSales=0.0;
int i;
for(i=0;i<7;i++)
{
Price[i]=doublerand(1.0,100.0); /* function call to generate double type random number */
Units[i]=intrand(0,100); /* function call to generate integer type random number */
totalSales[i]=sales(Units[i],Price[i]); /* function call to calculate total sales */
storeSales +=totalSales[i]; /* calculate the store sales*/
}
printf("Display Example;--------------------------------------\n");
printf("item# Price(random) Units Sold(random) Total Sales(Price*Units Sold)\n");
for(i=0;i<7;i++)
printf("%-5d%7.2lf%15d%25.2lf\n",i+1,Price[i],Units[i],totalSales[i]); /* display details */
printf("\nThe total sale of this store is: %.2lf",storeSales);
for(i=0;i<7;i++)
{
if(Units[i]>50) /* check for units solds>50*/
printf("\nItem #%d needs to be recordered.",i+1);
}
printf("\n------------------------------------------------------");
return 0;
}
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- In visual basic Write the code that will sequentially search through the array strFirstNames and will print out the word "Found" if the name "Brian" is in the array.arrow_forwardin visual c#arrow_forwardGiven an array of integers named ages, which of the following will query this array to get all values less than 20 and store the result in teens? Question 8 options: a) int teens = from item in ages where item < 20 select item; b) var teens = from ages where item < 20 select item; c) var teens = from item in ages where item < 20 select item; d) var ages = from item in teens where item < 20 select item;arrow_forward
- The intNum array is declared as follows: Dim intNum(,) As Integer = {{6, 12, 9, 5, 2}, {35, 60, 17, 8, 10}}. The intNum(1, 4) = intNum(1, 2) - 5 statement will _____________________. a. replace the 10 amount with 12 b. replace the 5 amount with 7 c. replace the 2 amount with 4 d. None of the above.arrow_forwardThis is need in Java Declare an array named temperatures that can hold 10 doubles.arrow_forwardThe logic will allow the user to: Load a single dimensional array of size 50 with a random number The random number will range from 1 to 1,000 (you may have duplicate values) Find the highest value and the index location that it was in Find the smallest value and the index location that it was in Display the array’s contents. Display the highest value and its index location Display the lowest value and its index location Allow the user to execute this application multiple times (some sort of loop?) You will need one for loop to load the array as well as one or two for loops to search that array. The rand also has a “nasty” tendency to create the same results repeatedly in an exe. To avoid that, we have to “shuffle the deck” every time. This ensures that all numbers are an equal probability of appearing and not the same set of values (would create a boring game). // Add this to "shuffle the deck" every time to ensure that // different values could occur else the exe produces the…arrow_forward
- JAVA based programarrow_forward5. Create an array that contents 10 integer elements, and let user randomly input 10 values and put into the array, Sorts array elements in ascending order, and then print out the array element.arrow_forwardCreate an array called sales to monitor one week's worth of sales over the course of two months. Assign sales data to the array's elements. Then, in a loop, compute the average sales for the one week of each of the two months that are recorded in the array.arrow_forward
- Weight Statistics Write a program that monitors monthly weight loss each of 12 months into an array of doubles. The program should calculate and display the total weight loss for the year, the average monthly weight loss, and the months with the highest and lowest amounts. Input Validation: Do not accept negative numbers for monthly weight loss.arrow_forwardC++ you have to you use #include <random> also included a sample output has too match the layout Thank youarrow_forwardThe program should allow the user to enter the age of the child and the number of days per week the child will be at the day care center. The program should output the appropriate weekly rate. The file provided for this lab contains all of the necessary variable declarations, except the two-dimensional array. You need to write the input statements and the code that initializes the two-dimensional array, determines the weekly rate, and prints the weekly rate. Comments in the code tell you where to write your statements. 1. Open the source code file named DayCare.cpp using Notepad or the text editor of your choice. 2. Declare and initialize the two-dimensional array. 3. Write the C++ statements that retrieve the age of the child and the number of days the child will be at the day care center. 4. Determine and print the weekly rate. 5. Save this source code file in a directory of your choice, and then make that directory your working directory. 6. Compile the source code…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
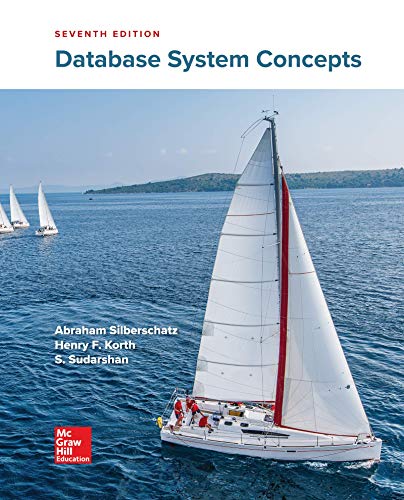
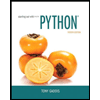
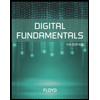
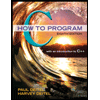
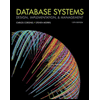
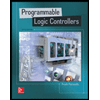