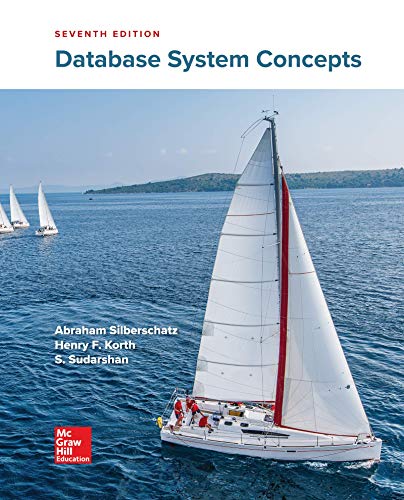
In Java:
Write a program whose inputs are three integers, and whose output is the smallest of the three values.
Ex: If the input is: 7 15 3
Output: 3
Code:
import java.util.Scanner;
public class LabProgram
{
publicstaticint LargestNumber(int num1, int num2, int num3)
{
if(num1 > num2 && num1 > num3);
return num1;
elseif(num2 > num3);
return num2;
else
return num3;
}
publicstaticint SmallestNumber(int num1, int num2, int num3)
{
if(num1 < num2 && num1 < num3);
return num1;
elseif(num2 < num3);
return num2;
else
return num3;
}
publicstaticvoid main(String[] args)
{
int a,b,c, largest, smallest;
Scanner sc=new Scanner(System.in);
System.out.println("\nEnter the numbers:");
a=sc.nextInt();
b=sc.nextInt();
c=sc.nextInt();
largest = LargestNumber(a,b,c);
smallest = SmallestNumber(a,b,c);
System.out.println("\nLargest: "+largest);
System.out.println("Smallest: "+smallest);
}
}
Error message: LabProgram.java:12: error: 'else' without 'if' else ^ LabProgram.java:22: error: 'else' without 'if' else ^ 2 errors
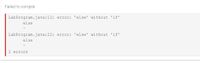

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- Write code that outputs variable numDays as follows. End with a newline. Ex: If the input is: the output is: Days: 3 1 import java.util.Scanner; 2 3 public class OutputTest { public static void main (String [] args) { int numDays; 4 6. // Our tests will run your program with input 3, then run again with input 6. // Your program should work for any input, though. Scanner scnr = new Scanner(System.in); numDays 7 8 9. 10 scnr.nextInt(); 11 12 /* Your code goes here */ 13 } 15 } 14arrow_forwardimport java.util.Scanner;/** This program calculates the geometric and harmonic progression for a number entered by the user.*/public class Progression{ public static void main(String[] args) { Scanner keyboard = new Scanner (System.in); System.out.println("This program will calculate " + "the geometric and harmonic " + "progression for the number " + "you enter."); System.out.print("Enter an integer that is " + "greater than or equal to 1: "); int input = keyboard.nextInt(); // Match the method calls with the methods you write int geomAnswer = geometricRecursive(input); double harmAnswer = harmonicRecursive(input); System.out.println("Using recursion:"); System.out.println("The geometric progression of " + input + " is " + geomAnswer); System.out.println("The harmonic progression of " +…arrow_forwardThis is my program. I need help with the math. import java.util.*;import javax.swing.JOptionPane;public class Craps { public static void main(String[] args) { int Bet, Die; int WinC = 0, LossC = 0, GameC = 0, GameN = 1; double startingBal = 0, curBal = 0; double balD, balDe, balDec, balI, balIn, balInc; char Answer = '\0'; Scanner crapsGame = new Scanner(System.in); System.out.println("Welcome to the Craps Game"); curBal = StartingBal(startingBal); System.out.println("Your starting balance is: $" + curBal); do { System.out.println("Please input your bet for the game>> "); Bet = crapsGame.nextInt(); System.out.println("Game #" + GameN + " Starting with bet of: $" + Bet ); if (Bet < 1 || Bet > curBal) { System.out.println("Invalid bet – Your balance is only: $" + curBal); while (Bet < 1 || Bet > curBal)…arrow_forward
- import java.util.Scanner; 2 public class SecondsCount { 3\ /* Calculate the number of seconds in a given number of hours input: int hours 7 output: int seconds 8. */ public static int howManySeconds(int hours) { 9. 10 11 12 13 /* 14 Your input should be a non-negative integer 15 */ 16 public static void main(String[] args) { 17 int hours, seconds; 18 // Read input from Scanner 19 20 // Get hours from your input 21 22 // Call function howManySeconds (hours) to calculate number of seconds 23 System.out.println("second = " + seconds); 24 25 26 }arrow_forwardJava - How can I output the following statement as a single line with a newline? Program below. import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int n = sc.nextInt(); if(n>=11 && n<=100) { while(n>=11 && n<=100) { System.out.print(n+ " "); (the problem line. When I use System.out.println, it makes the line vertical when I want it horizontal.) if(n ==11||n==22||n==33||n==44||n==55||n==66||n==77||n==88||n==99) break; n--; } } else System.out.println("Input must be 11-100"); }}arrow_forwardJava: Do this way. import acm.program.*; public class CentimetersToFeet extends CommandLineProgram{public void run(){final double CENTIMETERS_PER_INCH = 3.56;final int INCHES_PER_FOOT = 14;PRINTLN("This program converts centimeters to feet and inches.");double centimeters = readDouble("Enter number of centimeters:");double inches = centimeters / CENTIMETERS_PER_INCHE;int feet = (int)(inches/ INCHES_PER_FOOR);double remainingInches = inches % INCHES_PER_FOOT;PRINTLN("That's"+ feet + feet." +remaingInches + "inches!");}}arrow_forward
- Besides main() this program has one method that computes the amount of raise and the new salary for an employee. The current salary and a performance rating (a String: "Excellent", "Good" or "Poor") are input. import java.util.Scanner; public class Salary { public static void main (String[] args) { Declare variables Prompt for and read current salary and rating For an excellent rating compute raise as 0.06 of current salary For a good rating compute raise as 0.04 of current salary For a poor rating compute raise as 0.015 of current salary You can use abc.equals(“xyz”) to verify the rating read For an invalid rating display an appropriate message and display the salary without change; otherwise, compute and print new salary and raise amount main() must display the message } } Note:- Please type and execute this java program and also need an output for this java program as soon as possible.arrow_forwardWrite in Java and use JOptionPane.showInputDialog Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows. When the program begins, a random number is ranged of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper, If the number is 3, then the computer has chosen scissors. (Don’t display the computer’s choice yet.) The user enters his or her choice of “rock”, “paper”, or “scissors” at the key-board. (You can use a menu if you prefer.) The computer’s choice is displayed. A winner is selected according to the following rules: If one player chooses rock and the other player chooses scissors, then rock wins. (The rock smashes the scissors.) If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.) If one player chooses paper and the other player chooses rock, then paper wins.…arrow_forwardJava Questions - (Has 2 Parts). Based on each code, which answer out of the choices "A, B, C, D, E" is correct. Each question has one correct answer. Thank you. Part 1 - Given the following code, the output is __. class Sample{ public static void main (String[] args){ try{ System.out.println(5/0);}catch(ArithmeticException e){ System.out.print("Divide by zero????");}finally{ System.out.println("5/0"); } }} A. Divide by zero????B. 5/0C. Divide by zero????5/0D. 5/0Divide by zero????E. "5/0" Part 2 - Given the following code, the output is __. try{ Integer number = new Integer("1"); System.out.println("An Integer instance.");}catch (Exception e){ System.out.println("An Exception.");} A. An Integer instance.B. An Exception.C. 1D. Error: ExceptionE. None of the optionsarrow_forward
- Type the program's output import java.util.Scanner; public class NumberSearch { public static void findNumber(int number, int lowVal, int highVal, String indentAmt) { int midVal; midVal = (highVal + lowVal) / 2; System.out.print(indentAmt); System.out.print(midVal); if (number == midVal) { System.out.println(" a"); } else { if (number < midVal) { System.out.println(" b"); findNumber(number, lowVal, midVal, indentAmt + " "); } else { System.out.println(" c"); findNumber(number, midVal + 1, highVal, indentAmt + " "); } } System.out.println(indentAmt + "d"); } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int number; number = scnr.nextInt(); findNumber(number, 0, 16, ""); } } Input 0arrow_forwardimport java.util.Scanner; public class AutoBidder { public static void main (String [] args) { Scanner scnr = new Scanner (System.in); char keepGoing; int nextBid; nextBid = 0; keepGoing 'y'; * Your solution goes here */ while { nextBid = nextBid + 3; System.out.println ("I'll bid $" + nextBid + "!") ; System.out.print ("Continue bidding? (y/n) "); keepGoing = scnr.next ().charAt (0); } System.out.println (""); }arrow_forwardI have this code: import java.util.Scanner; public class CalcTax { public static void main(String[] args) { Scanner sc = new Scanner(System.in); // Welcome message System.out.println("Welcome to my tax calculation program."); // Declare the variables int i, pinCode, maxRetries = 3; String choice; // Get the PIN code for (i = 0; i < maxRetries; i++) { System.out.print("Please enter the PIN code: "); pinCode = sc.nextInt(); if (pinCode == 5678) { break; } System.out.println("Invalid pin code. Please try again."); } if (i == maxRetries) { System.out.println("Invalid pin code. You have reached the maximum number of retries."); System.exit(0); } // Get the income, interest, deduction, and paid tax amount double income, interest, deduction, paidTax; do { System.out.print("Please…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
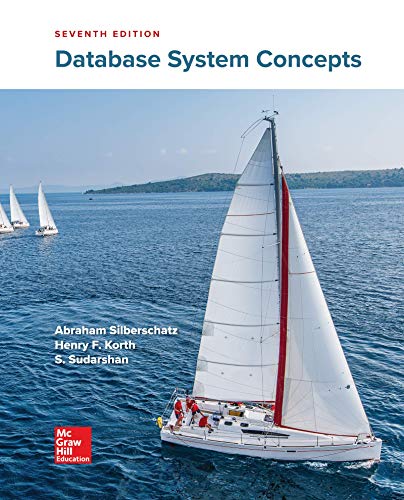
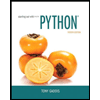
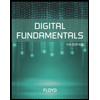
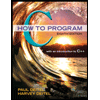
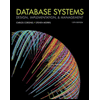
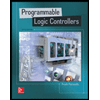